Training machine learning models on tabular data: an end-to-end example
Contents
Training machine learning models on tabular data: an end-to-end example#
This tutorial covers the following steps:
Import data from your local machine into the Databricks File System (DBFS)
Visualize the data using Seaborn and matplotlib
Run a parallel hyperparameter sweep to train machine learning models on the dataset
Explore the results of the hyperparameter sweep with MLflow
Register the best performing model in MLflow
Apply the registered model to another dataset using a Spark UDF
Set up model serving for low-latency requests
In this example, you build a model to predict the quality of Portugese “Vinho Verde” wine based on the wine’s physicochemical properties.
The example uses a dataset from the UCI Machine Learning Repository, presented in Modeling wine preferences by data mining from physicochemical properties [Cortez et al., 2009].
Requirements#
This notebook requires Databricks Runtime for Machine Learning.
If you are using Databricks Runtime 7.3 LTS ML or below, you must update the CloudPickle library. To do that, uncomment and run the %pip install
command in Cmd 2.
# This command is only required if you are using a cluster running DBR 7.3 LTS ML or below.
#%pip install --upgrade cloudpickle
Import data#
In this section, you download a dataset from the web and upload it to Databricks File System (DBFS).
Navigate to https://archive.ics.uci.edu/ml/machine-learning-databases/wine-quality/ and download both
winequality-red.csv
andwinequality-white.csv
to your local machine.From this Databricks notebook, select File > Upload Data, and drag these files to the drag-and-drop target to upload them to the Databricks File System (DBFS).
Note: if you don’t have the File > Upload Data option, you can load the dataset from the Databricks example datasets. Uncomment and run the last two lines in the following cell.
Click Next. Some auto-generated code to load the data appears. Select pandas, and copy the example code.
Create a new cell, then paste in the sample code. It will look similar to the code shown in the following cell. Make these changes:
Pass
sep=';'
topd.read_csv
Change the variable names from
df1
anddf2
towhite_wine
andred_wine
, as shown in the following cell.
# If you have the File > Upload Data menu option, follow the instructions in the previous cell to upload the data from your local machine.
# The generated code, including the required edits described in the previous cell, is shown here for reference.
import pandas as pd
# In the following lines, replace <username> with your username.
white_wine = pd.read_csv("/dbfs/FileStore/shared_uploads/<username>/winequality_white.csv", sep=';')
red_wine = pd.read_csv("/dbfs/FileStore/shared_uploads/<username>/winequality_red.csv", sep=';')
# If you do not have the File > Upload Data menu option, uncomment and run these lines to load the dataset.
#white_wine = pd.read_csv("/dbfs/databricks-datasets/wine-quality/winequality-white.csv", sep=";")
#red_wine = pd.read_csv("/dbfs/databricks-datasets/wine-quality/winequality-red.csv", sep=";")
Merge the two DataFrames into a single dataset, with a new binary feature “is_red” that indicates whether the wine is red or white.
red_wine['is_red'] = 1
white_wine['is_red'] = 0
data = pd.concat([red_wine, white_wine], axis=0)
# Remove spaces from column names
data.rename(columns=lambda x: x.replace(' ', '_'), inplace=True)
data.head()
fixed_acidity | volatile_acidity | citric_acid | residual_sugar | chlorides | free_sulfur_dioxide | total_sulfur_dioxide | density | pH | sulphates | alcohol | quality | is_red | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 7.4 | 0.70 | 0.00 | 1.9 | 0.076 | 11.0 | 34.0 | 0.9978 | 3.51 | 0.56 | 9.4 | 5 | 1 |
1 | 7.8 | 0.88 | 0.00 | 2.6 | 0.098 | 25.0 | 67.0 | 0.9968 | 3.20 | 0.68 | 9.8 | 5 | 1 |
2 | 7.8 | 0.76 | 0.04 | 2.3 | 0.092 | 15.0 | 54.0 | 0.9970 | 3.26 | 0.65 | 9.8 | 5 | 1 |
3 | 11.2 | 0.28 | 0.56 | 1.9 | 0.075 | 17.0 | 60.0 | 0.9980 | 3.16 | 0.58 | 9.8 | 6 | 1 |
4 | 7.4 | 0.70 | 0.00 | 1.9 | 0.076 | 11.0 | 34.0 | 0.9978 | 3.51 | 0.56 | 9.4 | 5 | 1 |
Visualize data#
Before training a model, explore the dataset using Seaborn and Matplotlib.
Plot a histogram of the dependent variable, quality.
import seaborn as sns
sns.distplot(data.quality, kde=False)
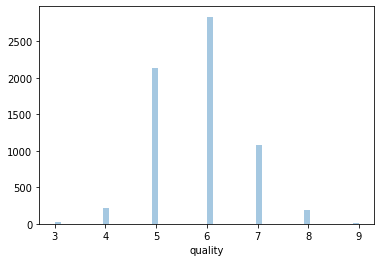
Looks like quality scores are normally distributed between 3 and 9.
Define a wine as high quality if it has quality >= 7.
high_quality = (data.quality >= 7).astype(int)
data.quality = high_quality
Box plots are useful in noticing correlations between features and a binary label.
import matplotlib.pyplot as plt
dims = (3, 4)
f, axes = plt.subplots(dims[0], dims[1], figsize=(25, 15))
axis_i, axis_j = 0, 0
for col in data.columns:
if col == 'is_red' or col == 'quality':
continue # Box plots cannot be used on indicator variables
sns.boxplot(x=high_quality, y=data[col], ax=axes[axis_i, axis_j])
axis_j += 1
if axis_j == dims[1]:
axis_i += 1
axis_j = 0
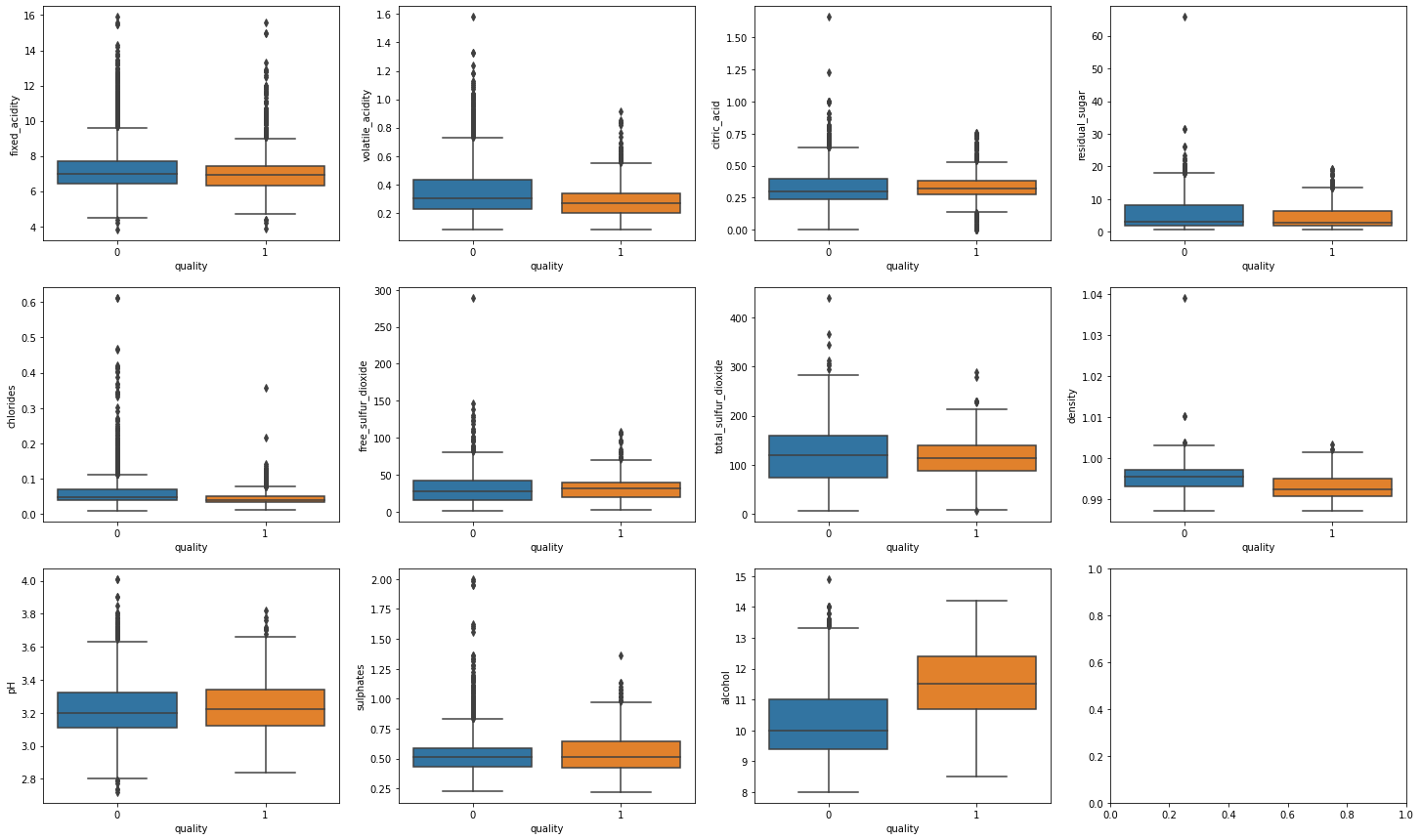
In the above box plots, a few variables stand out as good univariate predictors of quality.
In the alcohol box plot, the median alcohol content of high quality wines is greater than even the 75th quantile of low quality wines. High alcohol content is correlated with quality.
In the density box plot, low quality wines have a greater density than high quality wines. Density is inversely correlated with quality.
Preprocess data#
Prior to training a model, check for missing values and split the data into training and validation sets.
data.isna().any()
There are no missing values.
Prepare dataset for training baseline model#
Split the input data into 3 sets:
Train (60% of the dataset used to train the model)
Validation (20% of the dataset used to tune the hyperparameters)
Test (20% of the dataset used to report the true performance of the model on an unseen dataset)
from sklearn.model_selection import train_test_split
X = data.drop(["quality"], axis=1)
y = data.quality
# Split out the training data
X_train, X_rem, y_train, y_rem = train_test_split(X, y, train_size=0.6, random_state=123)
# Split the remaining data equally into validation and test
X_val, X_test, y_val, y_test = train_test_split(X_rem, y_rem, test_size=0.5, random_state=123)
Build a baseline model#
This task seems well suited to a random forest classifier, since the output is binary and there may be interactions between multiple variables.
The following code builds a simple classifier using scikit-learn. It uses MLflow to keep track of the model accuracy, and to save the model for later use.
import mlflow
import mlflow.pyfunc
import mlflow.sklearn
import numpy as np
import sklearn
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import roc_auc_score
from mlflow.models.signature import infer_signature
from mlflow.utils.environment import _mlflow_conda_env
import cloudpickle
import time
# The predict method of sklearn's RandomForestClassifier returns a binary classification (0 or 1).
# The following code creates a wrapper function, SklearnModelWrapper, that uses
# the predict_proba method to return the probability that the observation belongs to each class.
class SklearnModelWrapper(mlflow.pyfunc.PythonModel):
def __init__(self, model):
self.model = model
def predict(self, context, model_input):
return self.model.predict_proba(model_input)[:,1]
# mlflow.start_run creates a new MLflow run to track the performance of this model.
# Within the context, you call mlflow.log_param to keep track of the parameters used, and
# mlflow.log_metric to record metrics like accuracy.
with mlflow.start_run(run_name='untuned_random_forest'):
n_estimators = 10
model = RandomForestClassifier(n_estimators=n_estimators, random_state=np.random.RandomState(123))
model.fit(X_train, y_train)
# predict_proba returns [prob_negative, prob_positive], so slice the output with [:, 1]
predictions_test = model.predict_proba(X_test)[:,1]
auc_score = roc_auc_score(y_test, predictions_test)
mlflow.log_param('n_estimators', n_estimators)
# Use the area under the ROC curve as a metric.
mlflow.log_metric('auc', auc_score)
wrappedModel = SklearnModelWrapper(model)
# Log the model with a signature that defines the schema of the model's inputs and outputs.
# When the model is deployed, this signature will be used to validate inputs.
signature = infer_signature(X_train, wrappedModel.predict(None, X_train))
# MLflow contains utilities to create a conda environment used to serve models.
# The necessary dependencies are added to a conda.yaml file which is logged along with the model.
conda_env = _mlflow_conda_env(
additional_conda_deps=None,
additional_pip_deps=["cloudpickle=={}".format(cloudpickle.__version__), "scikit-learn=={}".format(sklearn.__version__)],
additional_conda_channels=None,
)
mlflow.pyfunc.log_model("random_forest_model", python_model=wrappedModel, conda_env=conda_env, signature=signature)
Examine the learned feature importances output by the model as a sanity-check.
feature_importances = pd.DataFrame(model.feature_importances_, index=X_train.columns.tolist(), columns=['importance'])
feature_importances.sort_values('importance', ascending=False)
importance | |
---|---|
alcohol | 0.160192 |
density | 0.117415 |
volatile_acidity | 0.093136 |
chlorides | 0.086618 |
residual_sugar | 0.082544 |
free_sulfur_dioxide | 0.080473 |
pH | 0.080212 |
total_sulfur_dioxide | 0.077798 |
sulphates | 0.075780 |
citric_acid | 0.071857 |
fixed_acidity | 0.071841 |
is_red | 0.002134 |
As illustrated by the boxplots shown previously, both alcohol and density are important in predicting quality.
You logged the Area Under the ROC Curve (AUC) to MLflow. Click Experiment at the upper right to display the Experiment Runs sidebar.
The model achieved an AUC of 0.854.
A random classifier would have an AUC of 0.5, and higher AUC values are better. For more information, see Receiver Operating Characteristic Curve.
Register the model in MLflow Model Registry#
By registering this model in Model Registry, you can easily reference the model from anywhere within Databricks.
The following section shows how to do this programmatically, but you can also register a model using the UI. See “Create or register a model using the UI” (AWS|Azure|GCP).
run_id = mlflow.search_runs(filter_string='tags.mlflow.runName = "untuned_random_forest"').iloc[0].run_id
# If you see the error "PERMISSION_DENIED: User does not have any permission level assigned to the registered model",
# the cause may be that a model already exists with the name "wine_quality". Try using a different name.
model_name = "wine_quality"
model_version = mlflow.register_model(f"runs:/{run_id}/random_forest_model", model_name)
# Registering the model takes a few seconds, so add a small delay
time.sleep(15)
You should now see the model in the Models page. To display the Models page, click the Models icon in the left sidebar.
Next, transition this model to production and load it into this notebook from Model Registry.
from mlflow.tracking import MlflowClient
client = MlflowClient()
client.transition_model_version_stage(
name=model_name,
version=model_version.version,
stage="Production",
)
The Models page now shows the model version in stage “Production”.
You can now refer to the model using the path “models:/wine_quality/production”.
model = mlflow.pyfunc.load_model(f"models:/{model_name}/production")
# Sanity-check: This should match the AUC logged by MLflow
print(f'AUC: {roc_auc_score(y_test, model.predict(X_test))}')
##Experiment with a new model
The random forest model performed well even without hyperparameter tuning.
The following code uses the xgboost library to train a more accurate model. It runs a parallel hyperparameter sweep to train multiple models in parallel, using Hyperopt and SparkTrials. As before, the code tracks the performance of each parameter configuration with MLflow.
from hyperopt import fmin, tpe, hp, SparkTrials, Trials, STATUS_OK
from hyperopt.pyll import scope
from math import exp
import mlflow.xgboost
import numpy as np
import xgboost as xgb
search_space = {
'max_depth': scope.int(hp.quniform('max_depth', 4, 100, 1)),
'learning_rate': hp.loguniform('learning_rate', -3, 0),
'reg_alpha': hp.loguniform('reg_alpha', -5, -1),
'reg_lambda': hp.loguniform('reg_lambda', -6, -1),
'min_child_weight': hp.loguniform('min_child_weight', -1, 3),
'objective': 'binary:logistic',
'seed': 123, # Set a seed for deterministic training
}
def train_model(params):
# With MLflow autologging, hyperparameters and the trained model are automatically logged to MLflow.
mlflow.xgboost.autolog()
with mlflow.start_run(nested=True):
train = xgb.DMatrix(data=X_train, label=y_train)
validation = xgb.DMatrix(data=X_val, label=y_val)
# Pass in the validation set so xgb can track an evaluation metric. XGBoost terminates training when the evaluation metric
# is no longer improving.
booster = xgb.train(params=params, dtrain=train, num_boost_round=1000,\
evals=[(validation, "validation")], early_stopping_rounds=50)
validation_predictions = booster.predict(validation)
auc_score = roc_auc_score(y_val, validation_predictions)
mlflow.log_metric('auc', auc_score)
signature = infer_signature(X_train, booster.predict(train))
mlflow.xgboost.log_model(booster, "model", signature=signature)
# Set the loss to -1*auc_score so fmin maximizes the auc_score
return {'status': STATUS_OK, 'loss': -1*auc_score, 'booster': booster.attributes()}
# Greater parallelism will lead to speedups, but a less optimal hyperparameter sweep.
# A reasonable value for parallelism is the square root of max_evals.
spark_trials = SparkTrials(parallelism=10)
# Run fmin within an MLflow run context so that each hyperparameter configuration is logged as a child run of a parent
# run called "xgboost_models" .
with mlflow.start_run(run_name='xgboost_models'):
best_params = fmin(
fn=train_model,
space=search_space,
algo=tpe.suggest,
max_evals=96,
trials=spark_trials,
)
Use MLflow to view the results#
Open up the Experiment Runs sidebar to see the MLflow runs. Click on Date next to the down arrow to display a menu, and select ‘auc’ to display the runs sorted by the auc metric. The highest auc value is 0.90.
MLflow tracks the parameters and performance metrics of each run. Click the External Link icon at the top of the Experiment Runs sidebar to navigate to the MLflow Runs Table.
Now investigate how the hyperparameter choice correlates with AUC. Click the “+” icon to expand the parent run, then select all runs except the parent, and click “Compare”. Select the Parallel Coordinates Plot.
The Parallel Coordinates Plot is useful in understanding the impact of parameters on a metric. You can drag the pink slider bar at the upper right corner of the plot to highlight a subset of AUC values and the corresponding parameter values. The plot below highlights the highest AUC values:

Notice that all of the top performing runs have a low value for reg_lambda and learning_rate.
You could run another hyperparameter sweep to explore even lower values for these parameters. For simplicity, that step is not included in this example.
You used MLflow to log the model produced by each hyperparameter configuration. The following code finds the best performing run and saves the model to Model Registry.
best_run = mlflow.search_runs(order_by=['metrics.auc DESC']).iloc[0]
print(f'AUC of Best Run: {best_run["metrics.auc"]}')
Update the production wine_quality
model in MLflow Model Registry#
Earlier, you saved the baseline model to Model Registry with the name wine_quality
. Now that you have a created a more accurate model, update wine_quality
.
new_model_version = mlflow.register_model(f"runs:/{best_run.run_id}/model", model_name)
# Registering the model takes a few seconds, so add a small delay
time.sleep(15)
Click Models in the left sidebar to see that the wine_quality
model now has two versions.
The following code promotes the new version to production.
# Archive the old model version
client.transition_model_version_stage(
name=model_name,
version=model_version.version,
stage="Archived"
)
# Promote the new model version to Production
client.transition_model_version_stage(
name=model_name,
version=new_model_version.version,
stage="Production"
)
Clients that call load_model now receive the new model.
# This code is the same as the last block of "Building a Baseline Model". No change is required for clients to get the new model!
model = mlflow.pyfunc.load_model(f"models:/{model_name}/production")
print(f'AUC: {roc_auc_score(y_test, model.predict(X_test))}')
The auc value on the test set for the new model is 0.90. You beat the baseline!
##Batch inference
There are many scenarios where you might want to evaluate a model on a corpus of new data. For example, you may have a fresh batch of data, or may need to compare the performance of two models on the same corpus of data.
The following code evaluates the model on data stored in a Delta table, using Spark to run the computation in parallel.
# To simulate a new corpus of data, save the existing X_train data to a Delta table.
# In the real world, this would be a new batch of data.
spark_df = spark.createDataFrame(X_train)
# Replace <username> with your username before running this cell.
table_path = "dbfs:/<username>/delta/wine_data"
# Delete the contents of this path in case this cell has already been run
dbutils.fs.rm(table_path, True)
spark_df.write.format("delta").save(table_path)
Load the model into a Spark UDF, so it can be applied to the Delta table.
import mlflow.pyfunc
apply_model_udf = mlflow.pyfunc.spark_udf(spark, f"models:/{model_name}/production")
# Read the "new data" from Delta
new_data = spark.read.format("delta").load(table_path)
display(new_data)
fixed_acidity | volatile_acidity | citric_acid | residual_sugar | chlorides | free_sulfur_dioxide | total_sulfur_dioxide | density | pH | sulphates | alcohol | is_red |
---|---|---|---|---|---|---|---|---|---|---|---|
7.2 | 0.23 | 0.39 | 2.3 | 0.033 | 29.0 | 102.0 | 0.9908 | 3.26 | 0.54 | 12.3 | 0 |
6.4 | 0.24 | 0.27 | 1.5 | 0.04 | 35.0 | 105.0 | 0.98914 | 3.13 | 0.3 | 12.4 | 0 |
7.4 | 0.24 | 0.29 | 10.1 | 0.05 | 21.0 | 105.0 | 0.9962 | 3.13 | 0.35 | 9.5 | 0 |
8.6 | 0.37 | 0.65 | 6.4 | 0.08 | 3.0 | 8.0 | 0.99817 | 3.27 | 0.58 | 11.0 | 1 |
5.9 | 0.32 | 0.39 | 3.3 | 0.114 | 24.0 | 140.0 | 0.9934 | 3.09 | 0.45 | 9.2 | 0 |
7.0 | 0.42 | 0.35 | 1.6 | 0.088 | 16.0 | 39.0 | 0.9961 | 3.34 | 0.55 | 9.2 | 1 |
6.7 | 0.31 | 0.44 | 6.7 | 0.054 | 29.0 | 160.0 | 0.9952 | 3.04 | 0.44 | 9.6 | 0 |
8.1 | 0.545 | 0.18 | 1.9 | 0.08 | 13.0 | 35.0 | 0.9972 | 3.3 | 0.59 | 9.0 | 1 |
6.3 | 0.41 | 0.3 | 3.2 | 0.03 | 49.0 | 164.0 | 0.9927 | 3.53 | 0.79 | 11.7 | 0 |
7.3 | 0.49 | 0.32 | 5.2 | 0.043 | 18.0 | 104.0 | 0.9952 | 3.24 | 0.45 | 10.7 | 0 |
5.7 | 0.24 | 0.47 | 6.3 | 0.069 | 35.0 | 182.0 | 0.99391 | 3.11 | 0.46 | 9.73333333333333 | 0 |
7.2 | 0.23 | 0.32 | 8.5 | 0.058 | 47.0 | 186.0 | 0.9956 | 3.19 | 0.4 | 9.9 | 0 |
6.9 | 0.31 | 0.34 | 7.4 | 0.059 | 36.0 | 174.0 | 0.9963 | 3.46 | 0.62 | 11.1 | 0 |
6.0 | 0.26 | 0.32 | 3.5 | 0.028 | 29.0 | 113.0 | 0.9912 | 3.4 | 0.71 | 12.3 | 0 |
6.5 | 0.32 | 0.12 | 11.5 | 0.033 | 35.0 | 165.0 | 0.9974 | 3.22 | 0.32 | 9.0 | 0 |
7.1 | 0.21 | 0.3 | 1.4 | 0.037 | 45.0 | 143.0 | 0.9932 | 3.13 | 0.33 | 9.9 | 0 |
8.2 | 0.27 | 0.39 | 7.8 | 0.039 | 49.0 | 208.0 | 0.9976 | 3.31 | 0.51 | 9.5 | 0 |
6.8 | 0.26 | 0.24 | 7.8 | 0.052 | 54.0 | 214.0 | 0.9961 | 3.13 | 0.47 | 8.9 | 0 |
7.0 | 0.36 | 0.35 | 2.5 | 0.048 | 67.0 | 161.0 | 0.99146 | 3.05 | 0.56 | 11.1 | 0 |
7.2 | 0.25 | 0.32 | 1.5 | 0.054 | 24.0 | 105.0 | 0.99154 | 3.17 | 0.48 | 11.1 | 0 |
8.4 | 0.19 | 0.43 | 2.1 | 0.052 | 20.0 | 104.0 | 0.994 | 2.85 | 0.46 | 9.5 | 0 |
5.6 | 0.615 | 0.0 | 1.6 | 0.089 | 16.0 | 59.0 | 0.9943 | 3.58 | 0.52 | 9.9 | 1 |
6.6 | 0.085 | 0.33 | 1.4 | 0.036 | 17.0 | 109.0 | 0.99306 | 3.27 | 0.61 | 9.5 | 0 |
8.8 | 0.39 | 0.35 | 1.8 | 0.096 | 22.0 | 80.0 | 0.99016 | 2.95 | 0.54 | 12.6 | 0 |
6.0 | 0.27 | 0.28 | 4.8 | 0.063 | 31.0 | 201.0 | 0.9964 | 3.69 | 0.71 | 10.0 | 0 |
7.3 | 0.32 | 0.23 | 2.3 | 0.066 | 35.0 | 70.0 | 0.99588 | 3.43 | 0.62 | 10.1 | 1 |
9.2 | 0.71 | 0.23 | 6.2 | 0.042 | 15.0 | 93.0 | 0.9948 | 2.89 | 0.34 | 10.1 | 0 |
7.5 | 0.22 | 0.32 | 2.4 | 0.045 | 29.0 | 100.0 | 0.99135 | 3.08 | 0.6 | 11.3 | 0 |
7.5 | 0.14 | 0.74 | 1.6 | 0.035 | 21.0 | 126.0 | 0.9933 | 3.26 | 0.45 | 10.2 | 0 |
5.6 | 0.245 | 0.32 | 1.1 | 0.047 | 24.0 | 152.0 | 0.9927 | 3.12 | 0.42 | 9.3 | 0 |
5.8 | 0.28 | 0.66 | 9.1 | 0.039 | 26.0 | 159.0 | 0.9965 | 3.66 | 0.55 | 10.8 | 0 |
6.3 | 0.15 | 0.3 | 1.4 | 0.022 | 38.0 | 100.0 | 0.99099 | 3.42 | 0.57 | 11.4 | 0 |
7.1 | 0.37 | 0.3 | 6.2 | 0.04 | 49.0 | 139.0 | 0.99021 | 3.17 | 0.27 | 13.6 | 0 |
6.5 | 0.29 | 0.53 | 1.7 | 0.04 | 41.0 | 192.0 | 0.9922 | 3.26 | 0.59 | 10.4 | 0 |
7.4 | 0.28 | 0.5 | 12.1 | 0.049 | 48.0 | 122.0 | 0.9973 | 3.01 | 0.44 | 9.0 | 0 |
7.1 | 0.32 | 0.24 | 13.1 | 0.05 | 52.0 | 204.0 | 0.998 | 3.1 | 0.49 | 8.8 | 0 |
6.0 | 0.27 | 0.32 | 3.6 | 0.035 | 36.0 | 133.0 | 0.99215 | 3.23 | 0.46 | 10.8 | 0 |
9.2 | 0.18 | 0.49 | 1.5 | 0.041 | 39.0 | 130.0 | 0.9945 | 3.04 | 0.49 | 9.8 | 0 |
6.6 | 0.84 | 0.03 | 2.3 | 0.059 | 32.0 | 48.0 | 0.9952 | 3.52 | 0.56 | 12.3 | 1 |
7.6 | 0.14 | 0.74 | 1.6 | 0.04 | 27.0 | 103.0 | 0.9916 | 3.07 | 0.4 | 10.8 | 0 |
5.5 | 0.29 | 0.3 | 1.1 | 0.022 | 20.0 | 110.0 | 0.98869 | 3.34 | 0.38 | 12.8 | 0 |
7.2 | 0.22 | 0.28 | 7.2 | 0.06 | 41.0 | 132.0 | 0.9935 | 3.08 | 0.59 | 11.3 | 0 |
7.6 | 0.27 | 0.29 | 2.5 | 0.059 | 37.0 | 115.0 | 0.99328 | 3.09 | 0.37 | 9.8 | 0 |
7.3 | 0.2 | 0.39 | 2.3 | 0.048 | 24.0 | 87.0 | 0.99044 | 2.94 | 0.35 | 12.0 | 0 |
7.2 | 0.66 | 0.03 | 2.3 | 0.078 | 16.0 | 86.0 | 0.99743 | 3.53 | 0.57 | 9.7 | 1 |
7.6 | 0.33 | 0.36 | 2.1 | 0.034 | 26.0 | 172.0 | 0.9944 | 3.42 | 0.48 | 10.5 | 0 |
9.0 | 0.43 | 0.3 | 1.5 | 0.05 | 7.0 | 175.0 | 0.9951 | 3.11 | 0.45 | 9.7 | 0 |
8.0 | 0.17 | 0.29 | 2.4 | 0.029 | 52.0 | 119.0 | 0.98944 | 3.03 | 0.33 | 12.9 | 0 |
7.4 | 0.27 | 0.31 | 2.4 | 0.014 | 15.0 | 143.0 | 0.99094 | 3.03 | 0.65 | 12.0 | 0 |
8.0 | 0.27 | 0.33 | 1.2 | 0.05 | 41.0 | 103.0 | 0.99002 | 3.0 | 0.45 | 12.4 | 0 |
7.5 | 0.21 | 0.29 | 1.5 | 0.046 | 35.0 | 107.0 | 0.99123 | 3.15 | 0.45 | 11.3 | 0 |
7.3 | 0.39 | 0.31 | 2.4 | 0.074 | 9.0 | 46.0 | 0.9962 | 3.41 | 0.54 | 9.4 | 1 |
6.9 | 0.19 | 0.35 | 13.5 | 0.038 | 49.0 | 118.0 | 0.99546 | 3.0 | 0.63 | 10.7 | 0 |
7.3 | 0.25 | 0.28 | 1.5 | 0.043 | 19.0 | 113.0 | 0.99338 | 3.38 | 0.56 | 10.1 | 0 |
8.0 | 0.19 | 0.3 | 2.0 | 0.053 | 48.0 | 140.0 | 0.994 | 3.18 | 0.49 | 9.6 | 0 |
6.4 | 0.24 | 0.32 | 0.95 | 0.041 | 23.0 | 131.0 | 0.99033 | 3.25 | 0.35 | 11.8 | 0 |
6.6 | 0.2 | 0.14 | 4.4 | 0.184 | 35.0 | 168.0 | 0.99396 | 2.93 | 0.45 | 9.4 | 0 |
6.1 | 0.15 | 0.29 | 6.2 | 0.046 | 39.0 | 151.0 | 0.99471 | 3.6 | 0.44 | 10.6 | 0 |
8.8 | 0.6 | 0.29 | 2.2 | 0.098 | 5.0 | 15.0 | 0.9988 | 3.36 | 0.49 | 9.1 | 1 |
7.1 | 0.38 | 0.29 | 13.6 | 0.041 | 30.0 | 137.0 | 0.99461 | 3.02 | 0.96 | 12.1 | 0 |
7.1 | 0.21 | 0.72 | 1.6 | 0.167 | 65.0 | 120.0 | 0.99324 | 2.97 | 0.51 | 9.2 | 0 |
7.5 | 0.29 | 0.36 | 15.7 | 0.05 | 29.0 | 124.0 | 0.9968 | 3.06 | 0.54 | 10.4 | 0 |
5.4 | 0.15 | 0.32 | 2.5 | 0.037 | 10.0 | 51.0 | 0.98878 | 3.04 | 0.58 | 12.6 | 0 |
7.2 | 0.39 | 0.63 | 11.0 | 0.044 | 55.0 | 156.0 | 0.9974 | 3.09 | 0.44 | 8.7 | 0 |
7.6 | 0.26 | 0.32 | 1.3 | 0.048 | 23.0 | 76.0 | 0.9903 | 2.96 | 0.46 | 12.0 | 0 |
11.8 | 0.23 | 0.38 | 11.1 | 0.034 | 15.0 | 123.0 | 0.9997 | 2.93 | 0.55 | 9.7 | 0 |
6.0 | 0.19 | 0.37 | 9.7 | 0.032 | 17.0 | 50.0 | 0.9932 | 3.08 | 0.66 | 12.0 | 0 |
8.1 | 0.28 | 0.34 | 1.3 | 0.035 | 11.0 | 126.0 | 0.99232 | 3.14 | 0.5 | 9.8 | 0 |
6.3 | 0.23 | 0.21 | 5.1 | 0.035 | 29.0 | 142.0 | 0.9942 | 3.36 | 0.33 | 10.1 | 0 |
6.4 | 0.28 | 0.36 | 1.3 | 0.053 | 28.0 | 186.0 | 0.99211 | 3.31 | 0.45 | 10.8 | 0 |
10.0 | 0.73 | 0.43 | 2.3 | 0.059 | 15.0 | 31.0 | 0.9966 | 3.15 | 0.57 | 11.0 | 1 |
6.5 | 0.5 | 0.22 | 4.1 | 0.036 | 35.0 | 131.0 | 0.9902 | 3.26 | 0.55 | 13.0 | 0 |
7.0 | 0.36 | 0.3 | 5.0 | 0.04 | 40.0 | 143.0 | 0.99173 | 3.33 | 0.42 | 12.2 | 0 |
5.7 | 0.23 | 0.28 | 9.65 | 0.025 | 26.0 | 121.0 | 0.9925 | 3.28 | 0.38 | 11.3 | 0 |
6.8 | 0.3 | 0.26 | 20.3 | 0.037 | 45.0 | 150.0 | 0.99727 | 3.04 | 0.38 | 12.3 | 0 |
7.5 | 0.22 | 0.33 | 6.7 | 0.036 | 45.0 | 138.0 | 0.9939 | 3.2 | 0.68 | 11.4 | 0 |
5.1 | 0.35 | 0.26 | 6.8 | 0.034 | 36.0 | 120.0 | 0.99188 | 3.38 | 0.4 | 11.5 | 0 |
6.15 | 0.21 | 0.37 | 3.2 | 0.021 | 20.0 | 80.0 | 0.99076 | 3.39 | 0.47 | 12.0 | 0 |
6.0 | 0.21 | 0.3 | 8.7 | 0.036 | 47.0 | 127.0 | 0.99368 | 3.18 | 0.39 | 10.6 | 0 |
6.2 | 0.23 | 0.36 | 17.2 | 0.039 | 37.0 | 130.0 | 0.99946 | 3.23 | 0.43 | 8.8 | 0 |
7.4 | 0.25 | 0.36 | 13.2 | 0.067 | 53.0 | 178.0 | 0.9976 | 3.01 | 0.48 | 9.0 | 0 |
6.5 | 0.22 | 0.28 | 3.7 | 0.059 | 29.0 | 151.0 | 0.99177 | 3.23 | 0.41 | 12.1 | 0 |
7.5 | 0.38 | 0.29 | 4.9 | 0.021 | 38.0 | 113.0 | 0.99026 | 3.08 | 0.48 | 13.0 | 0 |
6.6 | 0.27 | 0.33 | 1.4 | 0.042 | 24.0 | 183.0 | 0.99215 | 3.29 | 0.46 | 10.7 | 0 |
7.9 | 0.17 | 0.32 | 1.6 | 0.053 | 47.0 | 150.0 | 0.9948 | 3.29 | 0.76 | 9.6 | 0 |
8.0 | 0.2 | 0.3 | 8.1 | 0.037 | 42.0 | 130.0 | 0.99379 | 3.1 | 0.67 | 11.8 | 0 |
7.9 | 0.16 | 0.3 | 7.4 | 0.05 | 58.0 | 152.0 | 0.99612 | 3.12 | 0.37 | 9.5 | 0 |
6.6 | 0.3 | 0.3 | 4.8 | 0.17 | 60.0 | 166.0 | 0.9946 | 3.18 | 0.47 | 9.4 | 0 |
7.3 | 0.59 | 0.26 | 7.2 | 0.07 | 35.0 | 121.0 | 0.9981 | 3.37 | 0.49 | 9.4 | 1 |
7.0 | 0.15 | 0.34 | 1.4 | 0.039 | 21.0 | 177.0 | 0.9927 | 3.32 | 0.62 | 10.8 | 0 |
6.3 | 0.25 | 0.53 | 1.8 | 0.021 | 41.0 | 101.0 | 0.989315 | 3.19 | 0.31 | 13.0 | 0 |
6.7 | 0.25 | 0.33 | 2.9 | 0.057 | 52.0 | 173.0 | 0.9934 | 3.02 | 0.48 | 9.5 | 0 |
6.1 | 0.41 | 0.14 | 10.4 | 0.037 | 18.0 | 119.0 | 0.996 | 3.38 | 0.45 | 10.0 | 0 |
7.1 | 0.75 | 0.01 | 2.2 | 0.059 | 11.0 | 18.0 | 0.99242 | 3.39 | 0.4 | 12.8 | 1 |
7.1 | 0.37 | 0.67 | 10.5 | 0.045 | 49.0 | 155.0 | 0.9975 | 3.16 | 0.44 | 8.7 | 0 |
8.3 | 0.61 | 0.3 | 2.1 | 0.084 | 11.0 | 50.0 | 0.9972 | 3.4 | 0.61 | 10.2 | 1 |
7.5 | 0.33 | 0.28 | 4.9 | 0.042 | 21.0 | 155.0 | 0.99385 | 3.36 | 0.57 | 10.9 | 0 |
8.9 | 0.34 | 0.34 | 1.6 | 0.056 | 13.0 | 176.0 | 0.9946 | 3.14 | 0.47 | 9.7 | 0 |
6.5 | 0.28 | 0.35 | 9.8 | 0.067 | 61.0 | 180.0 | 0.9972 | 3.15 | 0.57 | 9.0 | 0 |
7.7 | 0.16 | 0.36 | 5.9 | 0.054 | 25.0 | 148.0 | 0.99578 | 3.25 | 0.54 | 10.2 | 0 |
7.5 | 0.52 | 0.16 | 1.9 | 0.085 | 12.0 | 35.0 | 0.9968 | 3.38 | 0.62 | 9.5 | 1 |
7.4 | 0.35 | 0.2 | 13.9 | 0.054 | 63.0 | 229.0 | 0.99888 | 3.11 | 0.5 | 8.9 | 0 |
7.3 | 0.29 | 0.37 | 8.3 | 0.044 | 45.0 | 227.0 | 0.9966 | 3.12 | 0.47 | 9.0 | 0 |
5.6 | 0.66 | 0.0 | 2.2 | 0.087 | 3.0 | 11.0 | 0.99378 | 3.71 | 0.63 | 12.8 | 1 |
5.9 | 0.65 | 0.23 | 5.0 | 0.035 | 20.0 | 128.0 | 0.99016 | 3.46 | 0.48 | 12.8 | 0 |
6.7 | 0.34 | 0.4 | 2.1 | 0.033 | 34.0 | 111.0 | 0.98924 | 2.97 | 0.48 | 12.2 | 0 |
7.4 | 0.29 | 0.29 | 1.6 | 0.045 | 53.0 | 180.0 | 0.9936 | 3.34 | 0.68 | 10.5 | 0 |
8.1 | 0.2 | 0.28 | 0.9 | 0.023 | 49.0 | 87.0 | 0.99062 | 2.92 | 0.36 | 11.1 | 0 |
7.2 | 0.37 | 0.32 | 2.0 | 0.062 | 15.0 | 28.0 | 0.9947 | 3.23 | 0.73 | 11.3 | 1 |
7.0 | 0.805 | 0.0 | 2.5 | 0.068 | 7.0 | 20.0 | 0.9969 | 3.48 | 0.56 | 9.6 | 1 |
4.9 | 0.335 | 0.14 | 1.3 | 0.036 | 69.0 | 168.0 | 0.99212 | 3.47 | 0.46 | 10.4666666666667 | 0 |
7.3 | 0.33 | 0.47 | 2.1 | 0.077 | 5.0 | 11.0 | 0.9958 | 3.33 | 0.53 | 10.3 | 1 |
8.8 | 0.28 | 0.45 | 6.0 | 0.022 | 14.0 | 49.0 | 0.9934 | 3.01 | 0.33 | 11.1 | 0 |
7.1 | 0.63 | 0.06 | 2.0 | 0.083 | 8.0 | 29.0 | 0.99855 | 3.67 | 0.73 | 9.6 | 1 |
6.9 | 0.29 | 0.4 | 19.45 | 0.043 | 36.0 | 156.0 | 0.9996 | 2.93 | 0.47 | 8.9 | 0 |
6.7 | 0.26 | 0.49 | 8.3 | 0.047 | 54.0 | 191.0 | 0.9954 | 3.23 | 0.4 | 10.3 | 0 |
7.7 | 0.23 | 0.37 | 1.8 | 0.046 | 23.0 | 60.0 | 0.9971 | 3.41 | 0.71 | 12.1 | 1 |
5.8 | 0.2 | 0.16 | 1.4 | 0.042 | 44.0 | 99.0 | 0.98912 | 3.23 | 0.37 | 12.2 | 0 |
7.4 | 0.635 | 0.1 | 2.4 | 0.08 | 16.0 | 33.0 | 0.99736 | 3.58 | 0.69 | 10.8 | 1 |
6.2 | 0.41 | 0.22 | 1.9 | 0.023 | 5.0 | 56.0 | 0.98928 | 3.04 | 0.79 | 13.0 | 0 |
7.7 | 0.38 | 0.4 | 2.0 | 0.038 | 28.0 | 152.0 | 0.9906 | 3.18 | 0.32 | 12.9 | 0 |
9.3 | 0.2 | 0.33 | 1.7 | 0.05 | 28.0 | 178.0 | 0.9954 | 3.16 | 0.43 | 9.0 | 0 |
8.2 | 0.3 | 0.44 | 12.4 | 0.043 | 52.0 | 154.0 | 0.99452 | 3.04 | 0.33 | 12.0 | 0 |
5.6 | 0.29 | 0.05 | 0.8 | 0.038 | 11.0 | 30.0 | 0.9924 | 3.36 | 0.35 | 9.2 | 0 |
9.8 | 0.98 | 0.32 | 2.3 | 0.078 | 35.0 | 152.0 | 0.998 | 3.25 | 0.48 | 9.4 | 1 |
6.2 | 0.29 | 0.23 | 12.4 | 0.048 | 33.0 | 201.0 | 0.99612 | 3.11 | 0.56 | 9.9 | 0 |
6.7 | 0.28 | 0.34 | 8.9 | 0.048 | 32.0 | 111.0 | 0.99455 | 3.25 | 0.54 | 11.0 | 0 |
7.2 | 0.21 | 0.34 | 1.1 | 0.046 | 25.0 | 80.0 | 0.992 | 3.25 | 0.4 | 11.3 | 0 |
7.0 | 0.43 | 0.3 | 2.0 | 0.085 | 6.0 | 39.0 | 0.99346 | 3.33 | 0.46 | 11.9 | 1 |
6.3 | 0.23 | 0.22 | 3.75 | 0.039 | 37.0 | 116.0 | 0.9927 | 3.23 | 0.5 | 10.7 | 0 |
6.4 | 0.69 | 0.0 | 1.65 | 0.055 | 7.0 | 12.0 | 0.99162 | 3.47 | 0.53 | 12.9 | 1 |
6.7 | 0.15 | 0.32 | 7.9 | 0.034 | 17.0 | 81.0 | 0.99512 | 3.29 | 0.31 | 10.0 | 0 |
6.3 | 0.26 | 0.25 | 7.8 | 0.058 | 44.0 | 166.0 | 0.9961 | 3.24 | 0.41 | 9.0 | 0 |
7.5 | 0.305 | 0.38 | 1.4 | 0.047 | 30.0 | 95.0 | 0.99158 | 3.22 | 0.52 | 11.5 | 0 |
7.9 | 0.19 | 0.45 | 1.5 | 0.045 | 17.0 | 96.0 | 0.9917 | 3.13 | 0.39 | 11.0 | 0 |
6.8 | 0.19 | 0.34 | 1.9 | 0.04 | 41.0 | 108.0 | 0.99 | 3.25 | 0.45 | 12.9 | 0 |
6.7 | 0.3 | 0.45 | 10.6 | 0.032 | 56.0 | 212.0 | 0.997 | 3.22 | 0.59 | 9.5 | 0 |
5.6 | 0.15 | 0.31 | 5.3 | 0.038 | 8.0 | 79.0 | 0.9923 | 3.3 | 0.39 | 10.5 | 0 |
6.6 | 0.61 | 0.01 | 1.9 | 0.08 | 8.0 | 25.0 | 0.99746 | 3.69 | 0.73 | 10.5 | 1 |
6.6 | 0.23 | 0.3 | 4.6 | 0.06 | 29.0 | 154.0 | 0.99142 | 3.23 | 0.49 | 12.2 | 0 |
6.8 | 0.28 | 0.44 | 11.5 | 0.04 | 58.0 | 223.0 | 0.9969 | 3.22 | 0.56 | 9.5 | 0 |
5.2 | 0.405 | 0.15 | 1.45 | 0.038 | 10.0 | 44.0 | 0.99125 | 3.52 | 0.4 | 11.6 | 0 |
7.0 | 0.31 | 0.39 | 7.5 | 0.055 | 42.0 | 218.0 | 0.99652 | 3.37 | 0.54 | 10.3 | 0 |
7.4 | 0.35 | 0.33 | 2.4 | 0.068 | 9.0 | 26.0 | 0.9947 | 3.36 | 0.6 | 11.9 | 1 |
6.9 | 0.22 | 0.31 | 6.3 | 0.029 | 41.0 | 131.0 | 0.99326 | 3.08 | 0.49 | 10.8 | 0 |
8.1 | 0.575 | 0.22 | 2.1 | 0.077 | 12.0 | 65.0 | 0.9967 | 3.29 | 0.51 | 9.2 | 1 |
6.7 | 0.24 | 0.36 | 8.4 | 0.042 | 42.0 | 123.0 | 0.99473 | 3.34 | 0.52 | 10.9 | 0 |
5.8 | 0.31 | 0.32 | 4.5 | 0.024 | 28.0 | 94.0 | 0.98906 | 3.25 | 0.52 | 13.7 | 0 |
6.4 | 0.24 | 0.26 | 8.2 | 0.054 | 47.0 | 182.0 | 0.99538 | 3.12 | 0.5 | 9.5 | 0 |
6.8 | 0.25 | 0.24 | 1.6 | 0.045 | 39.0 | 164.0 | 0.99402 | 3.53 | 0.58 | 10.8 | 0 |
8.9 | 0.33 | 0.34 | 1.4 | 0.056 | 14.0 | 171.0 | 0.9946 | 3.13 | 0.47 | 9.7 | 0 |
6.8 | 0.73 | 0.2 | 6.6 | 0.054 | 25.0 | 65.0 | 0.99324 | 3.12 | 0.28 | 11.1 | 0 |
7.3 | 0.2 | 0.39 | 2.3 | 0.048 | 24.0 | 87.0 | 0.99044 | 2.94 | 0.35 | 12.0 | 0 |
6.4 | 0.3 | 0.33 | 5.2 | 0.05 | 30.0 | 137.0 | 0.99304 | 3.26 | 0.58 | 11.1 | 0 |
8.0 | 0.62 | 0.35 | 2.8 | 0.086 | 28.0 | 52.0 | 0.997 | 3.31 | 0.62 | 10.8 | 1 |
7.5 | 0.17 | 0.32 | 1.7 | 0.04 | 51.0 | 148.0 | 0.9916 | 3.21 | 0.44 | 11.5 | 0 |
5.6 | 0.175 | 0.29 | 0.8 | 0.043 | 20.0 | 67.0 | 0.99112 | 3.28 | 0.48 | 9.9 | 0 |
4.4 | 0.46 | 0.1 | 2.8 | 0.024 | 31.0 | 111.0 | 0.98816 | 3.48 | 0.34 | 13.1 | 0 |
7.5 | 0.3 | 0.32 | 1.4 | 0.032 | 31.0 | 161.0 | 0.99154 | 2.95 | 0.42 | 10.5 | 0 |
6.3 | 0.26 | 0.42 | 7.1 | 0.045 | 62.0 | 209.0 | 0.99544 | 3.2 | 0.53 | 9.5 | 0 |
6.0 | 0.31 | 0.24 | 3.3 | 0.041 | 25.0 | 143.0 | 0.9914 | 3.31 | 0.44 | 11.3 | 0 |
11.6 | 0.47 | 0.44 | 1.6 | 0.147 | 36.0 | 51.0 | 0.99836 | 3.38 | 0.86 | 9.9 | 1 |
6.7 | 0.35 | 0.48 | 8.8 | 0.056 | 35.0 | 167.0 | 0.99628 | 3.04 | 0.47 | 9.4 | 0 |
9.0 | 0.62 | 0.04 | 1.9 | 0.146 | 27.0 | 90.0 | 0.9984 | 3.16 | 0.7 | 9.4 | 1 |
6.5 | 0.23 | 0.25 | 17.3 | 0.046 | 15.0 | 110.0 | 0.99828 | 3.15 | 0.42 | 9.2 | 0 |
9.8 | 0.36 | 0.46 | 10.5 | 0.038 | 4.0 | 83.0 | 0.9956 | 2.89 | 0.3 | 10.1 | 0 |
7.9 | 0.35 | 0.46 | 3.6 | 0.078 | 15.0 | 37.0 | 0.9973 | 3.35 | 0.86 | 12.8 | 1 |
7.1 | 0.24 | 0.41 | 17.8 | 0.046 | 39.0 | 145.0 | 0.9998 | 3.32 | 0.39 | 8.7 | 0 |
5.9 | 0.3 | 0.29 | 1.1 | 0.036 | 23.0 | 56.0 | 0.9904 | 3.19 | 0.38 | 11.3 | 0 |
6.7 | 0.7 | 0.08 | 3.75 | 0.067 | 8.0 | 16.0 | 0.99334 | 3.43 | 0.52 | 12.6 | 1 |
6.4 | 0.57 | 0.12 | 2.3 | 0.12 | 25.0 | 36.0 | 0.99519 | 3.47 | 0.71 | 11.3 | 1 |
6.0 | 0.26 | 0.29 | 3.1 | 0.041 | 37.0 | 144.0 | 0.98944 | 3.22 | 0.39 | 12.8 | 0 |
6.9 | 0.17 | 0.22 | 4.6 | 0.064 | 55.0 | 152.0 | 0.9952 | 3.29 | 0.37 | 9.3 | 0 |
5.6 | 0.35 | 0.37 | 1.0 | 0.038 | 6.0 | 72.0 | 0.9902 | 3.37 | 0.34 | 11.4 | 0 |
5.3 | 0.16 | 0.39 | 1.0 | 0.028 | 40.0 | 101.0 | 0.99156 | 3.57 | 0.59 | 10.6 | 0 |
6.2 | 0.31 | 0.23 | 3.3 | 0.052 | 34.0 | 113.0 | 0.99429 | 3.16 | 0.48 | 8.4 | 0 |
7.0 | 0.28 | 0.39 | 8.7 | 0.051 | 32.0 | 141.0 | 0.9961 | 3.38 | 0.53 | 10.5 | 0 |
7.4 | 0.64 | 0.17 | 5.4 | 0.168 | 52.0 | 98.0 | 0.99736 | 3.28 | 0.5 | 9.5 | 1 |
8.0 | 0.58 | 0.16 | 2.0 | 0.12 | 3.0 | 7.0 | 0.99454 | 3.22 | 0.58 | 11.2 | 1 |
6.1 | 0.38 | 0.15 | 1.8 | 0.072 | 6.0 | 19.0 | 0.9955 | 3.42 | 0.57 | 9.4 | 1 |
6.5 | 0.39 | 0.81 | 1.2 | 0.217 | 14.0 | 74.0 | 0.9936 | 3.08 | 0.53 | 9.5 | 0 |
8.2 | 0.52 | 0.34 | 1.2 | 0.042 | 18.0 | 167.0 | 0.99366 | 3.24 | 0.39 | 10.6 | 0 |
6.2 | 0.23 | 0.36 | 17.2 | 0.039 | 37.0 | 130.0 | 0.99946 | 3.23 | 0.43 | 8.8 | 0 |
8.4 | 0.22 | 0.28 | 18.8 | 0.028 | 55.0 | 130.0 | 0.998 | 2.96 | 0.35 | 11.6 | 0 |
7.0 | 0.48 | 0.12 | 4.5 | 0.05 | 23.0 | 86.0 | 0.99398 | 2.86 | 0.35 | 9.0 | 0 |
7.7 | 0.34 | 0.28 | 11.0 | 0.04 | 31.0 | 117.0 | 0.99815 | 3.27 | 0.29 | 9.2 | 0 |
9.9 | 0.54 | 0.45 | 2.3 | 0.071 | 16.0 | 40.0 | 0.9991 | 3.39 | 0.62 | 9.4 | 1 |
6.3 | 0.27 | 0.37 | 7.9 | 0.047 | 58.0 | 215.0 | 0.99542 | 3.19 | 0.48 | 9.5 | 0 |
9.6 | 0.29 | 0.46 | 1.45 | 0.039 | 77.5 | 223.0 | 0.9944 | 2.92 | 0.46 | 9.5 | 0 |
9.4 | 0.16 | 0.23 | 1.6 | 0.042 | 14.0 | 67.0 | 0.9942 | 3.07 | 0.32 | 9.5 | 0 |
6.8 | 0.38 | 0.29 | 9.9 | 0.037 | 40.0 | 146.0 | 0.99326 | 3.11 | 0.37 | 11.5 | 0 |
6.0 | 0.28 | 0.29 | 19.3 | 0.051 | 36.0 | 174.0 | 0.99911 | 3.14 | 0.5 | 9.0 | 0 |
6.3 | 0.24 | 0.29 | 1.6 | 0.052 | 48.0 | 185.0 | 0.9934 | 3.21 | 0.5 | 9.4 | 0 |
5.6 | 0.205 | 0.16 | 12.55 | 0.051 | 31.0 | 115.0 | 0.99564 | 3.4 | 0.38 | 10.8 | 0 |
8.1 | 0.36 | 0.59 | 13.6 | 0.051 | 60.0 | 134.0 | 0.99886 | 2.96 | 0.39 | 8.7 | 0 |
6.6 | 0.22 | 0.37 | 15.4 | 0.035 | 62.0 | 153.0 | 0.99845 | 3.02 | 0.4 | 9.3 | 0 |
7.7 | 0.29 | 0.48 | 2.3 | 0.049 | 36.0 | 178.0 | 0.9931 | 3.17 | 0.64 | 10.6 | 0 |
6.5 | 0.21 | 0.37 | 2.5 | 0.048 | 70.0 | 138.0 | 0.9917 | 3.33 | 0.75 | 11.4 | 0 |
6.8 | 0.59 | 0.06 | 6.0 | 0.06 | 11.0 | 18.0 | 0.9962 | 3.41 | 0.59 | 10.8 | 1 |
6.6 | 0.16 | 0.4 | 1.5 | 0.044 | 48.0 | 143.0 | 0.9912 | 3.54 | 0.52 | 12.4 | 0 |
7.6 | 0.39 | 0.32 | 3.6 | 0.035 | 22.0 | 93.0 | 0.99144 | 3.08 | 0.6 | 12.5 | 0 |
6.5 | 0.24 | 0.38 | 1.0 | 0.027 | 31.0 | 90.0 | 0.98926 | 3.24 | 0.36 | 12.3 | 0 |
9.8 | 0.44 | 0.47 | 2.5 | 0.063 | 9.0 | 28.0 | 0.9981 | 3.24 | 0.65 | 10.8 | 1 |
7.1 | 0.31 | 0.5 | 14.5 | 0.059 | 6.0 | 148.0 | 0.9983 | 2.94 | 0.44 | 9.1 | 0 |
6.8 | 0.32 | 0.28 | 4.8 | 0.034 | 25.0 | 100.0 | 0.99026 | 3.08 | 0.47 | 12.4 | 0 |
6.2 | 0.56 | 0.09 | 1.7 | 0.053 | 24.0 | 32.0 | 0.99402 | 3.54 | 0.6 | 11.3 | 1 |
7.7 | 0.46 | 0.18 | 3.3 | 0.054 | 18.0 | 143.0 | 0.99392 | 3.12 | 0.51 | 10.8 | 0 |
8.2 | 0.29 | 0.33 | 9.1 | 0.036 | 28.0 | 118.0 | 0.9953 | 2.96 | 0.4 | 10.9 | 0 |
10.6 | 1.02 | 0.43 | 2.9 | 0.076 | 26.0 | 88.0 | 0.9984 | 3.08 | 0.57 | 10.1 | 1 |
6.2 | 0.36 | 0.26 | 13.2 | 0.051 | 54.0 | 201.0 | 0.9976 | 3.25 | 0.46 | 9.0 | 0 |
7.6 | 0.48 | 0.28 | 10.4 | 0.049 | 57.0 | 205.0 | 0.99748 | 3.24 | 0.45 | 9.3 | 0 |
5.8 | 0.28 | 0.34 | 4.0 | 0.031 | 40.0 | 99.0 | 0.9896 | 3.39 | 0.39 | 12.8 | 0 |
7.5 | 0.4 | 1.0 | 19.5 | 0.041 | 33.0 | 148.0 | 0.9977 | 3.24 | 0.38 | 12.0 | 0 |
8.7 | 0.23 | 0.32 | 13.4 | 0.044 | 35.0 | 169.0 | 0.99975 | 3.12 | 0.47 | 8.8 | 0 |
7.0 | 0.27 | 0.48 | 6.1 | 0.042 | 60.0 | 184.0 | 0.99566 | 3.2 | 0.5 | 9.4 | 0 |
6.6 | 0.39 | 0.39 | 11.9 | 0.057 | 51.0 | 221.0 | 0.99851 | 3.26 | 0.51 | 8.9 | 0 |
7.8 | 0.56 | 0.19 | 1.8 | 0.104 | 12.0 | 47.0 | 0.9964 | 3.19 | 0.93 | 9.5 | 1 |
6.3 | 0.18 | 0.24 | 3.4 | 0.053 | 20.0 | 119.0 | 0.99373 | 3.11 | 0.52 | 9.2 | 0 |
9.0 | 0.38 | 0.53 | 2.1 | 0.102 | 19.0 | 76.0 | 0.99001 | 2.93 | 0.57 | 12.9 | 0 |
6.6 | 0.35 | 0.29 | 14.4 | 0.044 | 54.0 | 177.0 | 0.9991 | 3.17 | 0.58 | 8.9 | 0 |
6.4 | 0.38 | 0.14 | 2.2 | 0.038 | 15.0 | 25.0 | 0.99514 | 3.44 | 0.65 | 11.1 | 1 |
6.2 | 0.21 | 0.24 | 1.2 | 0.051 | 31.0 | 95.0 | 0.99036 | 3.24 | 0.57 | 11.3 | 0 |
6.8 | 0.26 | 0.34 | 15.1 | 0.06 | 42.0 | 162.0 | 0.99705 | 3.24 | 0.52 | 10.5 | 0 |
8.8 | 0.6 | 0.29 | 2.2 | 0.098 | 5.0 | 15.0 | 0.9988 | 3.36 | 0.49 | 9.1 | 1 |
6.7 | 0.32 | 0.44 | 2.4 | 0.061 | 24.0 | 34.0 | 0.99484 | 3.29 | 0.8 | 11.6 | 1 |
6.2 | 0.32 | 0.12 | 4.8 | 0.054 | 6.0 | 97.0 | 0.99424 | 3.16 | 0.5 | 9.3 | 0 |
7.15 | 0.17 | 0.24 | 9.6 | 0.119 | 56.0 | 178.0 | 0.99578 | 3.15 | 0.44 | 10.2 | 0 |
6.3 | 0.27 | 0.37 | 7.9 | 0.047 | 58.0 | 215.0 | 0.99542 | 3.19 | 0.48 | 9.5 | 0 |
6.6 | 0.27 | 0.31 | 5.3 | 0.137 | 35.0 | 163.0 | 0.9951 | 3.2 | 0.38 | 9.3 | 0 |
7.4 | 0.3 | 0.49 | 8.2 | 0.055 | 49.0 | 188.0 | 0.9974 | 3.52 | 0.58 | 9.7 | 0 |
7.5 | 0.29 | 0.67 | 8.1 | 0.037 | 53.0 | 166.0 | 0.9966 | 2.9 | 0.41 | 8.9 | 0 |
7.3 | 0.55 | 0.03 | 1.6 | 0.072 | 17.0 | 42.0 | 0.9956 | 3.37 | 0.48 | 9.0 | 1 |
8.2 | 0.18 | 0.38 | 1.1 | 0.04 | 41.0 | 92.0 | 0.99062 | 2.88 | 0.6 | 12.0 | 0 |
6.9 | 0.4 | 0.24 | 2.5 | 0.083 | 30.0 | 45.0 | 0.9959 | 3.26 | 0.58 | 10.0 | 1 |
7.0 | 0.26 | 0.26 | 10.8 | 0.039 | 37.0 | 184.0 | 0.99787 | 3.47 | 0.58 | 10.3 | 0 |
7.8 | 0.4 | 0.26 | 9.5 | 0.059 | 32.0 | 178.0 | 0.9955 | 3.04 | 0.43 | 10.9 | 0 |
6.4 | 0.15 | 0.44 | 1.2 | 0.043 | 67.0 | 150.0 | 0.9907 | 3.14 | 0.73 | 11.2 | 0 |
7.4 | 0.24 | 0.42 | 14.0 | 0.066 | 48.0 | 198.0 | 0.9979 | 2.89 | 0.42 | 8.9 | 0 |
9.2 | 0.23 | 0.35 | 10.7 | 0.037 | 34.0 | 145.0 | 0.9981 | 3.09 | 0.32 | 9.7 | 0 |
8.0 | 0.57 | 0.39 | 3.9 | 0.034 | 22.0 | 122.0 | 0.9917 | 3.29 | 0.67 | 12.8 | 0 |
7.1 | 0.18 | 0.26 | 1.3 | 0.041 | 20.0 | 71.0 | 0.9926 | 3.04 | 0.74 | 9.9 | 0 |
8.1 | 0.38 | 0.48 | 1.8 | 0.157 | 5.0 | 17.0 | 0.9976 | 3.3 | 1.05 | 9.4 | 1 |
7.1 | 0.21 | 0.27 | 8.6 | 0.056 | 26.0 | 111.0 | 0.9956 | 2.95 | 0.52 | 9.5 | 0 |
7.1 | 0.18 | 0.39 | 14.5 | 0.051 | 48.0 | 156.0 | 0.99947 | 3.35 | 0.78 | 9.1 | 0 |
5.7 | 0.36 | 0.21 | 6.7 | 0.038 | 51.0 | 166.0 | 0.9941 | 3.29 | 0.63 | 10.0 | 0 |
7.0 | 0.65 | 0.02 | 2.1 | 0.066 | 8.0 | 25.0 | 0.9972 | 3.47 | 0.67 | 9.5 | 1 |
6.9 | 0.4 | 0.14 | 2.4 | 0.085 | 21.0 | 40.0 | 0.9968 | 3.43 | 0.63 | 9.7 | 1 |
7.5 | 0.32 | 0.24 | 4.6 | 0.053 | 8.0 | 134.0 | 0.9958 | 3.14 | 0.5 | 9.1 | 0 |
7.0 | 0.3 | 0.38 | 14.9 | 0.032 | 60.0 | 181.0 | 0.9983 | 3.18 | 0.61 | 9.3 | 0 |
6.7 | 0.13 | 0.57 | 6.6 | 0.056 | 60.0 | 150.0 | 0.99548 | 2.96 | 0.43 | 9.4 | 0 |
8.0 | 0.28 | 0.42 | 7.1 | 0.045 | 41.0 | 169.0 | 0.9959 | 3.17 | 0.43 | 10.6 | 0 |
7.3 | 0.19 | 0.27 | 13.9 | 0.057 | 45.0 | 155.0 | 0.99807 | 2.94 | 0.41 | 8.8 | 0 |
5.7 | 0.28 | 0.28 | 2.2 | 0.019 | 15.0 | 65.0 | 0.9902 | 3.06 | 0.52 | 11.2 | 0 |
7.5 | 0.57 | 0.02 | 2.6 | 0.077 | 11.0 | 35.0 | 0.99557 | 3.36 | 0.62 | 10.8 | 1 |
6.6 | 0.55 | 0.01 | 2.7 | 0.034 | 56.0 | 122.0 | 0.9906 | 3.15 | 0.3 | 11.9 | 0 |
7.6 | 0.39 | 0.31 | 2.3 | 0.082 | 23.0 | 71.0 | 0.9982 | 3.52 | 0.65 | 9.7 | 1 |
6.6 | 0.28 | 0.42 | 8.2 | 0.044 | 60.0 | 196.0 | 0.99562 | 3.14 | 0.48 | 9.4 | 0 |
7.9 | 0.44 | 0.37 | 5.85 | 0.033 | 27.0 | 93.0 | 0.992 | 3.16 | 0.54 | 12.6 | 0 |
8.1 | 0.27 | 0.33 | 1.3 | 0.045 | 26.0 | 100.0 | 0.99066 | 2.98 | 0.44 | 12.4 | 0 |
6.2 | 0.37 | 0.24 | 6.1 | 0.032 | 19.0 | 86.0 | 0.98934 | 3.04 | 0.26 | 13.4 | 0 |
5.9 | 0.17 | 0.29 | 3.1 | 0.03 | 32.0 | 123.0 | 0.98913 | 3.41 | 0.33 | 13.7 | 0 |
7.7 | 0.965 | 0.1 | 2.1 | 0.112 | 11.0 | 22.0 | 0.9963 | 3.26 | 0.5 | 9.5 | 1 |
7.0 | 0.5 | 0.14 | 1.8 | 0.078 | 10.0 | 23.0 | 0.99636 | 3.53 | 0.61 | 10.4 | 1 |
6.6 | 0.28 | 0.42 | 8.2 | 0.044 | 60.0 | 196.0 | 0.99562 | 3.14 | 0.48 | 9.4 | 0 |
7.3 | 0.28 | 0.35 | 1.6 | 0.054 | 31.0 | 148.0 | 0.99178 | 3.18 | 0.47 | 10.7 | 0 |
6.8 | 0.27 | 0.3 | 13.0 | 0.047 | 69.0 | 160.0 | 0.99705 | 3.16 | 0.5 | 9.6 | 0 |
6.4 | 0.24 | 0.32 | 14.9 | 0.047 | 54.0 | 162.0 | 0.9968 | 3.28 | 0.5 | 10.2 | 0 |
6.8 | 0.32 | 0.37 | 3.4 | 0.023 | 19.0 | 87.0 | 0.9902 | 3.14 | 0.53 | 12.7 | 0 |
7.5 | 0.17 | 0.71 | 11.8 | 0.038 | 52.0 | 148.0 | 0.99801 | 3.03 | 0.46 | 8.9 | 0 |
7.6 | 0.48 | 0.37 | 1.2 | 0.034 | 5.0 | 57.0 | 0.99256 | 3.05 | 0.54 | 10.4 | 0 |
6.0 | 0.43 | 0.34 | 7.6 | 0.045 | 25.0 | 118.0 | 0.99222 | 3.03 | 0.37 | 11.0 | 0 |
8.4 | 0.35 | 0.56 | 13.8 | 0.048 | 55.0 | 190.0 | 0.9993 | 3.07 | 0.58 | 9.4 | 0 |
6.1 | 0.32 | 0.25 | 1.7 | 0.034 | 37.0 | 136.0 | 0.992 | 3.47 | 0.5 | 10.8 | 0 |
6.7 | 0.24 | 0.33 | 12.3 | 0.046 | 31.0 | 145.0 | 0.9983 | 3.36 | 0.4 | 9.5 | 0 |
6.7 | 0.23 | 0.33 | 1.8 | 0.036 | 23.0 | 96.0 | 0.9925 | 3.32 | 0.4 | 10.8 | 0 |
6.3 | 0.27 | 0.25 | 5.8 | 0.038 | 52.0 | 155.0 | 0.995 | 3.28 | 0.38 | 9.4 | 0 |
6.9 | 0.21 | 0.81 | 1.1 | 0.137 | 52.0 | 123.0 | 0.9932 | 3.03 | 0.39 | 9.2 | 0 |
8.0 | 0.25 | 0.13 | 17.2 | 0.036 | 49.0 | 219.0 | 0.9996 | 2.96 | 0.46 | 9.7 | 0 |
5.7 | 0.28 | 0.35 | 1.2 | 0.052 | 39.0 | 141.0 | 0.99108 | 3.44 | 0.69 | 11.3 | 0 |
8.3 | 0.66 | 0.15 | 1.9 | 0.079 | 17.0 | 42.0 | 0.9972 | 3.31 | 0.54 | 9.6 | 1 |
6.6 | 0.17 | 0.3 | 1.1 | 0.031 | 13.0 | 73.0 | 0.99095 | 3.17 | 0.58 | 11.0 | 0 |
7.9 | 0.54 | 0.34 | 2.5 | 0.076 | 8.0 | 17.0 | 0.99235 | 3.2 | 0.72 | 13.1 | 1 |
8.3 | 0.18 | 0.3 | 1.1 | 0.033 | 20.0 | 57.0 | 0.99109 | 3.02 | 0.51 | 11.0 | 0 |
10.9 | 0.53 | 0.49 | 4.6 | 0.118 | 10.0 | 17.0 | 1.0002 | 3.07 | 0.56 | 11.7 | 1 |
7.2 | 0.36 | 0.46 | 2.1 | 0.074 | 24.0 | 44.0 | 0.99534 | 3.4 | 0.85 | 11.0 | 1 |
10.6 | 0.31 | 0.49 | 2.2 | 0.063 | 18.0 | 40.0 | 0.9976 | 3.14 | 0.51 | 9.8 | 1 |
7.3 | 0.22 | 0.4 | 14.75 | 0.042 | 44.5 | 129.5 | 0.9998 | 3.36 | 0.41 | 9.1 | 0 |
8.2 | 0.31 | 0.43 | 7.0 | 0.047 | 18.0 | 87.0 | 0.99628 | 3.23 | 0.64 | 10.6 | 0 |
6.5 | 0.29 | 0.25 | 10.6 | 0.039 | 32.0 | 120.0 | 0.9962 | 3.31 | 0.34 | 10.1 | 0 |
9.1 | 0.22 | 0.24 | 2.1 | 0.078 | 1.0 | 28.0 | 0.999 | 3.41 | 0.87 | 10.3 | 1 |
7.2 | 0.23 | 0.19 | 13.7 | 0.052 | 47.0 | 197.0 | 0.99865 | 3.12 | 0.53 | 9.0 | 0 |
7.1 | 0.46 | 0.14 | 2.8 | 0.076 | 15.0 | 37.0 | 0.99624 | 3.36 | 0.49 | 10.7 | 1 |
6.9 | 0.52 | 0.25 | 2.6 | 0.081 | 10.0 | 37.0 | 0.99685 | 3.46 | 0.5 | 11.0 | 1 |
7.1 | 0.32 | 0.24 | 13.1 | 0.05 | 52.0 | 204.0 | 0.998 | 3.1 | 0.49 | 8.8 | 0 |
6.8 | 0.21 | 0.42 | 1.2 | 0.045 | 24.0 | 126.0 | 0.99234 | 3.09 | 0.87 | 10.9 | 0 |
8.0 | 0.2 | 0.4 | 5.2 | 0.055 | 41.0 | 167.0 | 0.9953 | 3.18 | 0.4 | 10.6 | 0 |
7.4 | 0.18 | 0.3 | 8.8 | 0.064 | 26.0 | 103.0 | 0.9961 | 2.94 | 0.56 | 9.3 | 0 |
6.8 | 0.22 | 0.31 | 6.9 | 0.037 | 33.0 | 121.0 | 0.99176 | 3.02 | 0.39 | 11.9 | 0 |
5.0 | 0.2 | 0.4 | 1.9 | 0.015 | 20.0 | 98.0 | 0.9897 | 3.37 | 0.55 | 12.05 | 0 |
6.3 | 0.24 | 0.22 | 11.9 | 0.05 | 65.0 | 179.0 | 0.99659 | 3.06 | 0.58 | 9.3 | 0 |
7.4 | 0.62 | 0.05 | 1.9 | 0.068 | 24.0 | 42.0 | 0.9961 | 3.42 | 0.57 | 11.5 | 1 |
9.6 | 0.68 | 0.24 | 2.2 | 0.087 | 5.0 | 28.0 | 0.9988 | 3.14 | 0.6 | 10.2 | 1 |
6.5 | 0.29 | 0.52 | 7.9 | 0.049 | 35.0 | 192.0 | 0.99551 | 3.16 | 0.51 | 9.5 | 0 |
7.0 | 0.46 | 0.2 | 16.7 | 0.046 | 50.0 | 184.0 | 0.99898 | 3.08 | 0.56 | 9.4 | 0 |
8.4 | 0.65 | 0.6 | 2.1 | 0.112 | 12.0 | 90.0 | 0.9973 | 3.2 | 0.52 | 9.2 | 1 |
7.8 | 0.39 | 0.26 | 9.9 | 0.059 | 33.0 | 181.0 | 0.9955 | 3.04 | 0.42 | 10.9 | 0 |
6.6 | 0.725 | 0.09 | 5.5 | 0.117 | 9.0 | 17.0 | 0.99655 | 3.35 | 0.49 | 10.8 | 1 |
5.8 | 0.36 | 0.5 | 1.0 | 0.127 | 63.0 | 178.0 | 0.99212 | 3.1 | 0.45 | 9.7 | 0 |
9.3 | 0.36 | 0.39 | 1.5 | 0.08 | 41.0 | 55.0 | 0.99652 | 3.47 | 0.73 | 10.9 | 1 |
7.5 | 0.18 | 0.31 | 11.7 | 0.051 | 24.0 | 94.0 | 0.997 | 3.19 | 0.44 | 9.5 | 0 |
8.0 | 0.4 | 0.33 | 7.7 | 0.034 | 27.0 | 98.0 | 0.9935 | 3.18 | 0.41 | 12.2 | 0 |
6.0 | 0.26 | 0.33 | 4.35 | 0.04 | 15.0 | 80.0 | 0.98934 | 3.29 | 0.5 | 12.7 | 0 |
8.8 | 0.55 | 0.04 | 2.2 | 0.119 | 14.0 | 56.0 | 0.9962 | 3.21 | 0.6 | 10.9 | 1 |
6.4 | 0.29 | 0.3 | 6.5 | 0.209 | 62.0 | 156.0 | 0.99478 | 3.1 | 0.4 | 9.4 | 0 |
7.3 | 0.13 | 0.31 | 2.3 | 0.054 | 22.0 | 104.0 | 0.9924 | 3.24 | 0.92 | 11.5 | 0 |
6.4 | 0.23 | 0.32 | 1.9 | 0.038 | 40.0 | 118.0 | 0.99074 | 3.32 | 0.53 | 11.8 | 0 |
7.4 | 0.21 | 0.27 | 1.2 | 0.041 | 27.0 | 99.0 | 0.9927 | 3.19 | 0.33 | 9.8 | 0 |
6.8 | 0.26 | 0.42 | 1.7 | 0.049 | 41.0 | 122.0 | 0.993 | 3.47 | 0.48 | 10.5 | 0 |
7.0 | 0.29 | 0.49 | 3.8 | 0.047 | 37.0 | 136.0 | 0.9938 | 2.95 | 0.4 | 9.4 | 0 |
9.4 | 0.28 | 0.3 | 1.6 | 0.045 | 36.0 | 139.0 | 0.99534 | 3.11 | 0.49 | 9.3 | 0 |
8.3 | 0.33 | 0.42 | 1.15 | 0.033 | 18.0 | 96.0 | 0.9911 | 3.2 | 0.32 | 12.4 | 0 |
5.3 | 0.275 | 0.24 | 7.4 | 0.038 | 28.0 | 114.0 | 0.99313 | 3.38 | 0.51 | 11.0 | 0 |
7.2 | 0.22 | 0.35 | 5.5 | 0.054 | 37.0 | 183.0 | 0.99474 | 3.08 | 0.5 | 10.3 | 0 |
6.6 | 0.32 | 0.26 | 4.6 | 0.031 | 26.0 | 120.0 | 0.99198 | 3.4 | 0.73 | 12.5 | 0 |
6.3 | 0.3 | 0.48 | 1.8 | 0.069 | 18.0 | 61.0 | 0.9959 | 3.44 | 0.78 | 10.3 | 1 |
7.8 | 0.44 | 0.28 | 2.7 | 0.1 | 18.0 | 95.0 | 0.9966 | 3.22 | 0.67 | 9.4 | 1 |
7.1 | 0.43 | 0.42 | 5.5 | 0.071 | 28.0 | 128.0 | 0.9973 | 3.42 | 0.71 | 10.5 | 1 |
5.9 | 0.24 | 0.26 | 12.3 | 0.053 | 34.0 | 134.0 | 0.9972 | 3.34 | 0.45 | 9.5 | 0 |
6.6 | 0.44 | 0.15 | 2.1 | 0.076 | 22.0 | 53.0 | 0.9957 | 3.32 | 0.62 | 9.3 | 1 |
6.7 | 0.19 | 0.39 | 1.0 | 0.032 | 14.0 | 71.0 | 0.98912 | 3.31 | 0.38 | 13.0 | 0 |
6.0 | 0.38 | 0.26 | 3.5 | 0.035 | 38.0 | 111.0 | 0.98872 | 3.18 | 0.47 | 13.6 | 0 |
6.8 | 0.5 | 0.11 | 1.5 | 0.075 | 16.0 | 49.0 | 0.99545 | 3.36 | 0.79 | 9.5 | 1 |
7.1 | 0.32 | 0.32 | 11.0 | 0.038 | 16.0 | 66.0 | 0.9937 | 3.24 | 0.4 | 11.5 | 0 |
7.8 | 0.4 | 0.49 | 7.8 | 0.06 | 34.0 | 162.0 | 0.9966 | 3.26 | 0.58 | 11.3 | 0 |
7.8 | 0.19 | 0.26 | 8.9 | 0.039 | 42.0 | 182.0 | 0.996 | 3.18 | 0.46 | 9.9 | 0 |
7.2 | 0.23 | 0.39 | 14.2 | 0.058 | 49.0 | 192.0 | 0.9979 | 2.98 | 0.48 | 9.0 | 0 |
7.3 | 0.91 | 0.1 | 1.8 | 0.074 | 20.0 | 56.0 | 0.99672 | 3.35 | 0.56 | 9.2 | 1 |
7.9 | 0.25 | 0.34 | 11.4 | 0.04 | 53.0 | 202.0 | 0.99708 | 3.11 | 0.57 | 9.6 | 0 |
6.7 | 0.24 | 0.46 | 2.2 | 0.033 | 19.0 | 111.0 | 0.99045 | 3.1 | 0.62 | 11.9 | 0 |
7.0 | 0.36 | 0.21 | 2.4 | 0.086 | 24.0 | 69.0 | 0.99556 | 3.4 | 0.53 | 10.1 | 1 |
6.7 | 0.5 | 0.36 | 11.5 | 0.096 | 18.0 | 92.0 | 0.99642 | 3.11 | 0.49 | 9.6 | 0 |
6.8 | 0.25 | 0.3 | 11.8 | 0.043 | 53.0 | 133.0 | 0.99524 | 3.03 | 0.58 | 10.4 | 0 |
7.0 | 0.14 | 0.28 | 1.3 | 0.026 | 10.0 | 56.0 | 0.99352 | 3.46 | 0.45 | 9.9 | 0 |
7.4 | 0.2 | 0.35 | 2.1 | 0.038 | 30.0 | 116.0 | 0.9949 | 3.49 | 0.77 | 10.3 | 0 |
6.6 | 0.7 | 0.08 | 2.6 | 0.106 | 14.0 | 27.0 | 0.99665 | 3.44 | 0.58 | 10.2 | 1 |
6.7 | 0.18 | 0.31 | 10.6 | 0.035 | 42.0 | 143.0 | 0.99572 | 3.08 | 0.49 | 9.8 | 0 |
7.5 | 0.38 | 0.33 | 5.0 | 0.045 | 30.0 | 131.0 | 0.9942 | 3.32 | 0.44 | 10.9 | 0 |
6.8 | 0.24 | 0.38 | 8.3 | 0.045 | 50.0 | 185.0 | 0.99578 | 3.15 | 0.5 | 9.5 | 0 |
9.2 | 0.25 | 0.34 | 1.2 | 0.026 | 31.0 | 93.0 | 0.9916 | 2.93 | 0.37 | 11.3 | 0 |
10.1 | 0.31 | 0.35 | 1.6 | 0.075 | 9.0 | 28.0 | 0.99672 | 3.24 | 0.83 | 11.2 | 1 |
6.15 | 0.21 | 0.37 | 3.2 | 0.021 | 20.0 | 80.0 | 0.99076 | 3.39 | 0.47 | 12.0 | 0 |
5.0 | 1.04 | 0.24 | 1.6 | 0.05 | 32.0 | 96.0 | 0.9934 | 3.74 | 0.62 | 11.5 | 1 |
7.1 | 0.38 | 0.4 | 2.2 | 0.042 | 54.0 | 201.0 | 0.99177 | 3.03 | 0.5 | 11.4 | 0 |
6.5 | 0.21 | 0.51 | 17.6 | 0.045 | 34.0 | 125.0 | 0.99966 | 3.2 | 0.47 | 8.8 | 0 |
6.8 | 0.14 | 0.18 | 1.4 | 0.047 | 30.0 | 90.0 | 0.99164 | 3.27 | 0.54 | 11.2 | 0 |
7.8 | 0.24 | 0.38 | 2.1 | 0.058 | 14.0 | 167.0 | 0.994 | 3.21 | 0.55 | 9.9 | 0 |
5.5 | 0.17 | 0.23 | 2.9 | 0.039 | 10.0 | 108.0 | 0.99243 | 3.28 | 0.5 | 10.0 | 0 |
6.7 | 0.56 | 0.09 | 2.9 | 0.079 | 7.0 | 22.0 | 0.99669 | 3.46 | 0.61 | 10.2 | 1 |
7.2 | 0.62 | 0.01 | 2.3 | 0.065 | 8.0 | 46.0 | 0.99332 | 3.32 | 0.51 | 11.8 | 1 |
6.7 | 0.22 | 0.33 | 1.2 | 0.036 | 36.0 | 86.0 | 0.99058 | 3.1 | 0.76 | 11.4 | 0 |
9.1 | 0.28 | 0.49 | 2.0 | 0.059 | 10.0 | 112.0 | 0.9958 | 3.15 | 0.46 | 10.1 | 0 |
6.4 | 0.31 | 0.26 | 13.2 | 0.046 | 57.0 | 205.0 | 0.9975 | 3.17 | 0.41 | 9.6 | 0 |
5.0 | 0.4 | 0.5 | 4.3 | 0.046 | 29.0 | 80.0 | 0.9902 | 3.49 | 0.66 | 13.6 | 1 |
8.0 | 0.17 | 0.29 | 2.4 | 0.029 | 52.0 | 119.0 | 0.98944 | 3.03 | 0.33 | 12.9 | 0 |
6.4 | 0.27 | 0.45 | 8.3 | 0.05 | 52.0 | 196.0 | 0.9955 | 3.18 | 0.48 | 9.5 | 0 |
8.4 | 0.2 | 0.38 | 11.8 | 0.055 | 51.0 | 170.0 | 1.0004 | 3.34 | 0.82 | 8.9 | 0 |
7.6 | 0.43 | 0.29 | 2.1 | 0.075 | 19.0 | 66.0 | 0.99718 | 3.4 | 0.64 | 9.5 | 1 |
6.4 | 0.31 | 0.28 | 1.5 | 0.037 | 12.0 | 119.0 | 0.9919 | 3.32 | 0.51 | 10.4 | 0 |
7.4 | 0.16 | 0.3 | 13.7 | 0.056 | 33.0 | 168.0 | 0.99825 | 2.9 | 0.44 | 8.7 | 0 |
7.3 | 0.21 | 0.29 | 1.6 | 0.034 | 29.0 | 118.0 | 0.9917 | 3.3 | 0.5 | 11.0 | 0 |
7.1 | 0.75 | 0.01 | 2.2 | 0.059 | 11.0 | 18.0 | 0.99242 | 3.39 | 0.4 | 12.8 | 1 |
5.8 | 0.26 | 0.3 | 2.6 | 0.034 | 75.0 | 129.0 | 0.9902 | 3.2 | 0.38 | 11.5 | 0 |
5.7 | 0.26 | 0.24 | 17.8 | 0.059 | 23.0 | 124.0 | 0.99773 | 3.3 | 0.5 | 10.1 | 0 |
6.5 | 0.26 | 0.34 | 16.3 | 0.051 | 56.0 | 197.0 | 1.0004 | 3.49 | 0.42 | 9.8 | 0 |
5.6 | 0.25 | 0.19 | 2.4 | 0.049 | 42.0 | 166.0 | 0.992 | 3.25 | 0.43 | 10.4 | 0 |
8.2 | 0.23 | 0.42 | 1.9 | 0.069 | 9.0 | 17.0 | 0.99376 | 3.21 | 0.54 | 12.3 | 1 |
5.7 | 0.22 | 0.2 | 16.0 | 0.044 | 41.0 | 113.0 | 0.99862 | 3.22 | 0.46 | 8.9 | 0 |
7.6 | 0.36 | 0.49 | 11.3 | 0.046 | 87.0 | 221.0 | 0.9984 | 3.01 | 0.43 | 9.2 | 0 |
6.2 | 0.46 | 0.17 | 1.6 | 0.073 | 7.0 | 11.0 | 0.99425 | 3.61 | 0.54 | 11.4 | 1 |
6.3 | 0.34 | 0.36 | 4.9 | 0.035 | 31.0 | 185.0 | 0.9946 | 3.15 | 0.49 | 9.7 | 0 |
6.9 | 0.21 | 0.24 | 1.8 | 0.021 | 17.0 | 80.0 | 0.98992 | 3.15 | 0.46 | 12.3 | 0 |
6.8 | 0.32 | 0.32 | 8.7 | 0.029 | 31.0 | 105.0 | 0.99146 | 3.0 | 0.34 | 12.3 | 0 |
6.7 | 0.31 | 0.3 | 2.4 | 0.038 | 30.0 | 83.0 | 0.98867 | 3.09 | 0.36 | 12.8 | 0 |
8.2 | 0.37 | 0.64 | 13.9 | 0.043 | 22.0 | 171.0 | 0.99873 | 2.99 | 0.8 | 9.3 | 0 |
6.6 | 0.31 | 0.07 | 1.5 | 0.033 | 55.0 | 144.0 | 0.99208 | 3.16 | 0.42 | 10.0 | 0 |
5.2 | 0.645 | 0.0 | 2.15 | 0.08 | 15.0 | 28.0 | 0.99444 | 3.78 | 0.61 | 12.5 | 1 |
6.3 | 0.32 | 0.26 | 12.0 | 0.049 | 63.0 | 170.0 | 0.9961 | 3.14 | 0.55 | 9.9 | 0 |
6.2 | 0.22 | 0.3 | 12.4 | 0.054 | 108.0 | 152.0 | 0.99728 | 3.1 | 0.47 | 9.5 | 0 |
7.4 | 0.18 | 0.29 | 1.4 | 0.042 | 34.0 | 101.0 | 0.99384 | 3.54 | 0.6 | 10.5 | 0 |
5.7 | 0.1 | 0.27 | 1.3 | 0.047 | 21.0 | 100.0 | 0.9928 | 3.27 | 0.46 | 9.5 | 0 |
10.5 | 0.42 | 0.66 | 2.95 | 0.116 | 12.0 | 29.0 | 0.997 | 3.24 | 0.75 | 11.7 | 1 |
8.3 | 0.49 | 0.43 | 2.5 | 0.036 | 32.0 | 116.0 | 0.9944 | 3.23 | 0.47 | 10.7 | 0 |
6.5 | 0.615 | 0.0 | 1.9 | 0.065 | 9.0 | 18.0 | 0.9972 | 3.46 | 0.65 | 9.2 | 1 |
8.9 | 0.27 | 0.28 | 0.8 | 0.024 | 29.0 | 128.0 | 0.98984 | 3.01 | 0.35 | 12.4 | 0 |
6.2 | 0.66 | 0.48 | 1.2 | 0.029 | 29.0 | 75.0 | 0.9892 | 3.33 | 0.39 | 12.8 | 0 |
6.1 | 0.68 | 0.52 | 1.4 | 0.037 | 32.0 | 123.0 | 0.99022 | 3.24 | 0.45 | 12.0 | 0 |
6.6 | 0.21 | 0.3 | 9.9 | 0.041 | 64.0 | 174.0 | 0.995 | 3.07 | 0.5 | 10.1 | 0 |
6.2 | 0.3 | 0.32 | 1.3 | 0.054 | 27.0 | 183.0 | 0.99266 | 3.3 | 0.43 | 10.1 | 0 |
5.7 | 0.21 | 0.25 | 1.1 | 0.035 | 26.0 | 81.0 | 0.9902 | 3.31 | 0.52 | 11.4 | 0 |
5.9 | 0.22 | 0.38 | 1.3 | 0.046 | 24.0 | 90.0 | 0.99232 | 3.2 | 0.47 | 10.0 | 0 |
7.8 | 0.57 | 0.31 | 1.8 | 0.069 | 26.0 | 120.0 | 0.99625 | 3.29 | 0.53 | 9.3 | 1 |
7.5 | 0.33 | 0.32 | 11.1 | 0.036 | 25.0 | 119.0 | 0.9962 | 3.15 | 0.34 | 10.5 | 0 |
6.6 | 0.24 | 0.22 | 12.3 | 0.051 | 35.0 | 146.0 | 0.99676 | 3.1 | 0.67 | 9.4 | 0 |
7.3 | 0.24 | 0.34 | 7.5 | 0.048 | 29.0 | 152.0 | 0.9962 | 3.1 | 0.54 | 9.0 | 0 |
6.6 | 0.23 | 0.2 | 11.4 | 0.044 | 45.0 | 131.0 | 0.99604 | 2.96 | 0.51 | 9.7 | 0 |
6.1 | 0.19 | 0.37 | 2.6 | 0.041 | 24.0 | 99.0 | 0.99153 | 3.18 | 0.5 | 10.9 | 0 |
6.0 | 0.24 | 0.33 | 2.5 | 0.026 | 31.0 | 85.0 | 0.99014 | 3.13 | 0.5 | 11.3 | 0 |
7.4 | 0.26 | 0.43 | 6.0 | 0.022 | 22.0 | 125.0 | 0.9928 | 3.13 | 0.55 | 11.5 | 0 |
6.0 | 0.24 | 0.32 | 6.3 | 0.03 | 34.0 | 129.0 | 0.9946 | 3.52 | 0.41 | 10.4 | 0 |
7.9 | 0.64 | 0.46 | 10.6 | 0.244 | 33.0 | 227.0 | 0.9983 | 2.87 | 0.74 | 9.1 | 0 |
8.7 | 0.23 | 0.32 | 13.4 | 0.044 | 35.0 | 169.0 | 0.99975 | 3.12 | 0.47 | 8.8 | 0 |
7.2 | 0.4 | 0.49 | 1.1 | 0.048 | 11.0 | 138.0 | 0.9929 | 3.01 | 0.42 | 9.3 | 0 |
6.9 | 0.32 | 0.16 | 1.4 | 0.051 | 15.0 | 96.0 | 0.994 | 3.22 | 0.38 | 9.5 | 0 |
6.4 | 0.24 | 0.28 | 11.5 | 0.05 | 34.0 | 163.0 | 0.9969 | 3.31 | 0.45 | 9.5 | 0 |
6.4 | 0.23 | 0.35 | 10.3 | 0.042 | 54.0 | 140.0 | 0.9967 | 3.23 | 0.47 | 9.2 | 0 |
7.4 | 0.26 | 0.43 | 6.0 | 0.022 | 22.0 | 125.0 | 0.9928 | 3.13 | 0.55 | 11.5 | 0 |
6.2 | 0.37 | 0.3 | 6.6 | 0.346 | 79.0 | 200.0 | 0.9954 | 3.29 | 0.58 | 9.6 | 0 |
8.5 | 0.18 | 0.3 | 1.1 | 0.028 | 34.0 | 95.0 | 0.99272 | 2.83 | 0.36 | 10.0 | 0 |
6.8 | 0.27 | 0.29 | 4.6 | 0.046 | 6.0 | 88.0 | 0.99458 | 3.34 | 0.48 | 10.6 | 0 |
7.8 | 0.39 | 0.42 | 2.0 | 0.086 | 9.0 | 21.0 | 0.99526 | 3.39 | 0.66 | 11.6 | 1 |
6.4 | 0.33 | 0.44 | 8.9 | 0.055 | 52.0 | 164.0 | 0.99488 | 3.1 | 0.48 | 9.6 | 0 |
7.1 | 0.23 | 0.39 | 13.7 | 0.058 | 26.0 | 172.0 | 0.99755 | 2.9 | 0.46 | 9.0 | 0 |
9.1 | 0.52 | 0.33 | 1.3 | 0.07 | 9.0 | 30.0 | 0.9978 | 3.24 | 0.6 | 9.3 | 1 |
6.6 | 0.34 | 0.28 | 1.3 | 0.035 | 32.0 | 90.0 | 0.9916 | 3.1 | 0.42 | 10.7 | 0 |
6.9 | 0.55 | 0.15 | 2.2 | 0.076 | 19.0 | 40.0 | 0.9961 | 3.41 | 0.59 | 10.1 | 1 |
6.3 | 0.22 | 0.22 | 5.6 | 0.039 | 31.0 | 128.0 | 0.99296 | 3.12 | 0.46 | 10.4 | 0 |
5.8 | 0.335 | 0.14 | 5.8 | 0.046 | 49.0 | 197.0 | 0.9937 | 3.3 | 0.71 | 10.3 | 0 |
8.8 | 0.47 | 0.49 | 2.9 | 0.085 | 17.0 | 110.0 | 0.9982 | 3.29 | 0.6 | 9.8 | 1 |
6.9 | 0.19 | 0.6 | 4.0 | 0.037 | 6.0 | 122.0 | 0.99255 | 2.92 | 0.59 | 10.4 | 0 |
7.5 | 0.34 | 0.28 | 4.0 | 0.028 | 46.0 | 100.0 | 0.98958 | 3.2 | 0.5 | 13.2 | 0 |
7.0 | 0.78 | 0.08 | 2.0 | 0.093 | 10.0 | 19.0 | 0.9956 | 3.4 | 0.47 | 10.0 | 1 |
7.2 | 0.21 | 1.0 | 1.1 | 0.154 | 46.0 | 114.0 | 0.9931 | 2.95 | 0.43 | 9.2 | 0 |
6.8 | 0.23 | 0.3 | 6.95 | 0.044 | 42.0 | 179.0 | 0.9946 | 3.25 | 0.56 | 10.6 | 0 |
7.7 | 0.39 | 0.28 | 4.9 | 0.035 | 36.0 | 109.0 | 0.9918 | 3.19 | 0.58 | 12.2 | 0 |
5.8 | 0.28 | 0.18 | 1.2 | 0.058 | 7.0 | 108.0 | 0.99288 | 3.23 | 0.58 | 9.55 | 0 |
6.6 | 0.26 | 0.46 | 6.9 | 0.047 | 59.0 | 183.0 | 0.99594 | 3.2 | 0.45 | 9.3 | 0 |
6.6 | 0.24 | 0.38 | 8.0 | 0.042 | 56.0 | 187.0 | 0.99577 | 3.21 | 0.46 | 9.2 | 0 |
6.0 | 0.58 | 0.2 | 2.4 | 0.075 | 15.0 | 50.0 | 0.99467 | 3.58 | 0.67 | 12.5 | 1 |
6.1 | 0.21 | 0.19 | 1.4 | 0.046 | 51.0 | 131.0 | 0.99184 | 3.22 | 0.39 | 10.5 | 0 |
7.3 | 0.38 | 0.23 | 6.5 | 0.05 | 18.0 | 102.0 | 0.99304 | 3.1 | 0.55 | 11.2 | 0 |
7.4 | 0.16 | 0.27 | 15.5 | 0.05 | 25.0 | 135.0 | 0.9984 | 2.9 | 0.43 | 8.7 | 0 |
7.1 | 0.21 | 0.37 | 2.4 | 0.026 | 23.0 | 100.0 | 0.9903 | 3.15 | 0.38 | 11.4 | 0 |
8.8 | 0.34 | 0.33 | 9.7 | 0.036 | 46.0 | 172.0 | 0.9966 | 3.08 | 0.4 | 10.2 | 0 |
6.8 | 0.23 | 0.32 | 1.6 | 0.026 | 43.0 | 147.0 | 0.9904 | 3.29 | 0.54 | 12.5 | 0 |
6.0 | 0.34 | 0.29 | 6.1 | 0.046 | 29.0 | 134.0 | 0.99462 | 3.48 | 0.57 | 10.7 | 0 |
6.3 | 0.39 | 0.16 | 1.4 | 0.08 | 11.0 | 23.0 | 0.9955 | 3.34 | 0.56 | 9.3 | 1 |
6.5 | 0.24 | 0.32 | 7.6 | 0.038 | 48.0 | 203.0 | 0.9958 | 3.45 | 0.54 | 9.7 | 0 |
6.5 | 0.27 | 0.19 | 6.6 | 0.045 | 98.0 | 175.0 | 0.99364 | 3.16 | 0.34 | 10.1 | 0 |
5.7 | 0.43 | 0.3 | 5.7 | 0.039 | 24.0 | 98.0 | 0.992 | 3.54 | 0.61 | 12.3 | 0 |
8.6 | 0.485 | 0.29 | 4.1 | 0.026 | 19.0 | 101.0 | 0.9918 | 3.01 | 0.38 | 12.4 | 0 |
6.2 | 0.45 | 0.2 | 1.6 | 0.069 | 3.0 | 15.0 | 0.9958 | 3.41 | 0.56 | 9.2 | 1 |
5.9 | 0.27 | 0.27 | 9.0 | 0.051 | 43.0 | 136.0 | 0.9941 | 3.25 | 0.53 | 10.7 | 0 |
7.4 | 0.28 | 0.3 | 5.3 | 0.054 | 44.0 | 161.0 | 0.9941 | 3.12 | 0.48 | 10.3 | 0 |
8.2 | 0.37 | 0.36 | 1.0 | 0.034 | 17.0 | 93.0 | 0.9906 | 3.04 | 0.32 | 11.7 | 0 |
7.9 | 0.51 | 0.34 | 2.6 | 0.049 | 13.0 | 135.0 | 0.99335 | 3.09 | 0.51 | 10.0 | 0 |
6.2 | 0.34 | 0.3 | 11.1 | 0.047 | 28.0 | 237.0 | 0.9981 | 3.18 | 0.49 | 8.7 | 0 |
6.4 | 0.27 | 0.49 | 7.3 | 0.046 | 53.0 | 206.0 | 0.9956 | 3.24 | 0.43 | 9.2 | 0 |
7.1 | 0.26 | 0.32 | 14.45 | 0.074 | 29.0 | 107.0 | 0.998 | 2.96 | 0.42 | 9.2 | 0 |
7.9 | 0.3 | 0.68 | 8.3 | 0.05 | 37.5 | 278.0 | 0.99316 | 3.01 | 0.51 | 12.3 | 1 |
7.2 | 0.685 | 0.21 | 9.5 | 0.07 | 33.0 | 172.0 | 0.9971 | 3.0 | 0.55 | 9.1 | 0 |
5.3 | 0.24 | 0.33 | 1.3 | 0.033 | 25.0 | 97.0 | 0.9906 | 3.59 | 0.38 | 11.0 | 0 |
6.1 | 0.45 | 0.27 | 0.8 | 0.039 | 13.0 | 82.0 | 0.9927 | 3.23 | 0.32 | 9.5 | 0 |
5.7 | 0.26 | 0.25 | 10.4 | 0.02 | 7.0 | 57.0 | 0.994 | 3.39 | 0.37 | 10.6 | 0 |
7.6 | 0.48 | 0.31 | 9.4 | 0.046 | 6.0 | 194.0 | 0.99714 | 3.07 | 0.61 | 9.4 | 0 |
6.1 | 0.38 | 0.14 | 3.9 | 0.06 | 27.0 | 113.0 | 0.99344 | 3.07 | 0.34 | 9.2 | 0 |
6.6 | 0.21 | 0.39 | 2.3 | 0.041 | 31.0 | 102.0 | 0.99221 | 3.22 | 0.58 | 10.9 | 0 |
7.5 | 0.29 | 0.26 | 14.95 | 0.067 | 47.0 | 178.0 | 0.99838 | 3.04 | 0.49 | 9.2 | 0 |
5.7 | 0.22 | 0.2 | 16.0 | 0.044 | 41.0 | 113.0 | 0.99862 | 3.22 | 0.46 | 8.9 | 0 |
7.3 | 0.22 | 0.37 | 14.3 | 0.063 | 48.0 | 191.0 | 0.9978 | 2.89 | 0.38 | 9.0 | 0 |
5.6 | 0.19 | 0.47 | 4.5 | 0.03 | 19.0 | 112.0 | 0.9922 | 3.56 | 0.45 | 11.2 | 0 |
6.4 | 0.15 | 0.36 | 1.8 | 0.034 | 43.0 | 150.0 | 0.9922 | 3.42 | 0.69 | 11.0 | 0 |
6.3 | 0.31 | 0.3 | 10.0 | 0.046 | 49.0 | 212.0 | 0.9962 | 3.74 | 0.55 | 11.9 | 0 |
7.6 | 0.16 | 0.44 | 1.4 | 0.043 | 25.0 | 109.0 | 0.9932 | 3.11 | 0.75 | 10.3 | 0 |
8.3 | 0.21 | 0.4 | 1.6 | 0.032 | 35.0 | 110.0 | 0.9907 | 3.02 | 0.6 | 12.9 | 0 |
6.6 | 0.46 | 0.49 | 7.4 | 0.052 | 19.0 | 184.0 | 0.9956 | 3.11 | 0.38 | 9.0 | 0 |
7.2 | 0.34 | 0.32 | 2.5 | 0.09 | 43.0 | 113.0 | 0.9966 | 3.32 | 0.79 | 11.1 | 1 |
5.6 | 0.35 | 0.14 | 5.0 | 0.046 | 48.0 | 198.0 | 0.9937 | 3.3 | 0.71 | 10.3 | 0 |
5.7 | 0.245 | 0.33 | 1.1 | 0.049 | 28.0 | 150.0 | 0.9927 | 3.13 | 0.42 | 9.3 | 0 |
7.6 | 0.645 | 0.03 | 1.9 | 0.086 | 14.0 | 57.0 | 0.9969 | 3.37 | 0.46 | 10.3 | 1 |
7.6 | 0.32 | 0.58 | 16.75 | 0.05 | 43.0 | 163.0 | 0.9999 | 3.15 | 0.54 | 9.2 | 0 |
7.8 | 0.91 | 0.07 | 1.9 | 0.058 | 22.0 | 47.0 | 0.99525 | 3.51 | 0.43 | 10.7 | 1 |
7.8 | 0.27 | 0.35 | 1.2 | 0.05 | 36.0 | 140.0 | 0.99138 | 3.09 | 0.45 | 11.2 | 0 |
7.6 | 0.34 | 0.39 | 7.6 | 0.04 | 45.0 | 215.0 | 0.9965 | 3.11 | 0.53 | 9.2 | 0 |
11.3 | 0.34 | 0.45 | 2.0 | 0.082 | 6.0 | 15.0 | 0.9988 | 2.94 | 0.66 | 9.2 | 1 |
9.4 | 0.615 | 0.28 | 3.2 | 0.087 | 18.0 | 72.0 | 1.0001 | 3.31 | 0.53 | 9.7 | 1 |
6.4 | 0.4 | 0.19 | 3.2 | 0.033 | 28.0 | 124.0 | 0.9904 | 3.22 | 0.54 | 12.7 | 0 |
6.6 | 0.25 | 0.36 | 8.1 | 0.045 | 54.0 | 180.0 | 0.9958 | 3.08 | 0.42 | 9.2 | 0 |
6.7 | 0.24 | 0.31 | 2.3 | 0.044 | 37.0 | 113.0 | 0.99013 | 3.29 | 0.46 | 12.9 | 0 |
6.4 | 0.18 | 0.32 | 9.6 | 0.052 | 24.0 | 90.0 | 0.9963 | 3.35 | 0.49 | 9.4 | 0 |
7.3 | 0.59 | 0.26 | 2.0 | 0.08 | 17.0 | 104.0 | 0.99584 | 3.28 | 0.52 | 9.9 | 1 |
6.6 | 0.16 | 0.25 | 9.8 | 0.049 | 59.5 | 137.0 | 0.995 | 3.16 | 0.38 | 10.0 | 0 |
7.5 | 0.58 | 0.14 | 2.2 | 0.077 | 27.0 | 60.0 | 0.9963 | 3.28 | 0.59 | 9.8 | 1 |
6.7 | 0.105 | 0.32 | 12.4 | 0.051 | 34.0 | 106.0 | 0.998 | 3.54 | 0.45 | 9.2 | 0 |
6.5 | 0.23 | 0.2 | 7.5 | 0.05 | 44.0 | 179.0 | 0.99504 | 3.18 | 0.48 | 9.53333333333333 | 0 |
5.7 | 0.16 | 0.26 | 6.3 | 0.043 | 28.0 | 113.0 | 0.9936 | 3.06 | 0.58 | 9.9 | 0 |
6.2 | 0.23 | 0.36 | 17.2 | 0.039 | 37.0 | 130.0 | 0.99946 | 3.23 | 0.43 | 8.8 | 0 |
7.7 | 0.28 | 0.33 | 6.7 | 0.037 | 32.0 | 155.0 | 0.9951 | 3.39 | 0.62 | 10.7 | 0 |
6.2 | 0.29 | 0.29 | 5.6 | 0.046 | 35.0 | 178.0 | 0.99313 | 3.25 | 0.51 | 10.5333333333333 | 0 |
7.8 | 0.54 | 0.26 | 2.0 | 0.088 | 23.0 | 48.0 | 0.9981 | 3.41 | 0.74 | 9.2 | 1 |
6.8 | 0.26 | 0.32 | 7.0 | 0.041 | 38.0 | 118.0 | 0.9939 | 3.25 | 0.52 | 10.8 | 0 |
5.0 | 0.29 | 0.54 | 5.7 | 0.035 | 54.0 | 155.0 | 0.98976 | 3.27 | 0.34 | 12.9 | 0 |
11.2 | 0.4 | 0.5 | 2.0 | 0.099 | 19.0 | 50.0 | 0.99783 | 3.1 | 0.58 | 10.4 | 1 |
7.4 | 0.3 | 0.22 | 5.25 | 0.053 | 33.0 | 180.0 | 0.9926 | 3.13 | 0.45 | 11.6 | 0 |
6.7 | 0.11 | 0.34 | 8.8 | 0.043 | 41.0 | 113.0 | 0.9962 | 3.42 | 0.4 | 9.3 | 0 |
6.3 | 0.17 | 0.42 | 2.8 | 0.028 | 45.0 | 107.0 | 0.9908 | 3.27 | 0.43 | 11.8 | 0 |
7.2 | 0.34 | 0.21 | 2.5 | 0.075 | 41.0 | 68.0 | 0.99586 | 3.37 | 0.54 | 10.1 | 1 |
7.6 | 0.37 | 0.51 | 11.7 | 0.094 | 58.0 | 181.0 | 0.99776 | 2.91 | 0.51 | 9.0 | 0 |
7.2 | 0.725 | 0.05 | 4.65 | 0.086 | 4.0 | 11.0 | 0.9962 | 3.41 | 0.39 | 10.9 | 1 |
6.4 | 0.14 | 0.31 | 1.2 | 0.034 | 53.0 | 138.0 | 0.99084 | 3.38 | 0.35 | 11.5 | 0 |
11.4 | 0.26 | 0.44 | 3.6 | 0.071 | 6.0 | 19.0 | 0.9986 | 3.12 | 0.82 | 9.3 | 1 |
6.2 | 0.44 | 0.18 | 7.7 | 0.096 | 28.0 | 210.0 | 0.99771 | 3.56 | 0.72 | 9.2 | 0 |
6.8 | 0.28 | 0.36 | 1.6 | 0.04 | 25.0 | 87.0 | 0.9924 | 3.23 | 0.66 | 10.3 | 0 |
6.9 | 0.3 | 0.25 | 3.3 | 0.041 | 26.0 | 124.0 | 0.99428 | 3.18 | 0.5 | 9.3 | 0 |
7.6 | 0.345 | 0.26 | 1.9 | 0.043 | 15.0 | 134.0 | 0.9936 | 3.08 | 0.38 | 9.5 | 0 |
12.4 | 0.42 | 0.49 | 4.6 | 0.073 | 19.0 | 43.0 | 0.9978 | 3.02 | 0.61 | 9.5 | 1 |
11.1 | 0.31 | 0.53 | 2.2 | 0.06 | 3.0 | 10.0 | 0.99572 | 3.02 | 0.83 | 10.9 | 1 |
5.9 | 0.26 | 0.29 | 5.4 | 0.046 | 34.0 | 116.0 | 0.99224 | 3.24 | 0.41 | 11.4 | 0 |
7.5 | 0.24 | 0.62 | 10.6 | 0.045 | 51.0 | 153.0 | 0.99779 | 3.16 | 0.44 | 8.8 | 0 |
8.0 | 0.32 | 0.36 | 4.6 | 0.042 | 56.0 | 178.0 | 0.9928 | 3.29 | 0.47 | 12.0 | 0 |
7.1 | 0.2 | 0.37 | 1.5 | 0.049 | 28.0 | 129.0 | 0.99226 | 3.15 | 0.52 | 10.8 | 0 |
9.4 | 0.42 | 0.32 | 6.5 | 0.027 | 20.0 | 167.0 | 0.99479 | 3.08 | 0.43 | 10.6 | 0 |
5.3 | 0.36 | 0.27 | 6.3 | 0.028 | 40.0 | 132.0 | 0.99186 | 3.37 | 0.4 | 11.6 | 0 |
8.8 | 0.46 | 0.45 | 2.6 | 0.065 | 7.0 | 18.0 | 0.9947 | 3.32 | 0.79 | 14.0 | 1 |
8.1 | 0.29 | 0.49 | 7.1 | 0.042 | 22.0 | 124.0 | 0.9944 | 3.14 | 0.41 | 10.8 | 0 |
7.1 | 0.46 | 0.14 | 2.8 | 0.076 | 15.0 | 37.0 | 0.99624 | 3.36 | 0.49 | 10.7 | 1 |
8.0 | 0.61 | 0.38 | 12.1 | 0.301 | 24.0 | 220.0 | 0.9993 | 2.94 | 0.48 | 9.2 | 0 |
6.2 | 0.15 | 0.49 | 0.9 | 0.033 | 17.0 | 51.0 | 0.9932 | 3.3 | 0.7 | 9.4 | 0 |
6.2 | 0.27 | 0.32 | 8.8 | 0.047 | 65.0 | 224.0 | 0.9961 | 3.17 | 0.47 | 8.9 | 0 |
6.9 | 0.31 | 0.33 | 12.7 | 0.038 | 33.0 | 116.0 | 0.9954 | 3.04 | 0.65 | 10.4 | 0 |
6.8 | 0.28 | 0.4 | 22.0 | 0.048 | 48.0 | 167.0 | 1.001 | 2.93 | 0.5 | 8.7 | 0 |
7.2 | 0.24 | 0.29 | 2.2 | 0.037 | 37.0 | 102.0 | 0.992 | 3.27 | 0.64 | 11.0 | 0 |
6.1 | 0.19 | 0.37 | 2.6 | 0.041 | 24.0 | 99.0 | 0.99153 | 3.18 | 0.5 | 10.9 | 0 |
5.9 | 0.415 | 0.02 | 0.8 | 0.038 | 22.0 | 63.0 | 0.9932 | 3.36 | 0.36 | 9.3 | 0 |
6.3 | 0.26 | 0.25 | 5.2 | 0.046 | 11.0 | 133.0 | 0.99202 | 2.97 | 0.68 | 11.0 | 0 |
7.1 | 0.25 | 0.39 | 2.1 | 0.036 | 30.0 | 124.0 | 0.9908 | 3.28 | 0.43 | 12.2 | 0 |
8.3 | 0.16 | 0.48 | 1.7 | 0.057 | 31.0 | 98.0 | 0.9943 | 3.15 | 0.41 | 10.3 | 0 |
7.6 | 0.41 | 0.14 | 3.0 | 0.087 | 21.0 | 43.0 | 0.9964 | 3.32 | 0.57 | 10.5 | 1 |
7.1 | 0.2 | 0.37 | 1.5 | 0.049 | 28.0 | 129.0 | 0.99226 | 3.15 | 0.52 | 10.8 | 0 |
7.5 | 0.24 | 0.31 | 13.1 | 0.05 | 26.0 | 180.0 | 0.99884 | 3.05 | 0.53 | 9.1 | 0 |
8.4 | 0.56 | 0.04 | 2.0 | 0.082 | 10.0 | 22.0 | 0.9976 | 3.22 | 0.44 | 9.6 | 1 |
6.3 | 0.12 | 0.36 | 2.1 | 0.044 | 47.0 | 146.0 | 0.9914 | 3.27 | 0.74 | 11.4 | 0 |
6.1 | 0.24 | 0.26 | 1.7 | 0.033 | 61.0 | 134.0 | 0.9903 | 3.19 | 0.81 | 11.9 | 0 |
7.6 | 0.29 | 0.29 | 4.4 | 0.051 | 26.0 | 146.0 | 0.9939 | 3.16 | 0.39 | 10.2 | 0 |
6.0 | 0.13 | 0.28 | 5.7 | 0.038 | 56.0 | 189.5 | 0.9948 | 3.59 | 0.43 | 10.6 | 0 |
7.4 | 0.29 | 0.48 | 12.8 | 0.037 | 61.5 | 182.0 | 0.99808 | 3.02 | 0.34 | 8.8 | 0 |
7.5 | 0.71 | 0.0 | 1.6 | 0.092 | 22.0 | 31.0 | 0.99635 | 3.38 | 0.58 | 10.0 | 1 |
8.2 | 0.23 | 0.49 | 0.9 | 0.057 | 15.0 | 73.0 | 0.9928 | 3.07 | 0.38 | 10.4 | 0 |
6.8 | 0.21 | 0.4 | 6.3 | 0.032 | 40.0 | 121.0 | 0.99214 | 3.18 | 0.53 | 12.0 | 0 |
6.4 | 0.28 | 0.29 | 1.6 | 0.052 | 34.0 | 127.0 | 0.9929 | 3.48 | 0.56 | 10.5 | 0 |
6.9 | 0.21 | 0.28 | 2.4 | 0.056 | 49.0 | 159.0 | 0.9944 | 3.02 | 0.47 | 8.8 | 0 |
7.6 | 0.79 | 0.21 | 2.3 | 0.087 | 21.0 | 68.0 | 0.9955 | 3.12 | 0.44 | 9.2 | 1 |
7.5 | 0.42 | 0.14 | 10.7 | 0.046 | 18.0 | 95.0 | 0.9959 | 3.22 | 0.33 | 10.7 | 0 |
10.2 | 0.29 | 0.65 | 2.4 | 0.075 | 6.0 | 17.0 | 0.99565 | 3.22 | 0.63 | 11.8 | 1 |
5.1 | 0.165 | 0.22 | 5.7 | 0.047 | 42.0 | 146.0 | 0.9934 | 3.18 | 0.55 | 9.9 | 0 |
6.4 | 0.17 | 0.34 | 13.4 | 0.044 | 45.0 | 139.0 | 0.99752 | 3.06 | 0.43 | 9.1 | 0 |
8.5 | 0.34 | 0.4 | 4.7 | 0.055 | 3.0 | 9.0 | 0.99738 | 3.38 | 0.66 | 11.6 | 1 |
7.6 | 0.51 | 0.24 | 2.4 | 0.091 | 8.0 | 38.0 | 0.998 | 3.47 | 0.66 | 9.6 | 1 |
7.5 | 0.19 | 0.4 | 7.1 | 0.056 | 50.0 | 110.0 | 0.9954 | 3.06 | 0.52 | 9.9 | 0 |
7.3 | 0.2 | 0.29 | 19.9 | 0.039 | 69.0 | 237.0 | 1.00037 | 3.1 | 0.48 | 9.2 | 0 |
6.6 | 0.36 | 0.52 | 10.1 | 0.05 | 29.0 | 140.0 | 0.99628 | 3.07 | 0.4 | 9.4 | 0 |
8.2 | 0.37 | 0.27 | 1.7 | 0.028 | 10.0 | 59.0 | 0.9923 | 2.97 | 0.48 | 10.4 | 0 |
7.0 | 0.16 | 0.25 | 14.3 | 0.044 | 27.0 | 149.0 | 0.998 | 2.91 | 0.46 | 9.2 | 0 |
7.2 | 0.2 | 0.61 | 16.2 | 0.043 | 14.0 | 103.0 | 0.9987 | 3.06 | 0.36 | 9.2 | 0 |
8.6 | 0.16 | 0.49 | 7.3 | 0.043 | 9.0 | 63.0 | 0.9953 | 3.13 | 0.59 | 10.5 | 0 |
12.7 | 0.6 | 0.49 | 2.8 | 0.075 | 5.0 | 19.0 | 0.9994 | 3.14 | 0.57 | 11.4 | 1 |
6.5 | 0.46 | 0.24 | 11.5 | 0.051 | 56.0 | 171.0 | 0.99588 | 3.08 | 0.56 | 9.8 | 0 |
6.5 | 0.28 | 0.34 | 4.6 | 0.054 | 22.0 | 130.0 | 0.99193 | 3.2 | 0.46 | 12.0 | 0 |
7.0 | 0.43 | 0.02 | 1.9 | 0.08 | 15.0 | 28.0 | 0.99492 | 3.35 | 0.81 | 10.6 | 1 |
7.2 | 0.61 | 0.08 | 4.0 | 0.082 | 26.0 | 108.0 | 0.99641 | 3.25 | 0.51 | 9.4 | 1 |
6.8 | 0.3 | 0.22 | 6.2 | 0.06 | 41.0 | 190.0 | 0.99858 | 3.18 | 0.51 | 9.2 | 0 |
7.4 | 0.19 | 0.42 | 6.4 | 0.067 | 39.0 | 212.0 | 0.9958 | 3.3 | 0.33 | 9.6 | 0 |
6.4 | 0.22 | 0.32 | 7.9 | 0.029 | 34.0 | 124.0 | 0.9948 | 3.4 | 0.39 | 10.2 | 0 |
5.8 | 0.28 | 0.27 | 2.6 | 0.054 | 30.0 | 156.0 | 0.9914 | 3.53 | 0.42 | 12.4 | 0 |
6.1 | 0.38 | 0.42 | 5.0 | 0.016 | 31.0 | 113.0 | 0.99007 | 3.15 | 0.31 | 12.4 | 0 |
6.9 | 0.56 | 0.26 | 10.9 | 0.06 | 55.0 | 193.0 | 0.9969 | 3.21 | 0.44 | 9.4 | 0 |
5.9 | 0.35 | 0.47 | 2.2 | 0.11 | 14.0 | 138.0 | 0.9932 | 3.09 | 0.5 | 9.1 | 0 |
7.3 | 0.31 | 0.69 | 10.2 | 0.041 | 58.0 | 160.0 | 0.9977 | 3.06 | 0.45 | 8.6 | 0 |
6.6 | 0.22 | 0.37 | 1.2 | 0.059 | 45.0 | 199.0 | 0.993 | 3.37 | 0.55 | 10.3 | 0 |
12.0 | 0.37 | 0.76 | 4.2 | 0.066 | 7.0 | 38.0 | 1.0004 | 3.22 | 0.6 | 13.0 | 1 |
6.8 | 0.18 | 0.24 | 9.8 | 0.058 | 64.0 | 188.0 | 0.9952 | 3.13 | 0.51 | 10.6 | 0 |
8.6 | 0.31 | 0.3 | 0.9 | 0.045 | 16.0 | 109.0 | 0.99249 | 2.95 | 0.39 | 10.1 | 0 |
4.8 | 0.13 | 0.32 | 1.2 | 0.042 | 40.0 | 98.0 | 0.9898 | 3.42 | 0.64 | 11.8 | 0 |
7.1 | 0.28 | 0.26 | 1.9 | 0.049 | 12.0 | 86.0 | 0.9934 | 3.15 | 0.38 | 9.4 | 0 |
7.8 | 0.43 | 0.49 | 13.0 | 0.033 | 37.0 | 158.0 | 0.9955 | 3.14 | 0.35 | 11.3 | 0 |
7.8 | 0.64 | 0.0 | 1.9 | 0.072 | 27.0 | 55.0 | 0.9962 | 3.31 | 0.63 | 11.0 | 1 |
7.1 | 0.26 | 0.32 | 5.9 | 0.037 | 39.0 | 97.0 | 0.9934 | 3.31 | 0.4 | 11.6 | 0 |
6.7 | 0.23 | 0.31 | 2.1 | 0.046 | 30.0 | 96.0 | 0.9926 | 3.33 | 0.64 | 10.7 | 0 |
8.2 | 0.24 | 0.34 | 5.1 | 0.062 | 8.0 | 22.0 | 0.9974 | 3.22 | 0.94 | 10.9 | 1 |
12.2 | 0.34 | 0.5 | 2.4 | 0.066 | 10.0 | 21.0 | 1.0 | 3.12 | 1.18 | 9.2 | 1 |
6.9 | 0.18 | 0.38 | 6.5 | 0.039 | 20.0 | 110.0 | 0.9943 | 3.1 | 0.42 | 10.5 | 0 |
5.2 | 0.155 | 0.33 | 1.6 | 0.028 | 13.0 | 59.0 | 0.98975 | 3.3 | 0.84 | 11.9 | 0 |
7.4 | 0.33 | 0.44 | 7.6 | 0.05 | 40.0 | 227.0 | 0.99679 | 3.12 | 0.52 | 9.0 | 0 |
10.3 | 0.27 | 0.24 | 2.1 | 0.072 | 15.0 | 33.0 | 0.9956 | 3.22 | 0.66 | 12.8 | 1 |
5.6 | 0.13 | 0.27 | 4.8 | 0.028 | 22.0 | 104.0 | 0.9948 | 3.34 | 0.45 | 9.2 | 0 |
7.3 | 0.305 | 0.39 | 1.2 | 0.059 | 7.0 | 11.0 | 0.99331 | 3.29 | 0.52 | 11.5 | 1 |
6.0 | 0.2 | 0.26 | 6.8 | 0.049 | 22.0 | 93.0 | 0.9928 | 3.15 | 0.42 | 11.0 | 0 |
5.3 | 0.585 | 0.07 | 7.1 | 0.044 | 34.0 | 145.0 | 0.9945 | 3.34 | 0.57 | 9.7 | 0 |
6.0 | 0.36 | 0.39 | 3.2 | 0.027 | 20.0 | 125.0 | 0.991 | 3.38 | 0.39 | 11.3 | 0 |
6.9 | 0.36 | 0.34 | 4.2 | 0.018 | 57.0 | 119.0 | 0.9898 | 3.28 | 0.36 | 12.7 | 0 |
8.5 | 0.16 | 0.35 | 1.6 | 0.039 | 24.0 | 147.0 | 0.9935 | 2.96 | 0.36 | 10.0 | 0 |
7.4 | 0.66 | 0.0 | 1.8 | 0.075 | 13.0 | 40.0 | 0.9978 | 3.51 | 0.56 | 9.4 | 1 |
6.4 | 0.595 | 0.14 | 5.2 | 0.058 | 15.0 | 97.0 | 0.9951 | 3.38 | 0.36 | 9.0 | 0 |
8.9 | 0.12 | 0.45 | 1.8 | 0.075 | 10.0 | 21.0 | 0.99552 | 3.41 | 0.76 | 11.9 | 1 |
6.9 | 0.25 | 0.35 | 9.2 | 0.034 | 42.0 | 150.0 | 0.9947 | 3.21 | 0.36 | 11.5 | 0 |
6.9 | 0.19 | 0.31 | 19.25 | 0.043 | 38.0 | 167.0 | 0.99954 | 2.93 | 0.52 | 9.1 | 0 |
6.7 | 0.3 | 0.74 | 5.0 | 0.038 | 35.0 | 157.0 | 0.9945 | 3.21 | 0.46 | 9.9 | 0 |
7.4 | 0.2 | 0.29 | 1.7 | 0.047 | 16.0 | 100.0 | 0.99243 | 3.28 | 0.45 | 10.6 | 0 |
7.6 | 0.31 | 0.29 | 10.5 | 0.04 | 21.0 | 145.0 | 0.9966 | 3.04 | 0.35 | 9.4 | 0 |
6.8 | 0.68 | 0.21 | 2.1 | 0.07 | 9.0 | 23.0 | 0.99546 | 3.38 | 0.6 | 10.3 | 1 |
6.4 | 0.21 | 0.28 | 5.9 | 0.047 | 29.0 | 101.0 | 0.99278 | 3.15 | 0.4 | 11.0 | 0 |
9.7 | 0.53 | 0.6 | 2.0 | 0.039 | 5.0 | 19.0 | 0.99585 | 3.3 | 0.86 | 12.4 | 1 |
5.8 | 0.32 | 0.2 | 2.6 | 0.027 | 17.0 | 123.0 | 0.98936 | 3.36 | 0.78 | 13.9 | 0 |
7.4 | 0.63 | 0.07 | 2.4 | 0.09 | 11.0 | 37.0 | 0.9979 | 3.43 | 0.76 | 9.7 | 1 |
7.5 | 0.24 | 0.31 | 13.1 | 0.05 | 26.0 | 180.0 | 0.99884 | 3.05 | 0.53 | 9.1 | 0 |
6.8 | 0.26 | 0.22 | 4.8 | 0.041 | 110.0 | 198.0 | 0.99437 | 3.29 | 0.67 | 10.6 | 0 |
7.3 | 0.815 | 0.09 | 11.4 | 0.044 | 45.0 | 204.0 | 0.99713 | 3.15 | 0.46 | 9.0 | 0 |
6.9 | 0.21 | 0.33 | 1.4 | 0.056 | 35.0 | 136.0 | 0.9938 | 3.63 | 0.78 | 10.3 | 0 |
7.2 | 0.695 | 0.13 | 2.0 | 0.076 | 12.0 | 20.0 | 0.99546 | 3.29 | 0.54 | 10.1 | 1 |
6.9 | 0.4 | 0.17 | 12.9 | 0.033 | 59.0 | 186.0 | 0.99754 | 3.08 | 0.49 | 9.4 | 0 |
7.2 | 0.25 | 0.28 | 14.4 | 0.055 | 55.0 | 205.0 | 0.9986 | 3.12 | 0.38 | 9.0 | 0 |
6.4 | 0.57 | 0.02 | 1.8 | 0.067 | 4.0 | 11.0 | 0.997 | 3.46 | 0.68 | 9.5 | 1 |
10.4 | 0.64 | 0.24 | 2.8 | 0.105 | 29.0 | 53.0 | 0.9998 | 3.24 | 0.67 | 9.9 | 1 |
4.9 | 0.47 | 0.17 | 1.9 | 0.035 | 60.0 | 148.0 | 0.98964 | 3.27 | 0.35 | 11.5 | 0 |
13.4 | 0.27 | 0.62 | 2.6 | 0.082 | 6.0 | 21.0 | 1.0002 | 3.16 | 0.67 | 9.7 | 1 |
7.1 | 0.2 | 0.3 | 0.9 | 0.019 | 4.0 | 28.0 | 0.98931 | 3.2 | 0.36 | 12.0 | 0 |
6.3 | 0.21 | 0.4 | 1.7 | 0.031 | 48.0 | 134.0 | 0.9917 | 3.42 | 0.49 | 11.5 | 0 |
6.6 | 0.32 | 0.47 | 15.6 | 0.063 | 27.0 | 173.0 | 0.99872 | 3.18 | 0.56 | 9.0 | 0 |
6.2 | 0.32 | 0.5 | 6.5 | 0.048 | 61.0 | 186.0 | 0.9948 | 3.19 | 0.45 | 9.6 | 0 |
6.9 | 0.3 | 0.49 | 7.6 | 0.057 | 25.0 | 156.0 | 0.9962 | 3.43 | 0.63 | 11.0 | 0 |
6.7 | 0.2 | 0.42 | 14.0 | 0.038 | 83.0 | 160.0 | 0.9987 | 3.16 | 0.5 | 9.4 | 0 |
6.9 | 0.3 | 0.21 | 15.7 | 0.056 | 49.0 | 159.0 | 0.99827 | 3.11 | 0.48 | 9.0 | 0 |
7.9 | 1.04 | 0.05 | 2.2 | 0.084 | 13.0 | 29.0 | 0.9959 | 3.22 | 0.55 | 9.9 | 1 |
6.5 | 0.27 | 0.19 | 6.6 | 0.045 | 98.0 | 175.0 | 0.99364 | 3.16 | 0.34 | 10.1 | 0 |
6.5 | 0.22 | 0.31 | 3.9 | 0.046 | 17.0 | 106.0 | 0.99098 | 3.15 | 0.31 | 11.5 | 0 |
6.3 | 0.3 | 0.34 | 1.6 | 0.049 | 14.0 | 132.0 | 0.994 | 3.3 | 0.49 | 9.5 | 0 |
6.4 | 0.22 | 0.3 | 11.2 | 0.046 | 53.0 | 149.0 | 0.99479 | 3.21 | 0.34 | 10.8 | 0 |
7.6 | 0.23 | 0.34 | 1.6 | 0.043 | 24.0 | 129.0 | 0.99305 | 3.12 | 0.7 | 10.4 | 0 |
6.8 | 0.3 | 0.33 | 12.8 | 0.041 | 60.0 | 168.0 | 0.99659 | 3.1 | 0.56 | 9.8 | 0 |
7.4 | 0.28 | 0.36 | 1.1 | 0.028 | 42.0 | 105.0 | 0.9893 | 2.99 | 0.39 | 12.4 | 0 |
6.2 | 0.235 | 0.34 | 1.9 | 0.036 | 4.0 | 117.0 | 0.99032 | 3.4 | 0.44 | 12.2 | 0 |
6.2 | 0.28 | 0.45 | 7.5 | 0.045 | 46.0 | 203.0 | 0.99573 | 3.26 | 0.46 | 9.2 | 0 |
6.3 | 0.27 | 0.51 | 7.6 | 0.049 | 35.0 | 200.0 | 0.99548 | 3.16 | 0.54 | 9.4 | 0 |
9.6 | 0.41 | 0.37 | 2.3 | 0.091 | 10.0 | 23.0 | 0.99786 | 3.24 | 0.56 | 10.5 | 1 |
6.5 | 0.32 | 0.23 | 8.5 | 0.051 | 20.0 | 138.0 | 0.9943 | 3.03 | 0.42 | 10.7 | 0 |
7.1 | 0.17 | 0.38 | 7.4 | 0.052 | 49.0 | 182.0 | 0.9958 | 3.35 | 0.52 | 9.6 | 0 |
8.1 | 0.19 | 0.4 | 0.9 | 0.037 | 73.0 | 180.0 | 0.9926 | 3.06 | 0.34 | 10.0 | 0 |
7.3 | 0.25 | 0.26 | 7.2 | 0.048 | 52.0 | 207.0 | 0.99587 | 3.12 | 0.37 | 9.2 | 0 |
9.9 | 0.5 | 0.24 | 2.3 | 0.103 | 6.0 | 14.0 | 0.9978 | 3.34 | 0.52 | 10.0 | 1 |
9.0 | 0.45 | 0.49 | 2.6 | 0.084 | 21.0 | 75.0 | 0.9987 | 3.35 | 0.57 | 9.7 | 1 |
6.6 | 0.27 | 0.25 | 1.2 | 0.033 | 36.0 | 111.0 | 0.98918 | 3.16 | 0.37 | 12.4 | 0 |
6.8 | 0.19 | 0.58 | 14.2 | 0.038 | 51.0 | 164.0 | 0.9975 | 3.12 | 0.48 | 9.6 | 0 |
7.3 | 0.14 | 0.49 | 1.1 | 0.038 | 28.0 | 99.0 | 0.9928 | 3.2 | 0.72 | 10.6 | 0 |
7.3 | 0.24 | 0.34 | 15.4 | 0.05 | 38.0 | 174.0 | 0.9983 | 3.03 | 0.42 | 9.0 | 0 |
7.8 | 0.445 | 0.56 | 1.0 | 0.04 | 8.0 | 84.0 | 0.9938 | 3.25 | 0.43 | 10.8 | 0 |
5.6 | 0.42 | 0.34 | 2.4 | 0.022 | 34.0 | 97.0 | 0.98915 | 3.22 | 0.38 | 12.8 | 0 |
8.7 | 0.42 | 0.45 | 2.4 | 0.072 | 32.0 | 59.0 | 0.99617 | 3.33 | 0.77 | 12.0 | 1 |
6.1 | 0.27 | 0.33 | 2.2 | 0.021 | 26.0 | 117.0 | 0.9886 | 3.12 | 0.3 | 12.5 | 0 |
6.7 | 0.16 | 0.28 | 2.5 | 0.046 | 40.0 | 153.0 | 0.9921 | 3.38 | 0.51 | 11.4 | 0 |
6.0 | 0.31 | 0.27 | 2.3 | 0.042 | 19.0 | 120.0 | 0.98952 | 3.32 | 0.41 | 12.7 | 0 |
6.4 | 0.32 | 0.5 | 10.7 | 0.047 | 57.0 | 206.0 | 0.9968 | 3.08 | 0.6 | 9.4 | 0 |
8.0 | 0.18 | 0.37 | 0.9 | 0.049 | 36.0 | 109.0 | 0.99007 | 2.89 | 0.44 | 12.7 | 1 |
7.5 | 0.28 | 0.33 | 7.7 | 0.048 | 42.0 | 180.0 | 0.9974 | 3.37 | 0.59 | 10.1 | 0 |
6.5 | 0.21 | 0.4 | 7.3 | 0.041 | 49.0 | 115.0 | 0.99268 | 3.21 | 0.43 | 11.0 | 0 |
6.7 | 0.28 | 0.42 | 3.5 | 0.035 | 43.0 | 105.0 | 0.99021 | 3.18 | 0.38 | 12.2 | 0 |
8.2 | 0.56 | 0.23 | 3.4 | 0.078 | 14.0 | 104.0 | 0.9976 | 3.28 | 0.62 | 9.4 | 1 |
7.0 | 0.16 | 0.3 | 2.6 | 0.043 | 34.0 | 90.0 | 0.99047 | 2.88 | 0.47 | 11.2 | 0 |
6.4 | 0.23 | 0.26 | 8.1 | 0.054 | 47.0 | 181.0 | 0.9954 | 3.12 | 0.49 | 9.4 | 0 |
6.3 | 0.28 | 0.34 | 8.1 | 0.038 | 44.0 | 129.0 | 0.99248 | 3.26 | 0.29 | 12.1 | 0 |
5.9 | 0.18 | 0.28 | 1.0 | 0.037 | 24.0 | 88.0 | 0.99094 | 3.29 | 0.55 | 10.65 | 0 |
6.8 | 0.21 | 0.27 | 18.15 | 0.042 | 41.0 | 146.0 | 1.0001 | 3.3 | 0.36 | 8.7 | 0 |
6.7 | 0.24 | 0.41 | 2.9 | 0.039 | 48.0 | 122.0 | 0.99052 | 3.25 | 0.43 | 12.0 | 0 |
6.3 | 0.13 | 0.42 | 1.1 | 0.043 | 63.0 | 146.0 | 0.99066 | 3.13 | 0.72 | 11.2 | 0 |
6.8 | 0.18 | 0.35 | 5.4 | 0.054 | 53.0 | 143.0 | 0.99287 | 3.1 | 0.54 | 11.0 | 0 |
7.3 | 0.21 | 0.3 | 10.9 | 0.037 | 18.0 | 112.0 | 0.997 | 3.4 | 0.5 | 9.6 | 0 |
6.5 | 0.24 | 0.39 | 17.3 | 0.052 | 22.0 | 126.0 | 0.99888 | 3.11 | 0.47 | 9.2 | 0 |
6.8 | 0.23 | 0.32 | 8.6 | 0.046 | 47.0 | 159.0 | 0.99452 | 3.08 | 0.52 | 10.5 | 0 |
6.8 | 0.34 | 0.69 | 1.3 | 0.058 | 12.0 | 171.0 | 0.9931 | 3.06 | 0.47 | 9.7 | 0 |
6.5 | 0.27 | 0.4 | 10.0 | 0.039 | 74.0 | 227.0 | 0.99582 | 3.18 | 0.5 | 9.4 | 0 |
8.0 | 0.24 | 0.26 | 1.7 | 0.033 | 36.0 | 136.0 | 0.99316 | 3.44 | 0.51 | 10.4 | 0 |
6.8 | 0.15 | 0.32 | 8.8 | 0.058 | 24.0 | 110.0 | 0.9972 | 3.4 | 0.4 | 8.8 | 0 |
6.0 | 0.11 | 0.47 | 10.6 | 0.052 | 69.0 | 148.0 | 0.9958 | 2.91 | 0.34 | 9.3 | 0 |
7.2 | 0.17 | 0.41 | 1.6 | 0.052 | 24.0 | 126.0 | 0.99228 | 3.19 | 0.49 | 10.8 | 0 |
6.9 | 0.2 | 0.3 | 4.7 | 0.041 | 40.0 | 148.0 | 0.9932 | 3.16 | 0.35 | 10.2 | 0 |
5.9 | 0.17 | 0.28 | 0.7 | 0.027 | 5.0 | 28.0 | 0.98985 | 3.13 | 0.32 | 10.6 | 0 |
8.3 | 0.18 | 0.3 | 1.1 | 0.033 | 20.0 | 57.0 | 0.99109 | 3.02 | 0.51 | 11.0 | 0 |
7.2 | 0.57 | 0.06 | 1.6 | 0.076 | 9.0 | 27.0 | 0.9972 | 3.36 | 0.7 | 9.6 | 1 |
6.6 | 0.25 | 0.51 | 8.0 | 0.047 | 61.0 | 189.0 | 0.99604 | 3.22 | 0.49 | 9.2 | 0 |
7.3 | 0.4 | 0.24 | 6.7 | 0.058 | 41.0 | 166.0 | 0.995 | 3.2 | 0.41 | 9.9 | 0 |
6.8 | 0.28 | 0.29 | 11.9 | 0.052 | 51.0 | 149.0 | 0.99544 | 3.02 | 0.58 | 10.4 | 0 |
6.8 | 0.33 | 0.28 | 1.2 | 0.032 | 38.0 | 131.0 | 0.9889 | 3.19 | 0.41 | 13.0 | 0 |
9.4 | 0.27 | 0.53 | 2.4 | 0.074 | 6.0 | 18.0 | 0.9962 | 3.2 | 1.13 | 12.0 | 1 |
7.7 | 0.28 | 0.24 | 2.4 | 0.044 | 29.0 | 157.0 | 0.99312 | 3.27 | 0.56 | 10.6 | 0 |
6.6 | 0.33 | 0.24 | 16.05 | 0.045 | 31.0 | 147.0 | 0.99822 | 3.08 | 0.52 | 9.2 | 0 |
7.1 | 0.59 | 0.01 | 2.5 | 0.077 | 20.0 | 85.0 | 0.99746 | 3.55 | 0.59 | 9.8 | 1 |
6.6 | 0.28 | 0.3 | 7.8 | 0.049 | 57.0 | 202.0 | 0.9958 | 3.24 | 0.39 | 9.5 | 0 |
10.8 | 0.45 | 0.33 | 2.5 | 0.099 | 20.0 | 38.0 | 0.99818 | 3.24 | 0.71 | 10.8 | 1 |
7.8 | 0.32 | 0.33 | 10.4 | 0.031 | 47.0 | 194.0 | 0.99692 | 3.07 | 0.58 | 9.6 | 0 |
6.7 | 0.36 | 0.28 | 8.3 | 0.034 | 29.0 | 81.0 | 0.99151 | 2.96 | 0.39 | 12.5 | 0 |
6.7 | 0.26 | 0.26 | 4.0 | 0.079 | 35.5 | 216.0 | 0.9956 | 3.31 | 0.68 | 9.5 | 0 |
6.9 | 0.28 | 0.33 | 1.2 | 0.039 | 16.0 | 98.0 | 0.9904 | 3.07 | 0.39 | 11.7 | 0 |
6.1 | 0.35 | 0.07 | 1.4 | 0.069 | 22.0 | 108.0 | 0.9934 | 3.23 | 0.52 | 9.2 | 0 |
5.9 | 0.24 | 0.12 | 1.4 | 0.035 | 60.0 | 247.0 | 0.99358 | 3.34 | 0.44 | 9.6 | 0 |
7.5 | 0.28 | 0.34 | 4.2 | 0.028 | 36.0 | 116.0 | 0.991 | 2.99 | 0.41 | 12.3 | 0 |
6.1 | 0.37 | 0.36 | 4.7 | 0.035 | 36.0 | 116.0 | 0.991 | 3.31 | 0.62 | 12.6 | 0 |
7.1 | 0.875 | 0.05 | 5.7 | 0.082 | 3.0 | 14.0 | 0.99808 | 3.4 | 0.52 | 10.2 | 1 |
7.8 | 0.53 | 0.33 | 2.4 | 0.08 | 24.0 | 144.0 | 0.99655 | 3.3 | 0.6 | 9.5 | 1 |
7.6 | 0.32 | 0.25 | 9.5 | 0.03 | 15.0 | 136.0 | 0.99367 | 3.1 | 0.44 | 12.1 | 0 |
6.4 | 0.24 | 0.49 | 5.8 | 0.053 | 25.0 | 120.0 | 0.9942 | 3.01 | 0.98 | 10.5 | 0 |
8.1 | 0.3 | 0.31 | 1.1 | 0.041 | 49.0 | 123.0 | 0.9914 | 2.99 | 0.45 | 11.1 | 0 |
6.7 | 0.36 | 0.26 | 7.9 | 0.034 | 39.0 | 123.0 | 0.99119 | 2.99 | 0.3 | 12.2 | 0 |
6.6 | 0.36 | 0.52 | 11.3 | 0.046 | 8.0 | 110.0 | 0.9966 | 3.07 | 0.46 | 9.4 | 0 |
6.3 | 0.27 | 0.46 | 11.75 | 0.037 | 61.0 | 212.0 | 0.9971 | 3.25 | 0.53 | 9.5 | 0 |
8.8 | 0.48 | 0.41 | 3.3 | 0.092 | 26.0 | 52.0 | 0.9982 | 3.31 | 0.53 | 10.5 | 1 |
9.2 | 0.22 | 0.4 | 2.4 | 0.054 | 18.0 | 151.0 | 0.9952 | 3.04 | 0.46 | 9.3 | 0 |
6.7 | 0.45 | 0.3 | 5.3 | 0.036 | 27.0 | 165.0 | 0.99122 | 3.12 | 0.46 | 12.2 | 0 |
8.2 | 0.28 | 0.4 | 2.4 | 0.052 | 4.0 | 10.0 | 0.99356 | 3.33 | 0.7 | 12.8 | 1 |
5.9 | 0.29 | 0.28 | 3.2 | 0.035 | 16.0 | 117.0 | 0.98959 | 3.26 | 0.42 | 12.6 | 0 |
7.2 | 0.17 | 0.34 | 6.4 | 0.042 | 16.0 | 111.0 | 0.99278 | 2.99 | 0.4 | 10.8 | 0 |
7.1 | 0.36 | 0.56 | 1.3 | 0.046 | 25.0 | 102.0 | 0.9923 | 3.24 | 0.33 | 10.5 | 0 |
9.2 | 0.19 | 0.42 | 2.0 | 0.047 | 16.0 | 104.0 | 0.99517 | 3.09 | 0.66 | 10.0 | 0 |
7.8 | 0.2 | 0.24 | 1.6 | 0.026 | 26.0 | 189.0 | 0.991 | 3.08 | 0.74 | 12.1 | 0 |
8.2 | 0.42 | 0.49 | 2.6 | 0.084 | 32.0 | 55.0 | 0.9988 | 3.34 | 0.75 | 8.7 | 1 |
9.9 | 0.54 | 0.45 | 2.3 | 0.071 | 16.0 | 40.0 | 0.9991 | 3.39 | 0.62 | 9.4 | 1 |
7.6 | 0.665 | 0.1 | 1.5 | 0.066 | 27.0 | 55.0 | 0.99655 | 3.39 | 0.51 | 9.3 | 1 |
6.8 | 0.11 | 0.42 | 1.1 | 0.042 | 51.0 | 132.0 | 0.99059 | 3.18 | 0.74 | 11.3 | 0 |
8.3 | 0.6 | 0.13 | 2.6 | 0.085 | 6.0 | 24.0 | 0.9984 | 3.31 | 0.59 | 9.2 | 1 |
6.4 | 0.16 | 0.28 | 2.2 | 0.042 | 33.0 | 93.0 | 0.9914 | 3.31 | 0.43 | 11.1 | 0 |
8.5 | 0.46 | 0.31 | 2.25 | 0.078 | 32.0 | 58.0 | 0.998 | 3.33 | 0.54 | 9.8 | 1 |
6.2 | 0.16 | 0.32 | 1.1 | 0.036 | 74.0 | 184.0 | 0.99096 | 3.22 | 0.41 | 11.0 | 0 |
8.2 | 0.27 | 0.43 | 1.6 | 0.035 | 31.0 | 128.0 | 0.9916 | 3.1 | 0.5 | 12.3 | 0 |
6.9 | 0.32 | 0.17 | 7.6 | 0.042 | 69.0 | 219.0 | 0.9959 | 3.13 | 0.4 | 8.9 | 0 |
10.4 | 0.44 | 0.42 | 1.5 | 0.145 | 34.0 | 48.0 | 0.99832 | 3.38 | 0.86 | 9.9 | 1 |
6.5 | 0.19 | 0.3 | 0.8 | 0.043 | 33.0 | 144.0 | 0.9936 | 3.42 | 0.39 | 9.1 | 0 |
6.3 | 0.26 | 0.42 | 7.1 | 0.045 | 62.0 | 209.0 | 0.99544 | 3.2 | 0.53 | 9.5 | 0 |
6.9 | 0.63 | 0.01 | 2.4 | 0.076 | 14.0 | 39.0 | 0.99522 | 3.34 | 0.53 | 10.8 | 1 |
6.1 | 0.25 | 0.18 | 10.5 | 0.049 | 41.0 | 124.0 | 0.9963 | 3.14 | 0.35 | 10.5 | 0 |
6.3 | 0.34 | 0.19 | 5.8 | 0.041 | 22.0 | 145.0 | 0.9943 | 3.15 | 0.63 | 9.9 | 0 |
6.3 | 0.22 | 0.43 | 4.55 | 0.038 | 31.0 | 130.0 | 0.9918 | 3.35 | 0.33 | 11.5 | 0 |
7.4 | 0.24 | 0.36 | 2.0 | 0.031 | 27.0 | 139.0 | 0.99055 | 3.28 | 0.48 | 12.5 | 0 |
6.0 | 0.33 | 0.2 | 1.8 | 0.031 | 49.0 | 159.0 | 0.9919 | 3.41 | 0.53 | 11.0 | 0 |
6.7 | 0.18 | 0.37 | 1.3 | 0.027 | 42.0 | 125.0 | 0.98939 | 3.24 | 0.37 | 12.8 | 0 |
9.2 | 0.34 | 0.54 | 17.3 | 0.06 | 46.0 | 235.0 | 1.00182 | 3.08 | 0.61 | 8.8 | 0 |
6.2 | 0.43 | 0.49 | 6.4 | 0.045 | 12.0 | 115.0 | 0.9963 | 3.27 | 0.57 | 9.0 | 0 |
7.0 | 0.975 | 0.04 | 2.0 | 0.087 | 12.0 | 67.0 | 0.99565 | 3.35 | 0.6 | 9.4 | 1 |
6.6 | 0.22 | 0.23 | 17.3 | 0.047 | 37.0 | 118.0 | 0.99906 | 3.08 | 0.46 | 8.8 | 0 |
6.8 | 0.21 | 0.62 | 6.4 | 0.041 | 7.0 | 113.0 | 0.99358 | 2.96 | 0.59 | 10.2 | 0 |
6.9 | 0.49 | 0.1 | 2.3 | 0.074 | 12.0 | 30.0 | 0.9959 | 3.42 | 0.58 | 10.2 | 1 |
6.4 | 0.31 | 0.53 | 8.8 | 0.057 | 36.0 | 221.0 | 0.99642 | 3.17 | 0.44 | 9.1 | 0 |
6.6 | 0.29 | 0.44 | 9.0 | 0.053 | 62.0 | 178.0 | 0.99685 | 3.02 | 0.45 | 8.9 | 0 |
7.5 | 0.42 | 0.45 | 9.1 | 0.029 | 20.0 | 125.0 | 0.996 | 3.12 | 0.36 | 10.1 | 0 |
7.0 | 0.34 | 0.39 | 6.9 | 0.066 | 43.0 | 162.0 | 0.99561 | 3.11 | 0.53 | 9.5 | 0 |
6.9 | 0.25 | 0.34 | 1.3 | 0.035 | 27.0 | 82.0 | 0.99045 | 3.18 | 0.44 | 12.2 | 0 |
9.3 | 0.37 | 0.44 | 1.6 | 0.038 | 21.0 | 42.0 | 0.99526 | 3.24 | 0.81 | 10.8 | 1 |
7.9 | 0.29 | 0.39 | 6.7 | 0.036 | 6.0 | 117.0 | 0.9938 | 3.12 | 0.42 | 10.7 | 0 |
6.6 | 0.56 | 0.15 | 10.0 | 0.037 | 38.0 | 157.0 | 0.99642 | 3.28 | 0.52 | 9.4 | 0 |
6.6 | 0.58 | 0.02 | 2.4 | 0.069 | 19.0 | 40.0 | 0.99387 | 3.38 | 0.66 | 12.6 | 1 |
6.9 | 0.38 | 0.29 | 13.65 | 0.048 | 52.0 | 189.0 | 0.99784 | 3.0 | 0.6 | 9.5 | 0 |
7.1 | 0.13 | 0.38 | 1.8 | 0.046 | 14.0 | 114.0 | 0.9925 | 3.32 | 0.9 | 11.7 | 0 |
5.8 | 0.315 | 0.27 | 1.55 | 0.026 | 15.0 | 70.0 | 0.98994 | 3.37 | 0.4 | 11.9 | 0 |
6.8 | 0.44 | 0.37 | 5.1 | 0.047 | 46.0 | 201.0 | 0.9938 | 3.08 | 0.65 | 10.5 | 0 |
6.1 | 1.1 | 0.16 | 4.4 | 0.033 | 8.0 | 109.0 | 0.99058 | 3.35 | 0.47 | 12.4 | 0 |
6.7 | 0.29 | 0.45 | 14.3 | 0.054 | 30.0 | 181.0 | 0.99869 | 3.14 | 0.57 | 9.1 | 0 |
7.0 | 0.54 | 0.0 | 2.1 | 0.079 | 39.0 | 55.0 | 0.9956 | 3.39 | 0.84 | 11.4 | 1 |
7.3 | 0.26 | 0.36 | 5.2 | 0.04 | 31.0 | 141.0 | 0.9931 | 3.16 | 0.59 | 11.0 | 0 |
6.2 | 0.43 | 0.22 | 1.8 | 0.078 | 21.0 | 56.0 | 0.99633 | 3.52 | 0.6 | 9.5 | 1 |
7.3 | 0.19 | 0.24 | 6.3 | 0.054 | 34.0 | 231.0 | 0.9964 | 3.36 | 0.54 | 10.0 | 0 |
6.7 | 0.46 | 0.24 | 1.7 | 0.077 | 18.0 | 34.0 | 0.9948 | 3.39 | 0.6 | 10.6 | 1 |
6.0 | 0.28 | 0.29 | 19.3 | 0.051 | 36.0 | 174.0 | 0.99911 | 3.14 | 0.5 | 9.0 | 0 |
5.5 | 0.16 | 0.31 | 1.2 | 0.026 | 31.0 | 68.0 | 0.9898 | 3.33 | 0.44 | 11.65 | 0 |
6.0 | 0.34 | 0.32 | 3.8 | 0.044 | 13.0 | 116.0 | 0.99108 | 3.39 | 0.44 | 11.8 | 0 |
6.2 | 0.36 | 0.24 | 2.2 | 0.095 | 19.0 | 42.0 | 0.9946 | 3.57 | 0.57 | 11.7 | 1 |
6.8 | 0.48 | 0.08 | 1.8 | 0.074 | 40.0 | 64.0 | 0.99529 | 3.12 | 0.49 | 9.6 | 1 |
6.8 | 0.17 | 0.34 | 2.0 | 0.04 | 38.0 | 111.0 | 0.99 | 3.24 | 0.45 | 12.9 | 0 |
6.5 | 0.22 | 0.19 | 4.5 | 0.096 | 16.0 | 115.0 | 0.9937 | 3.02 | 0.44 | 9.6 | 0 |
8.1 | 0.2 | 0.3 | 1.3 | 0.036 | 7.0 | 49.0 | 0.99242 | 2.99 | 0.73 | 10.3 | 0 |
6.5 | 0.22 | 0.19 | 1.1 | 0.064 | 36.0 | 191.0 | 0.99297 | 3.05 | 0.5 | 9.5 | 0 |
6.9 | 0.22 | 0.32 | 5.8 | 0.041 | 20.0 | 119.0 | 0.99296 | 3.17 | 0.55 | 11.2 | 0 |
6.5 | 0.23 | 0.45 | 2.1 | 0.027 | 43.0 | 104.0 | 0.99054 | 3.02 | 0.52 | 11.3 | 0 |
7.6 | 0.19 | 0.41 | 1.1 | 0.04 | 38.0 | 143.0 | 0.9907 | 2.92 | 0.42 | 11.4 | 0 |
6.0 | 0.24 | 0.28 | 3.95 | 0.038 | 61.0 | 134.0 | 0.99146 | 3.3 | 0.54 | 11.3 | 0 |
8.6 | 0.685 | 0.1 | 1.6 | 0.092 | 3.0 | 12.0 | 0.99745 | 3.31 | 0.65 | 9.55 | 1 |
7.1 | 0.755 | 0.15 | 1.8 | 0.107 | 20.0 | 84.0 | 0.99593 | 3.19 | 0.5 | 9.5 | 1 |
6.7 | 0.22 | 0.39 | 10.2 | 0.038 | 60.0 | 149.0 | 0.99725 | 3.17 | 0.54 | 10.0 | 0 |
9.2 | 0.43 | 0.49 | 2.4 | 0.086 | 23.0 | 116.0 | 0.9976 | 3.23 | 0.64 | 9.5 | 1 |
6.1 | 0.27 | 0.25 | 1.8 | 0.041 | 9.0 | 109.0 | 0.9929 | 3.08 | 0.54 | 9.0 | 0 |
6.4 | 0.31 | 0.28 | 2.5 | 0.039 | 34.0 | 137.0 | 0.98946 | 3.22 | 0.38 | 12.7 | 0 |
6.0 | 0.33 | 0.38 | 9.7 | 0.04 | 29.0 | 124.0 | 0.9954 | 3.47 | 0.48 | 11.0 | 0 |
7.4 | 0.26 | 0.31 | 7.6 | 0.047 | 52.0 | 177.0 | 0.9962 | 3.13 | 0.45 | 8.9 | 0 |
7.6 | 0.31 | 0.26 | 1.7 | 0.073 | 40.0 | 157.0 | 0.9938 | 3.1 | 0.46 | 9.8 | 0 |
6.0 | 0.28 | 0.22 | 12.15 | 0.048 | 42.0 | 163.0 | 0.9957 | 3.2 | 0.46 | 10.1 | 0 |
7.4 | 0.26 | 0.29 | 3.7 | 0.048 | 14.0 | 73.0 | 0.9915 | 3.06 | 0.45 | 11.4 | 0 |
8.0 | 0.81 | 0.25 | 3.4 | 0.076 | 34.0 | 85.0 | 0.99668 | 3.19 | 0.42 | 9.2 | 1 |
6.3 | 0.48 | 0.04 | 1.1 | 0.046 | 30.0 | 99.0 | 0.9928 | 3.24 | 0.36 | 9.6 | 0 |
6.8 | 0.27 | 0.28 | 13.3 | 0.076 | 50.0 | 163.0 | 0.9979 | 3.03 | 0.38 | 8.6 | 0 |
6.9 | 0.2 | 0.5 | 10.0 | 0.036 | 78.0 | 167.0 | 0.9964 | 3.15 | 0.55 | 10.2 | 0 |
7.4 | 0.34 | 0.28 | 12.1 | 0.049 | 31.0 | 149.0 | 0.99677 | 3.22 | 0.49 | 10.3 | 0 |
8.7 | 0.63 | 0.28 | 2.7 | 0.096 | 17.0 | 69.0 | 0.99734 | 3.26 | 0.63 | 10.2 | 1 |
6.2 | 0.28 | 0.28 | 4.3 | 0.026 | 22.0 | 105.0 | 0.989 | 2.98 | 0.64 | 13.1 | 0 |
6.7 | 0.24 | 0.33 | 12.3 | 0.046 | 31.0 | 145.0 | 0.9983 | 3.36 | 0.4 | 9.5 | 0 |
6.7 | 0.19 | 0.23 | 6.2 | 0.047 | 36.0 | 117.0 | 0.9945 | 3.34 | 0.43 | 9.6 | 0 |
6.2 | 0.26 | 0.32 | 15.3 | 0.031 | 64.0 | 185.0 | 0.99835 | 3.31 | 0.61 | 9.4 | 0 |
8.3 | 0.6 | 0.25 | 2.2 | 0.118 | 9.0 | 38.0 | 0.99616 | 3.15 | 0.53 | 9.8 | 1 |
6.4 | 0.42 | 0.19 | 9.3 | 0.043 | 28.0 | 145.0 | 0.99433 | 3.23 | 0.53 | 10.98 | 0 |
8.4 | 0.665 | 0.61 | 2.0 | 0.112 | 13.0 | 95.0 | 0.997 | 3.16 | 0.54 | 9.1 | 1 |
6.7 | 0.34 | 0.3 | 15.6 | 0.054 | 51.0 | 196.0 | 0.9982 | 3.19 | 0.49 | 9.3 | 0 |
7.4 | 0.23 | 0.25 | 1.4 | 0.049 | 43.0 | 141.0 | 0.9934 | 3.42 | 0.54 | 10.2 | 0 |
6.2 | 0.3 | 0.32 | 1.2 | 0.052 | 32.0 | 185.0 | 0.99266 | 3.28 | 0.44 | 10.1 | 0 |
6.9 | 0.33 | 0.26 | 5.0 | 0.027 | 46.0 | 143.0 | 0.9924 | 3.25 | 0.43 | 11.2 | 0 |
7.4 | 0.37 | 0.26 | 9.6 | 0.05 | 33.0 | 134.0 | 0.99608 | 3.13 | 0.46 | 10.4 | 0 |
9.3 | 0.36 | 0.39 | 1.5 | 0.08 | 41.0 | 55.0 | 0.99652 | 3.47 | 0.73 | 10.9 | 1 |
6.9 | 0.54 | 0.04 | 3.0 | 0.077 | 7.0 | 27.0 | 0.9987 | 3.69 | 0.91 | 9.4 | 1 |
6.2 | 0.21 | 0.27 | 1.7 | 0.038 | 41.0 | 150.0 | 0.9933 | 3.49 | 0.71 | 10.5 | 0 |
5.8 | 0.6 | 0.0 | 1.3 | 0.044 | 72.0 | 197.0 | 0.99202 | 3.56 | 0.43 | 10.9 | 0 |
6.5 | 0.51 | 0.25 | 1.7 | 0.048 | 39.0 | 177.0 | 0.99212 | 3.28 | 0.57 | 10.6 | 0 |
6.8 | 0.36 | 0.32 | 1.6 | 0.039 | 10.0 | 124.0 | 0.9948 | 3.3 | 0.67 | 9.6 | 0 |
13.2 | 0.46 | 0.52 | 2.2 | 0.071 | 12.0 | 35.0 | 1.0006 | 3.1 | 0.56 | 9.0 | 1 |
7.7 | 0.18 | 0.3 | 1.2 | 0.046 | 49.0 | 199.0 | 0.99413 | 3.03 | 0.38 | 9.3 | 0 |
6.3 | 0.695 | 0.55 | 12.9 | 0.056 | 58.0 | 252.0 | 0.99806 | 3.29 | 0.49 | 8.7 | 0 |
7.9 | 0.35 | 0.24 | 15.6 | 0.072 | 44.0 | 229.0 | 0.99785 | 3.03 | 0.59 | 10.5 | 0 |
6.8 | 0.12 | 0.3 | 12.9 | 0.049 | 32.0 | 88.0 | 0.99654 | 3.2 | 0.35 | 9.9 | 0 |
7.3 | 0.19 | 0.49 | 15.55 | 0.058 | 50.0 | 134.0 | 0.9998 | 3.42 | 0.36 | 9.1 | 0 |
6.7 | 0.47 | 0.34 | 8.9 | 0.043 | 31.0 | 172.0 | 0.9964 | 3.22 | 0.6 | 9.2 | 0 |
8.3 | 0.3 | 0.49 | 3.8 | 0.09 | 11.0 | 24.0 | 0.99498 | 3.27 | 0.64 | 12.1 | 1 |
7.1 | 0.16 | 0.25 | 1.3 | 0.034 | 28.0 | 123.0 | 0.9915 | 3.27 | 0.55 | 11.4 | 0 |
7.0 | 0.42 | 0.19 | 2.3 | 0.071 | 18.0 | 36.0 | 0.99476 | 3.39 | 0.56 | 10.9 | 1 |
8.4 | 0.35 | 0.71 | 12.2 | 0.046 | 22.0 | 160.0 | 0.9982 | 2.98 | 0.65 | 9.4 | 0 |
6.4 | 0.24 | 0.49 | 5.8 | 0.053 | 25.0 | 120.0 | 0.9942 | 3.01 | 0.98 | 10.5 | 0 |
8.0 | 0.28 | 0.44 | 1.8 | 0.081 | 28.0 | 68.0 | 0.99501 | 3.36 | 0.66 | 11.2 | 1 |
5.0 | 0.35 | 0.25 | 7.8 | 0.031 | 24.0 | 116.0 | 0.99241 | 3.39 | 0.4 | 11.3 | 0 |
5.8 | 0.36 | 0.26 | 3.3 | 0.038 | 40.0 | 153.0 | 0.9911 | 3.34 | 0.55 | 11.3 | 0 |
11.9 | 0.695 | 0.53 | 3.4 | 0.128 | 7.0 | 21.0 | 0.9992 | 3.17 | 0.84 | 12.2 | 1 |
7.3 | 0.33 | 0.22 | 1.4 | 0.041 | 40.0 | 177.0 | 0.99287 | 3.14 | 0.48 | 9.9 | 0 |
6.5 | 0.26 | 0.28 | 12.5 | 0.046 | 80.0 | 225.0 | 0.99685 | 3.18 | 0.41 | 10.0 | 0 |
7.0 | 0.31 | 0.29 | 1.4 | 0.037 | 33.0 | 128.0 | 0.9896 | 3.12 | 0.36 | 12.2 | 0 |
7.0 | 0.12 | 0.29 | 10.3 | 0.039 | 41.0 | 98.0 | 0.99564 | 3.19 | 0.38 | 9.8 | 0 |
6.8 | 0.36 | 0.32 | 1.8 | 0.067 | 4.0 | 8.0 | 0.9928 | 3.36 | 0.55 | 12.8 | 1 |
7.0 | 0.56 | 0.17 | 1.7 | 0.065 | 15.0 | 24.0 | 0.99514 | 3.44 | 0.68 | 10.55 | 1 |
10.9 | 0.39 | 0.47 | 1.8 | 0.118 | 6.0 | 14.0 | 0.9982 | 3.3 | 0.75 | 9.8 | 1 |
7.0 | 0.31 | 0.35 | 1.6 | 0.063 | 13.0 | 119.0 | 0.99184 | 3.22 | 0.5 | 10.7 | 0 |
6.4 | 0.31 | 0.39 | 7.5 | 0.04 | 57.0 | 213.0 | 0.99475 | 3.32 | 0.43 | 10.0 | 0 |
6.0 | 0.13 | 0.36 | 1.6 | 0.052 | 23.0 | 72.0 | 0.98974 | 3.1 | 0.5 | 11.5 | 0 |
7.3 | 0.205 | 0.31 | 1.7 | 0.06 | 34.0 | 110.0 | 0.9963 | 3.72 | 0.69 | 10.5 | 0 |
10.6 | 0.34 | 0.49 | 3.2 | 0.078 | 20.0 | 78.0 | 0.9992 | 3.19 | 0.7 | 10.0 | 1 |
6.8 | 0.64 | 0.1 | 2.1 | 0.085 | 18.0 | 101.0 | 0.9956 | 3.34 | 0.52 | 10.2 | 1 |
8.6 | 0.37 | 0.7 | 12.15 | 0.039 | 21.0 | 158.0 | 0.9983 | 3.0 | 0.73 | 9.3 | 0 |
7.1 | 0.62 | 0.06 | 1.3 | 0.07 | 5.0 | 12.0 | 0.9942 | 3.17 | 0.48 | 9.8 | 1 |
6.9 | 0.29 | 0.3 | 8.2 | 0.026 | 35.0 | 112.0 | 0.99144 | 3.0 | 0.37 | 12.3 | 0 |
7.4 | 0.22 | 0.28 | 9.0 | 0.046 | 22.0 | 121.0 | 0.99468 | 3.1 | 0.55 | 10.8 | 0 |
6.9 | 0.37 | 0.23 | 9.5 | 0.057 | 54.0 | 166.0 | 0.99568 | 3.23 | 0.42 | 10.0 | 0 |
6.0 | 0.26 | 0.18 | 7.0 | 0.055 | 50.0 | 194.0 | 0.99591 | 3.21 | 0.43 | 9.0 | 0 |
5.2 | 0.34 | 0.0 | 1.8 | 0.05 | 27.0 | 63.0 | 0.9916 | 3.68 | 0.79 | 14.0 | 1 |
7.2 | 0.21 | 0.29 | 3.1 | 0.044 | 39.0 | 122.0 | 0.99143 | 3.0 | 0.6 | 11.3 | 0 |
5.6 | 0.225 | 0.24 | 9.8 | 0.054 | 59.0 | 140.0 | 0.99545 | 3.17 | 0.39 | 10.2 | 0 |
7.4 | 0.49 | 0.27 | 2.1 | 0.071 | 14.0 | 25.0 | 0.99388 | 3.35 | 0.63 | 12.0 | 1 |
7.6 | 0.17 | 0.46 | 0.9 | 0.036 | 63.0 | 147.0 | 0.99126 | 3.02 | 0.41 | 10.7 | 0 |
6.7 | 0.66 | 0.0 | 13.0 | 0.033 | 32.0 | 75.0 | 0.99551 | 3.15 | 0.5 | 10.7 | 0 |
7.4 | 0.36 | 0.32 | 1.9 | 0.036 | 27.0 | 119.0 | 0.99196 | 3.15 | 0.49 | 11.2 | 0 |
7.9 | 0.69 | 0.21 | 2.1 | 0.08 | 33.0 | 141.0 | 0.9962 | 3.25 | 0.51 | 9.9 | 1 |
7.4 | 0.18 | 0.27 | 1.3 | 0.048 | 26.0 | 105.0 | 0.994 | 3.52 | 0.66 | 10.6 | 0 |
7.3 | 0.22 | 0.31 | 2.3 | 0.018 | 45.0 | 80.0 | 0.98936 | 3.06 | 0.34 | 12.9 | 0 |
7.5 | 0.18 | 0.45 | 4.6 | 0.041 | 67.0 | 158.0 | 0.9927 | 3.01 | 0.38 | 10.6 | 0 |
7.3 | 0.23 | 0.37 | 1.9 | 0.041 | 51.0 | 165.0 | 0.9908 | 3.26 | 0.4 | 12.2 | 0 |
7.1 | 0.12 | 0.3 | 3.1 | 0.018 | 15.0 | 37.0 | 0.99004 | 3.02 | 0.52 | 11.9 | 0 |
7.1 | 0.34 | 0.86 | 1.4 | 0.174 | 36.0 | 99.0 | 0.99288 | 2.92 | 0.5 | 9.3 | 0 |
5.8 | 0.24 | 0.28 | 1.4 | 0.038 | 40.0 | 76.0 | 0.98711 | 3.1 | 0.29 | 13.9 | 0 |
7.3 | 0.34 | 0.33 | 2.5 | 0.064 | 21.0 | 37.0 | 0.9952 | 3.35 | 0.77 | 12.1 | 1 |
9.8 | 0.36 | 0.45 | 1.6 | 0.042 | 11.0 | 124.0 | 0.9944 | 2.93 | 0.46 | 10.8 | 0 |
6.4 | 0.17 | 0.32 | 2.4 | 0.048 | 41.0 | 200.0 | 0.9938 | 3.5 | 0.5 | 9.7 | 0 |
11.9 | 0.39 | 0.69 | 2.8 | 0.095 | 17.0 | 35.0 | 0.9994 | 3.1 | 0.61 | 10.8 | 1 |
7.0 | 0.13 | 0.37 | 12.85 | 0.042 | 36.0 | 105.0 | 0.99581 | 3.05 | 0.55 | 10.7 | 0 |
5.8 | 0.35 | 0.29 | 3.2 | 0.034 | 41.0 | 151.0 | 0.9912 | 3.35 | 0.58 | 11.6333333333333 | 0 |
6.5 | 0.2 | 0.31 | 2.1 | 0.033 | 32.0 | 95.0 | 0.989435 | 2.96 | 0.61 | 12.0 | 0 |
5.5 | 0.32 | 0.45 | 4.9 | 0.028 | 25.0 | 191.0 | 0.9922 | 3.51 | 0.49 | 11.5 | 0 |
7.5 | 0.77 | 0.2 | 8.1 | 0.098 | 30.0 | 92.0 | 0.99892 | 3.2 | 0.58 | 9.2 | 1 |
6.9 | 0.17 | 0.25 | 1.6 | 0.047 | 34.0 | 132.0 | 0.9914 | 3.16 | 0.48 | 11.4 | 0 |
6.6 | 0.25 | 0.35 | 2.9 | 0.034 | 38.0 | 121.0 | 0.99008 | 3.19 | 0.4 | 12.8 | 0 |
6.3 | 0.24 | 0.37 | 1.8 | 0.031 | 6.0 | 61.0 | 0.9897 | 3.3 | 0.34 | 12.2 | 0 |
8.3 | 0.6 | 0.25 | 2.2 | 0.118 | 9.0 | 38.0 | 0.99616 | 3.15 | 0.53 | 9.8 | 1 |
6.6 | 0.39 | 0.39 | 11.9 | 0.057 | 51.0 | 221.0 | 0.99851 | 3.26 | 0.51 | 8.9 | 0 |
7.4 | 0.16 | 0.27 | 15.5 | 0.05 | 25.0 | 135.0 | 0.9984 | 2.9 | 0.43 | 8.7 | 0 |
8.9 | 0.4 | 0.32 | 5.6 | 0.087 | 10.0 | 47.0 | 0.9991 | 3.38 | 0.77 | 10.5 | 1 |
6.2 | 0.12 | 0.26 | 5.7 | 0.044 | 56.0 | 158.0 | 0.9951 | 3.52 | 0.37 | 10.5 | 0 |
6.7 | 0.41 | 0.27 | 2.6 | 0.033 | 25.0 | 85.0 | 0.99086 | 3.05 | 0.34 | 11.7 | 0 |
6.5 | 0.41 | 0.22 | 4.8 | 0.052 | 49.0 | 142.0 | 0.9946 | 3.14 | 0.62 | 9.2 | 0 |
7.1 | 0.6 | 0.0 | 1.8 | 0.074 | 16.0 | 34.0 | 0.9972 | 3.47 | 0.7 | 9.9 | 1 |
7.3 | 0.3 | 0.25 | 2.5 | 0.045 | 32.0 | 122.0 | 0.99329 | 3.18 | 0.54 | 10.3 | 0 |
6.8 | 0.32 | 0.3 | 3.3 | 0.029 | 15.0 | 80.0 | 0.99061 | 3.33 | 0.63 | 12.6 | 0 |
6.7 | 0.28 | 0.28 | 2.4 | 0.012 | 36.0 | 100.0 | 0.99064 | 3.26 | 0.39 | 11.7 | 0 |
6.2 | 0.3 | 0.17 | 2.8 | 0.04 | 24.0 | 125.0 | 0.9939 | 3.01 | 0.46 | 9.0 | 0 |
6.7 | 0.18 | 0.28 | 10.2 | 0.039 | 29.0 | 115.0 | 0.99469 | 3.11 | 0.45 | 10.9 | 0 |
6.8 | 0.15 | 0.41 | 12.9 | 0.044 | 79.5 | 182.0 | 0.99742 | 3.24 | 0.78 | 10.2 | 0 |
8.7 | 0.69 | 0.31 | 3.0 | 0.086 | 23.0 | 81.0 | 1.0002 | 3.48 | 0.74 | 11.6 | 1 |
6.2 | 0.26 | 0.2 | 8.0 | 0.047 | 35.0 | 111.0 | 0.99445 | 3.11 | 0.42 | 10.4 | 0 |
5.9 | 0.25 | 0.19 | 12.4 | 0.047 | 50.0 | 162.0 | 0.9973 | 3.35 | 0.38 | 9.5 | 0 |
8.3 | 0.54 | 0.24 | 3.4 | 0.076 | 16.0 | 112.0 | 0.9976 | 3.27 | 0.61 | 9.4 | 1 |
8.2 | 0.31 | 0.4 | 2.2 | 0.058 | 6.0 | 10.0 | 0.99536 | 3.31 | 0.68 | 11.2 | 1 |
7.0 | 0.24 | 0.24 | 1.8 | 0.047 | 29.0 | 91.0 | 0.99251 | 3.3 | 0.43 | 9.9 | 0 |
7.6 | 0.345 | 0.26 | 1.9 | 0.043 | 15.0 | 134.0 | 0.9936 | 3.08 | 0.38 | 9.5 | 0 |
8.2 | 0.74 | 0.09 | 2.0 | 0.067 | 5.0 | 10.0 | 0.99418 | 3.28 | 0.57 | 11.8 | 1 |
6.9 | 0.23 | 0.34 | 4.0 | 0.047 | 24.0 | 128.0 | 0.9944 | 3.2 | 0.52 | 9.7 | 0 |
7.0 | 0.6 | 0.12 | 2.2 | 0.083 | 13.0 | 28.0 | 0.9966 | 3.52 | 0.62 | 10.2 | 1 |
11.6 | 0.44 | 0.64 | 2.1 | 0.059 | 5.0 | 15.0 | 0.998 | 3.21 | 0.67 | 10.2 | 1 |
7.1 | 0.17 | 0.31 | 1.6 | 0.037 | 15.0 | 103.0 | 0.991 | 3.14 | 0.5 | 12.0 | 0 |
7.4 | 0.19 | 0.3 | 12.8 | 0.053 | 48.5 | 229.0 | 0.9986 | 3.14 | 0.49 | 9.1 | 0 |
6.6 | 0.28 | 0.34 | 0.8 | 0.037 | 42.0 | 119.0 | 0.9888 | 3.03 | 0.37 | 12.5 | 0 |
7.2 | 0.53 | 0.13 | 2.0 | 0.058 | 18.0 | 22.0 | 0.99573 | 3.21 | 0.68 | 9.9 | 1 |
7.7 | 0.39 | 0.28 | 4.9 | 0.035 | 36.0 | 109.0 | 0.9918 | 3.19 | 0.58 | 12.2 | 0 |
7.6 | 0.21 | 0.6 | 2.1 | 0.046 | 47.0 | 165.0 | 0.9936 | 3.05 | 0.54 | 10.1 | 0 |
8.4 | 0.18 | 0.42 | 5.1 | 0.036 | 7.0 | 77.0 | 0.9939 | 3.16 | 0.52 | 11.7 | 0 |
6.4 | 0.42 | 0.46 | 8.4 | 0.05 | 58.0 | 180.0 | 0.99495 | 3.18 | 0.46 | 9.7 | 0 |
6.9 | 0.19 | 0.33 | 1.6 | 0.043 | 63.0 | 149.0 | 0.9925 | 3.44 | 0.52 | 10.8 | 0 |
7.2 | 0.27 | 0.74 | 12.5 | 0.037 | 47.0 | 156.0 | 0.9981 | 3.04 | 0.44 | 8.7 | 0 |
7.7 | 0.39 | 0.12 | 1.7 | 0.097 | 19.0 | 27.0 | 0.99596 | 3.16 | 0.49 | 9.4 | 1 |
7.0 | 0.29 | 0.26 | 1.6 | 0.044 | 12.0 | 87.0 | 0.9923 | 3.08 | 0.46 | 10.5 | 0 |
8.3 | 0.23 | 0.43 | 3.2 | 0.035 | 14.0 | 101.0 | 0.9928 | 3.15 | 0.36 | 11.5 | 0 |
7.4 | 0.34 | 0.3 | 14.9 | 0.037 | 70.0 | 169.0 | 0.99698 | 3.25 | 0.37 | 10.4 | 0 |
8.2 | 0.73 | 0.21 | 1.7 | 0.074 | 5.0 | 13.0 | 0.9968 | 3.2 | 0.52 | 9.5 | 1 |
7.1 | 0.3 | 0.36 | 6.8 | 0.055 | 44.5 | 234.0 | 0.9972 | 3.49 | 0.64 | 10.2 | 0 |
7.1 | 0.59 | 0.0 | 2.2 | 0.078 | 26.0 | 44.0 | 0.99522 | 3.42 | 0.68 | 10.8 | 1 |
6.1 | 0.3 | 0.3 | 2.1 | 0.031 | 50.0 | 163.0 | 0.9895 | 3.39 | 0.43 | 12.7 | 0 |
5.4 | 0.74 | 0.0 | 1.2 | 0.041 | 16.0 | 46.0 | 0.99258 | 4.01 | 0.59 | 12.5 | 1 |
8.4 | 0.29 | 0.29 | 1.05 | 0.032 | 4.0 | 55.0 | 0.9908 | 2.91 | 0.32 | 11.4 | 0 |
7.8 | 0.3 | 0.29 | 16.85 | 0.054 | 23.0 | 135.0 | 0.9998 | 3.16 | 0.38 | 9.0 | 0 |
7.4 | 0.2 | 0.37 | 1.2 | 0.028 | 28.0 | 89.0 | 0.99132 | 3.14 | 0.61 | 11.8 | 0 |
12.5 | 0.37 | 0.55 | 2.6 | 0.083 | 25.0 | 68.0 | 0.9995 | 3.15 | 0.82 | 10.4 | 1 |
6.0 | 0.36 | 0.16 | 6.3 | 0.036 | 36.0 | 191.0 | 0.9942 | 3.17 | 0.62 | 9.8 | 0 |
8.1 | 0.33 | 0.44 | 1.5 | 0.042 | 6.0 | 12.0 | 0.99542 | 3.35 | 0.61 | 10.7 | 1 |
7.6 | 0.39 | 0.32 | 3.6 | 0.035 | 22.0 | 93.0 | 0.99144 | 3.08 | 0.6 | 12.5 | 0 |
5.9 | 0.29 | 0.33 | 7.4 | 0.037 | 58.0 | 205.0 | 0.99495 | 3.26 | 0.41 | 9.6 | 0 |
6.4 | 0.38 | 0.24 | 7.2 | 0.047 | 41.0 | 151.0 | 0.99604 | 3.11 | 0.6 | 9.2 | 0 |
6.0 | 0.16 | 0.36 | 1.6 | 0.042 | 13.0 | 61.0 | 0.99143 | 3.22 | 0.54 | 10.8 | 0 |
6.9 | 0.22 | 0.49 | 7.0 | 0.063 | 50.0 | 168.0 | 0.9957 | 3.54 | 0.5 | 10.3 | 0 |
7.1 | 0.36 | 0.3 | 1.6 | 0.08 | 35.0 | 70.0 | 0.99693 | 3.44 | 0.5 | 9.4 | 1 |
7.3 | 0.13 | 0.27 | 4.6 | 0.08 | 34.0 | 172.0 | 0.9938 | 3.23 | 0.39 | 11.1 | 0 |
6.6 | 0.32 | 0.33 | 2.5 | 0.052 | 40.0 | 219.5 | 0.99316 | 3.15 | 0.6 | 10.0 | 0 |
7.3 | 0.22 | 0.37 | 14.3 | 0.063 | 48.0 | 191.0 | 0.9978 | 2.89 | 0.38 | 9.0 | 0 |
6.6 | 0.25 | 0.3 | 14.4 | 0.052 | 40.0 | 183.0 | 0.998 | 3.02 | 0.5 | 9.1 | 0 |
8.4 | 0.35 | 0.56 | 13.8 | 0.048 | 55.0 | 190.0 | 0.9993 | 3.07 | 0.58 | 9.4 | 0 |
10.2 | 0.645 | 0.36 | 1.8 | 0.053 | 5.0 | 14.0 | 0.9982 | 3.17 | 0.42 | 10.0 | 1 |
6.5 | 0.22 | 0.34 | 12.0 | 0.053 | 55.0 | 177.0 | 0.9983 | 3.52 | 0.44 | 9.9 | 0 |
6.5 | 0.25 | 0.27 | 17.4 | 0.064 | 29.0 | 140.0 | 0.99776 | 3.2 | 0.49 | 10.1 | 0 |
7.6 | 0.18 | 0.28 | 7.1 | 0.041 | 29.0 | 110.0 | 0.99652 | 3.2 | 0.42 | 9.2 | 0 |
5.9 | 0.26 | 0.25 | 12.5 | 0.034 | 38.0 | 152.0 | 0.9977 | 3.33 | 0.43 | 9.4 | 0 |
6.2 | 0.21 | 0.27 | 1.7 | 0.038 | 41.0 | 150.0 | 0.9933 | 3.49 | 0.71 | 10.5 | 0 |
6.8 | 0.19 | 0.32 | 7.6 | 0.049 | 37.0 | 107.0 | 0.99332 | 3.12 | 0.44 | 10.7 | 0 |
6.2 | 0.39 | 0.24 | 4.8 | 0.037 | 45.0 | 138.0 | 0.99174 | 3.23 | 0.43 | 11.2 | 0 |
6.5 | 0.24 | 0.39 | 17.3 | 0.052 | 22.0 | 126.0 | 0.99888 | 3.11 | 0.47 | 9.2 | 0 |
7.1 | 0.28 | 0.31 | 1.5 | 0.053 | 20.0 | 98.0 | 0.99069 | 3.15 | 0.5 | 11.4 | 0 |
6.6 | 0.19 | 0.28 | 11.8 | 0.042 | 54.0 | 137.0 | 0.99492 | 3.18 | 0.37 | 10.8 | 0 |
7.0 | 0.28 | 0.33 | 14.6 | 0.043 | 47.0 | 168.0 | 0.9994 | 3.34 | 0.67 | 8.8 | 0 |
7.3 | 0.27 | 0.37 | 9.7 | 0.042 | 36.0 | 130.0 | 0.9979 | 3.48 | 0.75 | 9.9 | 0 |
6.0 | 0.2 | 0.38 | 1.3 | 0.034 | 37.0 | 104.0 | 0.98865 | 3.11 | 0.52 | 12.7 | 0 |
6.2 | 0.34 | 0.25 | 12.1 | 0.059 | 33.0 | 171.0 | 0.99769 | 3.14 | 0.56 | 8.7 | 0 |
7.4 | 0.29 | 0.28 | 10.2 | 0.032 | 43.0 | 138.0 | 0.9951 | 3.1 | 0.47 | 10.6 | 0 |
6.1 | 0.46 | 0.32 | 6.2 | 0.053 | 10.0 | 94.0 | 0.99537 | 3.35 | 0.47 | 10.1 | 0 |
8.2 | 0.23 | 0.29 | 1.8 | 0.047 | 47.0 | 187.0 | 0.9933 | 3.13 | 0.5 | 10.2 | 0 |
8.2 | 0.28 | 0.42 | 1.8 | 0.031 | 30.0 | 93.0 | 0.9917 | 3.09 | 0.39 | 11.4 | 0 |
7.6 | 0.28 | 0.39 | 1.2 | 0.038 | 21.0 | 115.0 | 0.994 | 3.16 | 0.67 | 10.0 | 0 |
7.0 | 0.34 | 0.3 | 1.8 | 0.045 | 44.0 | 142.0 | 0.9914 | 2.99 | 0.45 | 10.8 | 0 |
7.6 | 0.5 | 0.29 | 2.3 | 0.086 | 5.0 | 14.0 | 0.99502 | 3.32 | 0.62 | 11.5 | 1 |
5.9 | 0.29 | 0.16 | 7.9 | 0.044 | 48.0 | 197.0 | 0.99512 | 3.21 | 0.36 | 9.4 | 0 |
8.7 | 0.765 | 0.22 | 2.3 | 0.064 | 9.0 | 42.0 | 0.9963 | 3.1 | 0.55 | 9.4 | 1 |
7.0 | 0.24 | 0.26 | 1.7 | 0.041 | 31.0 | 110.0 | 0.99142 | 3.2 | 0.53 | 11.0 | 0 |
6.3 | 0.68 | 0.01 | 3.7 | 0.103 | 32.0 | 54.0 | 0.99586 | 3.51 | 0.66 | 11.3 | 1 |
5.8 | 0.26 | 0.29 | 1.0 | 0.042 | 35.0 | 101.0 | 0.99044 | 3.36 | 0.48 | 11.4 | 0 |
7.8 | 0.15 | 0.34 | 1.1 | 0.035 | 31.0 | 93.0 | 0.99096 | 3.07 | 0.72 | 11.3 | 0 |
7.3 | 0.18 | 0.31 | 17.3 | 0.055 | 32.0 | 197.0 | 1.0002 | 3.13 | 0.46 | 9.0 | 0 |
7.6 | 0.35 | 0.46 | 14.7 | 0.047 | 33.0 | 151.0 | 0.99709 | 3.03 | 0.53 | 10.3 | 0 |
15.5 | 0.645 | 0.49 | 4.2 | 0.095 | 10.0 | 23.0 | 1.00315 | 2.92 | 0.74 | 11.1 | 1 |
6.2 | 0.17 | 0.3 | 1.1 | 0.037 | 14.0 | 79.0 | 0.993 | 3.5 | 0.54 | 10.3 | 0 |
7.1 | 0.35 | 0.27 | 3.1 | 0.034 | 28.0 | 134.0 | 0.9897 | 3.26 | 0.38 | 13.1 | 0 |
8.6 | 0.37 | 0.65 | 6.4 | 0.08 | 3.0 | 8.0 | 0.99817 | 3.27 | 0.58 | 11.0 | 1 |
8.5 | 0.17 | 0.31 | 1.0 | 0.024 | 13.0 | 91.0 | 0.993 | 2.79 | 0.37 | 10.1 | 0 |
5.6 | 0.41 | 0.22 | 7.1 | 0.05 | 44.0 | 154.0 | 0.9931 | 3.3 | 0.4 | 10.5 | 0 |
6.7 | 0.24 | 0.41 | 8.7 | 0.036 | 29.0 | 148.0 | 0.9952 | 3.22 | 0.62 | 9.9 | 0 |
5.8 | 0.28 | 0.3 | 3.9 | 0.026 | 36.0 | 105.0 | 0.98963 | 3.26 | 0.58 | 12.75 | 0 |
6.1 | 0.22 | 0.49 | 1.5 | 0.051 | 18.0 | 87.0 | 0.9928 | 3.3 | 0.46 | 9.6 | 0 |
5.3 | 0.76 | 0.03 | 2.7 | 0.043 | 27.0 | 93.0 | 0.9932 | 3.34 | 0.38 | 9.2 | 0 |
6.1 | 0.64 | 0.02 | 2.4 | 0.069 | 26.0 | 46.0 | 0.99358 | 3.47 | 0.45 | 11.0 | 1 |
8.5 | 0.25 | 0.27 | 4.7 | 0.031 | 31.0 | 92.0 | 0.9922 | 3.01 | 0.33 | 12.0 | 0 |
6.0 | 0.54 | 0.06 | 1.8 | 0.05 | 38.0 | 89.0 | 0.99236 | 3.3 | 0.5 | 10.55 | 1 |
7.9 | 0.21 | 0.4 | 1.2 | 0.039 | 38.0 | 107.0 | 0.992 | 3.21 | 0.54 | 10.8 | 0 |
5.6 | 0.26 | 0.26 | 5.7 | 0.031 | 12.0 | 80.0 | 0.9923 | 3.25 | 0.38 | 10.8 | 0 |
7.0 | 0.69 | 0.07 | 2.5 | 0.091 | 15.0 | 21.0 | 0.99572 | 3.38 | 0.6 | 11.3 | 1 |
5.0 | 1.02 | 0.04 | 1.4 | 0.045 | 41.0 | 85.0 | 0.9938 | 3.75 | 0.48 | 10.5 | 1 |
7.4 | 0.6 | 0.26 | 2.1 | 0.083 | 17.0 | 91.0 | 0.99616 | 3.29 | 0.56 | 9.8 | 1 |
8.0 | 0.38 | 0.44 | 1.9 | 0.098 | 6.0 | 15.0 | 0.9956 | 3.3 | 0.64 | 11.4 | 1 |
6.6 | 0.25 | 0.31 | 12.4 | 0.059 | 52.0 | 181.0 | 0.9984 | 3.51 | 0.47 | 9.8 | 0 |
8.0 | 0.3 | 0.36 | 11.0 | 0.034 | 8.0 | 70.0 | 0.99354 | 3.05 | 0.41 | 12.2 | 0 |
6.1 | 0.21 | 0.3 | 6.3 | 0.039 | 47.0 | 136.0 | 0.99068 | 3.27 | 0.31 | 12.7 | 0 |
6.5 | 0.18 | 0.31 | 1.7 | 0.044 | 30.0 | 127.0 | 0.9928 | 3.49 | 0.5 | 10.2 | 0 |
6.2 | 0.33 | 0.19 | 5.6 | 0.042 | 22.0 | 143.0 | 0.99425 | 3.15 | 0.63 | 9.9 | 0 |
5.5 | 0.485 | 0.0 | 1.5 | 0.065 | 8.0 | 103.0 | 0.994 | 3.63 | 0.4 | 9.7 | 0 |
8.5 | 0.28 | 0.34 | 13.8 | 0.041 | 32.0 | 161.0 | 0.9981 | 3.13 | 0.4 | 9.9 | 0 |
6.0 | 0.17 | 0.29 | 5.0 | 0.028 | 25.0 | 108.0 | 0.99076 | 3.14 | 0.34 | 12.3 | 0 |
5.6 | 0.695 | 0.06 | 6.8 | 0.042 | 9.0 | 84.0 | 0.99432 | 3.44 | 0.44 | 10.2 | 0 |
7.6 | 0.3 | 0.4 | 2.2 | 0.054 | 29.0 | 175.0 | 0.99445 | 3.19 | 0.53 | 9.8 | 0 |
7.3 | 0.51 | 0.18 | 2.1 | 0.07 | 12.0 | 28.0 | 0.99768 | 3.52 | 0.73 | 9.5 | 1 |
6.7 | 0.41 | 0.27 | 2.6 | 0.033 | 25.0 | 85.0 | 0.99086 | 3.05 | 0.34 | 11.7 | 0 |
6.7 | 0.28 | 0.34 | 8.9 | 0.048 | 32.0 | 111.0 | 0.99455 | 3.25 | 0.54 | 11.0 | 0 |
from pyspark.sql.functions import struct
# Apply the model to the new data
udf_inputs = struct(*(X_train.columns.tolist()))
new_data = new_data.withColumn(
"prediction",
apply_model_udf(udf_inputs)
)
# Each row now has an associated prediction. Note that the xgboost function does not output probabilities by default, so the predictions are not limited to the range [0, 1].
display(new_data)
fixed_acidity | volatile_acidity | citric_acid | residual_sugar | chlorides | free_sulfur_dioxide | total_sulfur_dioxide | density | pH | sulphates | alcohol | is_red | prediction |
---|---|---|---|---|---|---|---|---|---|---|---|---|
7.2 | 0.23 | 0.39 | 2.3 | 0.033 | 29.0 | 102.0 | 0.9908 | 3.26 | 0.54 | 12.3 | 0 | 0.9751631617546082 |
6.4 | 0.24 | 0.27 | 1.5 | 0.04 | 35.0 | 105.0 | 0.98914 | 3.13 | 0.3 | 12.4 | 0 | 0.01992025412619114 |
7.4 | 0.24 | 0.29 | 10.1 | 0.05 | 21.0 | 105.0 | 0.9962 | 3.13 | 0.35 | 9.5 | 0 | 7.046426762826741E-4 |
8.6 | 0.37 | 0.65 | 6.4 | 0.08 | 3.0 | 8.0 | 0.99817 | 3.27 | 0.58 | 11.0 | 1 | 0.011003587394952774 |
5.9 | 0.32 | 0.39 | 3.3 | 0.114 | 24.0 | 140.0 | 0.9934 | 3.09 | 0.45 | 9.2 | 0 | 0.00361012015491724 |
7.0 | 0.42 | 0.35 | 1.6 | 0.088 | 16.0 | 39.0 | 0.9961 | 3.34 | 0.55 | 9.2 | 1 | 0.0010293669765815139 |
6.7 | 0.31 | 0.44 | 6.7 | 0.054 | 29.0 | 160.0 | 0.9952 | 3.04 | 0.44 | 9.6 | 0 | 0.0010825126664713025 |
8.1 | 0.545 | 0.18 | 1.9 | 0.08 | 13.0 | 35.0 | 0.9972 | 3.3 | 0.59 | 9.0 | 1 | 0.0016736732795834541 |
6.3 | 0.41 | 0.3 | 3.2 | 0.03 | 49.0 | 164.0 | 0.9927 | 3.53 | 0.79 | 11.7 | 0 | 0.9625353217124939 |
7.3 | 0.49 | 0.32 | 5.2 | 0.043 | 18.0 | 104.0 | 0.9952 | 3.24 | 0.45 | 10.7 | 0 | 0.012135892175137997 |
5.7 | 0.24 | 0.47 | 6.3 | 0.069 | 35.0 | 182.0 | 0.99391 | 3.11 | 0.46 | 9.73333333333333 | 0 | 0.002645439701154828 |
7.2 | 0.23 | 0.32 | 8.5 | 0.058 | 47.0 | 186.0 | 0.9956 | 3.19 | 0.4 | 9.9 | 0 | 0.0032776198349893093 |
6.9 | 0.31 | 0.34 | 7.4 | 0.059 | 36.0 | 174.0 | 0.9963 | 3.46 | 0.62 | 11.1 | 0 | 0.9063054323196411 |
6.0 | 0.26 | 0.32 | 3.5 | 0.028 | 29.0 | 113.0 | 0.9912 | 3.4 | 0.71 | 12.3 | 0 | 0.9731684327125549 |
6.5 | 0.32 | 0.12 | 11.5 | 0.033 | 35.0 | 165.0 | 0.9974 | 3.22 | 0.32 | 9.0 | 0 | 0.001156796352006495 |
7.1 | 0.21 | 0.3 | 1.4 | 0.037 | 45.0 | 143.0 | 0.9932 | 3.13 | 0.33 | 9.9 | 0 | 0.011401111260056496 |
8.2 | 0.27 | 0.39 | 7.8 | 0.039 | 49.0 | 208.0 | 0.9976 | 3.31 | 0.51 | 9.5 | 0 | 0.009010124020278454 |
6.8 | 0.26 | 0.24 | 7.8 | 0.052 | 54.0 | 214.0 | 0.9961 | 3.13 | 0.47 | 8.9 | 0 | 0.0013020867481827736 |
7.0 | 0.36 | 0.35 | 2.5 | 0.048 | 67.0 | 161.0 | 0.99146 | 3.05 | 0.56 | 11.1 | 0 | 0.0716884657740593 |
7.2 | 0.25 | 0.32 | 1.5 | 0.054 | 24.0 | 105.0 | 0.99154 | 3.17 | 0.48 | 11.1 | 0 | 0.007840806618332863 |
8.4 | 0.19 | 0.43 | 2.1 | 0.052 | 20.0 | 104.0 | 0.994 | 2.85 | 0.46 | 9.5 | 0 | 0.00898531824350357 |
5.6 | 0.615 | 0.0 | 1.6 | 0.089 | 16.0 | 59.0 | 0.9943 | 3.58 | 0.52 | 9.9 | 1 | 0.0019848872907459736 |
6.6 | 0.085 | 0.33 | 1.4 | 0.036 | 17.0 | 109.0 | 0.99306 | 3.27 | 0.61 | 9.5 | 0 | 0.017587896436452866 |
8.8 | 0.39 | 0.35 | 1.8 | 0.096 | 22.0 | 80.0 | 0.99016 | 2.95 | 0.54 | 12.6 | 0 | 0.01584608294069767 |
6.0 | 0.27 | 0.28 | 4.8 | 0.063 | 31.0 | 201.0 | 0.9964 | 3.69 | 0.71 | 10.0 | 0 | 0.010482852347195148 |
7.3 | 0.32 | 0.23 | 2.3 | 0.066 | 35.0 | 70.0 | 0.99588 | 3.43 | 0.62 | 10.1 | 1 | 0.005606499500572681 |
9.2 | 0.71 | 0.23 | 6.2 | 0.042 | 15.0 | 93.0 | 0.9948 | 2.89 | 0.34 | 10.1 | 0 | 6.88909029122442E-4 |
7.5 | 0.22 | 0.32 | 2.4 | 0.045 | 29.0 | 100.0 | 0.99135 | 3.08 | 0.6 | 11.3 | 0 | 0.9589137434959412 |
7.5 | 0.14 | 0.74 | 1.6 | 0.035 | 21.0 | 126.0 | 0.9933 | 3.26 | 0.45 | 10.2 | 0 | 0.009778814390301704 |
5.6 | 0.245 | 0.32 | 1.1 | 0.047 | 24.0 | 152.0 | 0.9927 | 3.12 | 0.42 | 9.3 | 0 | 0.0013140885857865214 |
5.8 | 0.28 | 0.66 | 9.1 | 0.039 | 26.0 | 159.0 | 0.9965 | 3.66 | 0.55 | 10.8 | 0 | 0.02977527678012848 |
6.3 | 0.15 | 0.3 | 1.4 | 0.022 | 38.0 | 100.0 | 0.99099 | 3.42 | 0.57 | 11.4 | 0 | 0.9320000410079956 |
7.1 | 0.37 | 0.3 | 6.2 | 0.04 | 49.0 | 139.0 | 0.99021 | 3.17 | 0.27 | 13.6 | 0 | 0.05198657140135765 |
6.5 | 0.29 | 0.53 | 1.7 | 0.04 | 41.0 | 192.0 | 0.9922 | 3.26 | 0.59 | 10.4 | 0 | 0.8902062773704529 |
7.4 | 0.28 | 0.5 | 12.1 | 0.049 | 48.0 | 122.0 | 0.9973 | 3.01 | 0.44 | 9.0 | 0 | 0.004505288787186146 |
7.1 | 0.32 | 0.24 | 13.1 | 0.05 | 52.0 | 204.0 | 0.998 | 3.1 | 0.49 | 8.8 | 0 | 0.010533100925385952 |
6.0 | 0.27 | 0.32 | 3.6 | 0.035 | 36.0 | 133.0 | 0.99215 | 3.23 | 0.46 | 10.8 | 0 | 0.015155753120779991 |
9.2 | 0.18 | 0.49 | 1.5 | 0.041 | 39.0 | 130.0 | 0.9945 | 3.04 | 0.49 | 9.8 | 0 | 0.9047970771789551 |
6.6 | 0.84 | 0.03 | 2.3 | 0.059 | 32.0 | 48.0 | 0.9952 | 3.52 | 0.56 | 12.3 | 1 | 0.9120600819587708 |
7.6 | 0.14 | 0.74 | 1.6 | 0.04 | 27.0 | 103.0 | 0.9916 | 3.07 | 0.4 | 10.8 | 0 | 0.8818873763084412 |
5.5 | 0.29 | 0.3 | 1.1 | 0.022 | 20.0 | 110.0 | 0.98869 | 3.34 | 0.38 | 12.8 | 0 | 0.9537860155105591 |
7.2 | 0.22 | 0.28 | 7.2 | 0.06 | 41.0 | 132.0 | 0.9935 | 3.08 | 0.59 | 11.3 | 0 | 0.11146280914545059 |
7.6 | 0.27 | 0.29 | 2.5 | 0.059 | 37.0 | 115.0 | 0.99328 | 3.09 | 0.37 | 9.8 | 0 | 0.0033767824061214924 |
7.3 | 0.2 | 0.39 | 2.3 | 0.048 | 24.0 | 87.0 | 0.99044 | 2.94 | 0.35 | 12.0 | 0 | 0.028567757457494736 |
7.2 | 0.66 | 0.03 | 2.3 | 0.078 | 16.0 | 86.0 | 0.99743 | 3.53 | 0.57 | 9.7 | 1 | 5.143549642525613E-4 |
7.6 | 0.33 | 0.36 | 2.1 | 0.034 | 26.0 | 172.0 | 0.9944 | 3.42 | 0.48 | 10.5 | 0 | 0.05245935171842575 |
9.0 | 0.43 | 0.3 | 1.5 | 0.05 | 7.0 | 175.0 | 0.9951 | 3.11 | 0.45 | 9.7 | 0 | 4.268118354957551E-4 |
8.0 | 0.17 | 0.29 | 2.4 | 0.029 | 52.0 | 119.0 | 0.98944 | 3.03 | 0.33 | 12.9 | 0 | 0.04263770952820778 |
7.4 | 0.27 | 0.31 | 2.4 | 0.014 | 15.0 | 143.0 | 0.99094 | 3.03 | 0.65 | 12.0 | 0 | 0.03980858996510506 |
8.0 | 0.27 | 0.33 | 1.2 | 0.05 | 41.0 | 103.0 | 0.99002 | 3.0 | 0.45 | 12.4 | 0 | 0.005018413532525301 |
7.5 | 0.21 | 0.29 | 1.5 | 0.046 | 35.0 | 107.0 | 0.99123 | 3.15 | 0.45 | 11.3 | 0 | 0.06088990345597267 |
7.3 | 0.39 | 0.31 | 2.4 | 0.074 | 9.0 | 46.0 | 0.9962 | 3.41 | 0.54 | 9.4 | 1 | 0.0020936760120093822 |
6.9 | 0.19 | 0.35 | 13.5 | 0.038 | 49.0 | 118.0 | 0.99546 | 3.0 | 0.63 | 10.7 | 0 | 0.018375016748905182 |
7.3 | 0.25 | 0.28 | 1.5 | 0.043 | 19.0 | 113.0 | 0.99338 | 3.38 | 0.56 | 10.1 | 0 | 0.015154771506786346 |
8.0 | 0.19 | 0.3 | 2.0 | 0.053 | 48.0 | 140.0 | 0.994 | 3.18 | 0.49 | 9.6 | 0 | 0.010957462713122368 |
6.4 | 0.24 | 0.32 | 0.95 | 0.041 | 23.0 | 131.0 | 0.99033 | 3.25 | 0.35 | 11.8 | 0 | 0.00997467152774334 |
6.6 | 0.2 | 0.14 | 4.4 | 0.184 | 35.0 | 168.0 | 0.99396 | 2.93 | 0.45 | 9.4 | 0 | 0.004101524129509926 |
6.1 | 0.15 | 0.29 | 6.2 | 0.046 | 39.0 | 151.0 | 0.99471 | 3.6 | 0.44 | 10.6 | 0 | 0.00821105670183897 |
8.8 | 0.6 | 0.29 | 2.2 | 0.098 | 5.0 | 15.0 | 0.9988 | 3.36 | 0.49 | 9.1 | 1 | 0.002779798349365592 |
7.1 | 0.38 | 0.29 | 13.6 | 0.041 | 30.0 | 137.0 | 0.99461 | 3.02 | 0.96 | 12.1 | 0 | 0.0432555116713047 |
7.1 | 0.21 | 0.72 | 1.6 | 0.167 | 65.0 | 120.0 | 0.99324 | 2.97 | 0.51 | 9.2 | 0 | 0.014048242941498756 |
7.5 | 0.29 | 0.36 | 15.7 | 0.05 | 29.0 | 124.0 | 0.9968 | 3.06 | 0.54 | 10.4 | 0 | 0.005064977332949638 |
5.4 | 0.15 | 0.32 | 2.5 | 0.037 | 10.0 | 51.0 | 0.98878 | 3.04 | 0.58 | 12.6 | 0 | 0.040418840944767 |
7.2 | 0.39 | 0.63 | 11.0 | 0.044 | 55.0 | 156.0 | 0.9974 | 3.09 | 0.44 | 8.7 | 0 | 8.166952757164836E-4 |
7.6 | 0.26 | 0.32 | 1.3 | 0.048 | 23.0 | 76.0 | 0.9903 | 2.96 | 0.46 | 12.0 | 0 | 0.00441924249753356 |
11.8 | 0.23 | 0.38 | 11.1 | 0.034 | 15.0 | 123.0 | 0.9997 | 2.93 | 0.55 | 9.7 | 0 | 0.02016282081604004 |
6.0 | 0.19 | 0.37 | 9.7 | 0.032 | 17.0 | 50.0 | 0.9932 | 3.08 | 0.66 | 12.0 | 0 | 0.05290091410279274 |
8.1 | 0.28 | 0.34 | 1.3 | 0.035 | 11.0 | 126.0 | 0.99232 | 3.14 | 0.5 | 9.8 | 0 | 0.004231251776218414 |
6.3 | 0.23 | 0.21 | 5.1 | 0.035 | 29.0 | 142.0 | 0.9942 | 3.36 | 0.33 | 10.1 | 0 | 0.9384641051292419 |
6.4 | 0.28 | 0.36 | 1.3 | 0.053 | 28.0 | 186.0 | 0.99211 | 3.31 | 0.45 | 10.8 | 0 | 0.0073373401537537575 |
10.0 | 0.73 | 0.43 | 2.3 | 0.059 | 15.0 | 31.0 | 0.9966 | 3.15 | 0.57 | 11.0 | 1 | 0.00571498554199934 |
6.5 | 0.5 | 0.22 | 4.1 | 0.036 | 35.0 | 131.0 | 0.9902 | 3.26 | 0.55 | 13.0 | 0 | 0.953966498374939 |
7.0 | 0.36 | 0.3 | 5.0 | 0.04 | 40.0 | 143.0 | 0.99173 | 3.33 | 0.42 | 12.2 | 0 | 0.8731345534324646 |
5.7 | 0.23 | 0.28 | 9.65 | 0.025 | 26.0 | 121.0 | 0.9925 | 3.28 | 0.38 | 11.3 | 0 | 0.04899931326508522 |
6.8 | 0.3 | 0.26 | 20.3 | 0.037 | 45.0 | 150.0 | 0.99727 | 3.04 | 0.38 | 12.3 | 0 | 0.01285466831177473 |
7.5 | 0.22 | 0.33 | 6.7 | 0.036 | 45.0 | 138.0 | 0.9939 | 3.2 | 0.68 | 11.4 | 0 | 0.03544529154896736 |
5.1 | 0.35 | 0.26 | 6.8 | 0.034 | 36.0 | 120.0 | 0.99188 | 3.38 | 0.4 | 11.5 | 0 | 0.02100011520087719 |
6.15 | 0.21 | 0.37 | 3.2 | 0.021 | 20.0 | 80.0 | 0.99076 | 3.39 | 0.47 | 12.0 | 0 | 0.017040716484189034 |
6.0 | 0.21 | 0.3 | 8.7 | 0.036 | 47.0 | 127.0 | 0.99368 | 3.18 | 0.39 | 10.6 | 0 | 0.009342623874545097 |
6.2 | 0.23 | 0.36 | 17.2 | 0.039 | 37.0 | 130.0 | 0.99946 | 3.23 | 0.43 | 8.8 | 0 | 7.341491291299462E-4 |
7.4 | 0.25 | 0.36 | 13.2 | 0.067 | 53.0 | 178.0 | 0.9976 | 3.01 | 0.48 | 9.0 | 0 | 0.02032722532749176 |
6.5 | 0.22 | 0.28 | 3.7 | 0.059 | 29.0 | 151.0 | 0.99177 | 3.23 | 0.41 | 12.1 | 0 | 0.9381306171417236 |
7.5 | 0.38 | 0.29 | 4.9 | 0.021 | 38.0 | 113.0 | 0.99026 | 3.08 | 0.48 | 13.0 | 0 | 0.9875358939170837 |
6.6 | 0.27 | 0.33 | 1.4 | 0.042 | 24.0 | 183.0 | 0.99215 | 3.29 | 0.46 | 10.7 | 0 | 0.005234047770500183 |
7.9 | 0.17 | 0.32 | 1.6 | 0.053 | 47.0 | 150.0 | 0.9948 | 3.29 | 0.76 | 9.6 | 0 | 0.003185991896316409 |
8.0 | 0.2 | 0.3 | 8.1 | 0.037 | 42.0 | 130.0 | 0.99379 | 3.1 | 0.67 | 11.8 | 0 | 0.03378769010305405 |
7.9 | 0.16 | 0.3 | 7.4 | 0.05 | 58.0 | 152.0 | 0.99612 | 3.12 | 0.37 | 9.5 | 0 | 0.001650699065066874 |
6.6 | 0.3 | 0.3 | 4.8 | 0.17 | 60.0 | 166.0 | 0.9946 | 3.18 | 0.47 | 9.4 | 0 | 0.0019682596903294325 |
7.3 | 0.59 | 0.26 | 7.2 | 0.07 | 35.0 | 121.0 | 0.9981 | 3.37 | 0.49 | 9.4 | 1 | 0.0014398160856217146 |
7.0 | 0.15 | 0.34 | 1.4 | 0.039 | 21.0 | 177.0 | 0.9927 | 3.32 | 0.62 | 10.8 | 0 | 0.0142179811373353 |
6.3 | 0.25 | 0.53 | 1.8 | 0.021 | 41.0 | 101.0 | 0.989315 | 3.19 | 0.31 | 13.0 | 0 | 0.09868034720420837 |
6.7 | 0.25 | 0.33 | 2.9 | 0.057 | 52.0 | 173.0 | 0.9934 | 3.02 | 0.48 | 9.5 | 0 | 0.8918036222457886 |
6.1 | 0.41 | 0.14 | 10.4 | 0.037 | 18.0 | 119.0 | 0.996 | 3.38 | 0.45 | 10.0 | 0 | 0.016627971082925797 |
7.1 | 0.75 | 0.01 | 2.2 | 0.059 | 11.0 | 18.0 | 0.99242 | 3.39 | 0.4 | 12.8 | 1 | 0.008807744830846786 |
7.1 | 0.37 | 0.67 | 10.5 | 0.045 | 49.0 | 155.0 | 0.9975 | 3.16 | 0.44 | 8.7 | 0 | 0.0011050212197005749 |
8.3 | 0.61 | 0.3 | 2.1 | 0.084 | 11.0 | 50.0 | 0.9972 | 3.4 | 0.61 | 10.2 | 1 | 0.0070987269282341 |
7.5 | 0.33 | 0.28 | 4.9 | 0.042 | 21.0 | 155.0 | 0.99385 | 3.36 | 0.57 | 10.9 | 0 | 0.004508147016167641 |
8.9 | 0.34 | 0.34 | 1.6 | 0.056 | 13.0 | 176.0 | 0.9946 | 3.14 | 0.47 | 9.7 | 0 | 7.869903929531574E-4 |
6.5 | 0.28 | 0.35 | 9.8 | 0.067 | 61.0 | 180.0 | 0.9972 | 3.15 | 0.57 | 9.0 | 0 | 0.002090145368129015 |
7.7 | 0.16 | 0.36 | 5.9 | 0.054 | 25.0 | 148.0 | 0.99578 | 3.25 | 0.54 | 10.2 | 0 | 0.005096611101180315 |
7.5 | 0.52 | 0.16 | 1.9 | 0.085 | 12.0 | 35.0 | 0.9968 | 3.38 | 0.62 | 9.5 | 1 | 0.8352470397949219 |
7.4 | 0.35 | 0.2 | 13.9 | 0.054 | 63.0 | 229.0 | 0.99888 | 3.11 | 0.5 | 8.9 | 0 | 0.0011468409793451428 |
7.3 | 0.29 | 0.37 | 8.3 | 0.044 | 45.0 | 227.0 | 0.9966 | 3.12 | 0.47 | 9.0 | 0 | 7.734125829301775E-4 |
5.6 | 0.66 | 0.0 | 2.2 | 0.087 | 3.0 | 11.0 | 0.99378 | 3.71 | 0.63 | 12.8 | 1 | 0.9435859322547913 |
5.9 | 0.65 | 0.23 | 5.0 | 0.035 | 20.0 | 128.0 | 0.99016 | 3.46 | 0.48 | 12.8 | 0 | 0.05909093841910362 |
6.7 | 0.34 | 0.4 | 2.1 | 0.033 | 34.0 | 111.0 | 0.98924 | 2.97 | 0.48 | 12.2 | 0 | 0.9525443911552429 |
7.4 | 0.29 | 0.29 | 1.6 | 0.045 | 53.0 | 180.0 | 0.9936 | 3.34 | 0.68 | 10.5 | 0 | 0.04980198293924332 |
8.1 | 0.2 | 0.28 | 0.9 | 0.023 | 49.0 | 87.0 | 0.99062 | 2.92 | 0.36 | 11.1 | 0 | 0.016284562647342682 |
7.2 | 0.37 | 0.32 | 2.0 | 0.062 | 15.0 | 28.0 | 0.9947 | 3.23 | 0.73 | 11.3 | 1 | 0.9765864610671997 |
7.0 | 0.805 | 0.0 | 2.5 | 0.068 | 7.0 | 20.0 | 0.9969 | 3.48 | 0.56 | 9.6 | 1 | 0.0016839306335896254 |
4.9 | 0.335 | 0.14 | 1.3 | 0.036 | 69.0 | 168.0 | 0.99212 | 3.47 | 0.46 | 10.4666666666667 | 0 | 0.005408146418631077 |
7.3 | 0.33 | 0.47 | 2.1 | 0.077 | 5.0 | 11.0 | 0.9958 | 3.33 | 0.53 | 10.3 | 1 | 0.001486302469857037 |
8.8 | 0.28 | 0.45 | 6.0 | 0.022 | 14.0 | 49.0 | 0.9934 | 3.01 | 0.33 | 11.1 | 0 | 0.8960228562355042 |
7.1 | 0.63 | 0.06 | 2.0 | 0.083 | 8.0 | 29.0 | 0.99855 | 3.67 | 0.73 | 9.6 | 1 | 0.004544001538306475 |
6.9 | 0.29 | 0.4 | 19.45 | 0.043 | 36.0 | 156.0 | 0.9996 | 2.93 | 0.47 | 8.9 | 0 | 0.008597329258918762 |
6.7 | 0.26 | 0.49 | 8.3 | 0.047 | 54.0 | 191.0 | 0.9954 | 3.23 | 0.4 | 10.3 | 0 | 0.002832855563610792 |
7.7 | 0.23 | 0.37 | 1.8 | 0.046 | 23.0 | 60.0 | 0.9971 | 3.41 | 0.71 | 12.1 | 1 | 0.028161071240901947 |
5.8 | 0.2 | 0.16 | 1.4 | 0.042 | 44.0 | 99.0 | 0.98912 | 3.23 | 0.37 | 12.2 | 0 | 0.00863594003021717 |
7.4 | 0.635 | 0.1 | 2.4 | 0.08 | 16.0 | 33.0 | 0.99736 | 3.58 | 0.69 | 10.8 | 1 | 0.7882183790206909 |
6.2 | 0.41 | 0.22 | 1.9 | 0.023 | 5.0 | 56.0 | 0.98928 | 3.04 | 0.79 | 13.0 | 0 | 0.9526894688606262 |
7.7 | 0.38 | 0.4 | 2.0 | 0.038 | 28.0 | 152.0 | 0.9906 | 3.18 | 0.32 | 12.9 | 0 | 0.10345277190208435 |
9.3 | 0.2 | 0.33 | 1.7 | 0.05 | 28.0 | 178.0 | 0.9954 | 3.16 | 0.43 | 9.0 | 0 | 0.019169747829437256 |
8.2 | 0.3 | 0.44 | 12.4 | 0.043 | 52.0 | 154.0 | 0.99452 | 3.04 | 0.33 | 12.0 | 0 | 0.018337378278374672 |
5.6 | 0.29 | 0.05 | 0.8 | 0.038 | 11.0 | 30.0 | 0.9924 | 3.36 | 0.35 | 9.2 | 0 | 0.002770686289295554 |
9.8 | 0.98 | 0.32 | 2.3 | 0.078 | 35.0 | 152.0 | 0.998 | 3.25 | 0.48 | 9.4 | 1 | 0.0011920671677216887 |
6.2 | 0.29 | 0.23 | 12.4 | 0.048 | 33.0 | 201.0 | 0.99612 | 3.11 | 0.56 | 9.9 | 0 | 7.936731562949717E-4 |
6.7 | 0.28 | 0.34 | 8.9 | 0.048 | 32.0 | 111.0 | 0.99455 | 3.25 | 0.54 | 11.0 | 0 | 0.9808989763259888 |
7.2 | 0.21 | 0.34 | 1.1 | 0.046 | 25.0 | 80.0 | 0.992 | 3.25 | 0.4 | 11.3 | 0 | 0.010724720545113087 |
7.0 | 0.43 | 0.3 | 2.0 | 0.085 | 6.0 | 39.0 | 0.99346 | 3.33 | 0.46 | 11.9 | 1 | 0.0028391489759087563 |
6.3 | 0.23 | 0.22 | 3.75 | 0.039 | 37.0 | 116.0 | 0.9927 | 3.23 | 0.5 | 10.7 | 0 | 0.00362543691881001 |
6.4 | 0.69 | 0.0 | 1.65 | 0.055 | 7.0 | 12.0 | 0.99162 | 3.47 | 0.53 | 12.9 | 1 | 0.038824670016765594 |
6.7 | 0.15 | 0.32 | 7.9 | 0.034 | 17.0 | 81.0 | 0.99512 | 3.29 | 0.31 | 10.0 | 0 | 0.022858623415231705 |
6.3 | 0.26 | 0.25 | 7.8 | 0.058 | 44.0 | 166.0 | 0.9961 | 3.24 | 0.41 | 9.0 | 0 | 0.001252196030691266 |
7.5 | 0.305 | 0.38 | 1.4 | 0.047 | 30.0 | 95.0 | 0.99158 | 3.22 | 0.52 | 11.5 | 0 | 0.9291372895240784 |
7.9 | 0.19 | 0.45 | 1.5 | 0.045 | 17.0 | 96.0 | 0.9917 | 3.13 | 0.39 | 11.0 | 0 | 0.03443913906812668 |
6.8 | 0.19 | 0.34 | 1.9 | 0.04 | 41.0 | 108.0 | 0.99 | 3.25 | 0.45 | 12.9 | 0 | 0.041260771453380585 |
6.7 | 0.3 | 0.45 | 10.6 | 0.032 | 56.0 | 212.0 | 0.997 | 3.22 | 0.59 | 9.5 | 0 | 0.004056371748447418 |
5.6 | 0.15 | 0.31 | 5.3 | 0.038 | 8.0 | 79.0 | 0.9923 | 3.3 | 0.39 | 10.5 | 0 | 0.05107461288571358 |
6.6 | 0.61 | 0.01 | 1.9 | 0.08 | 8.0 | 25.0 | 0.99746 | 3.69 | 0.73 | 10.5 | 1 | 0.008754988200962543 |
6.6 | 0.23 | 0.3 | 4.6 | 0.06 | 29.0 | 154.0 | 0.99142 | 3.23 | 0.49 | 12.2 | 0 | 0.9374986886978149 |
6.8 | 0.28 | 0.44 | 11.5 | 0.04 | 58.0 | 223.0 | 0.9969 | 3.22 | 0.56 | 9.5 | 0 | 0.0020736174192279577 |
5.2 | 0.405 | 0.15 | 1.45 | 0.038 | 10.0 | 44.0 | 0.99125 | 3.52 | 0.4 | 11.6 | 0 | 0.007123155519366264 |
7.0 | 0.31 | 0.39 | 7.5 | 0.055 | 42.0 | 218.0 | 0.99652 | 3.37 | 0.54 | 10.3 | 0 | 0.004135448485612869 |
7.4 | 0.35 | 0.33 | 2.4 | 0.068 | 9.0 | 26.0 | 0.9947 | 3.36 | 0.6 | 11.9 | 1 | 0.02276768907904625 |
6.9 | 0.22 | 0.31 | 6.3 | 0.029 | 41.0 | 131.0 | 0.99326 | 3.08 | 0.49 | 10.8 | 0 | 0.032101958990097046 |
8.1 | 0.575 | 0.22 | 2.1 | 0.077 | 12.0 | 65.0 | 0.9967 | 3.29 | 0.51 | 9.2 | 1 | 3.2956121140159667E-4 |
6.7 | 0.24 | 0.36 | 8.4 | 0.042 | 42.0 | 123.0 | 0.99473 | 3.34 | 0.52 | 10.9 | 0 | 0.022492729127407074 |
5.8 | 0.31 | 0.32 | 4.5 | 0.024 | 28.0 | 94.0 | 0.98906 | 3.25 | 0.52 | 13.7 | 0 | 0.963473379611969 |
6.4 | 0.24 | 0.26 | 8.2 | 0.054 | 47.0 | 182.0 | 0.99538 | 3.12 | 0.5 | 9.5 | 0 | 6.619771593250334E-4 |
6.8 | 0.25 | 0.24 | 1.6 | 0.045 | 39.0 | 164.0 | 0.99402 | 3.53 | 0.58 | 10.8 | 0 | 0.01979026384651661 |
8.9 | 0.33 | 0.34 | 1.4 | 0.056 | 14.0 | 171.0 | 0.9946 | 3.13 | 0.47 | 9.7 | 0 | 0.0016350742662325501 |
6.8 | 0.73 | 0.2 | 6.6 | 0.054 | 25.0 | 65.0 | 0.99324 | 3.12 | 0.28 | 11.1 | 0 | 0.0011171246878802776 |
7.3 | 0.2 | 0.39 | 2.3 | 0.048 | 24.0 | 87.0 | 0.99044 | 2.94 | 0.35 | 12.0 | 0 | 0.028567757457494736 |
6.4 | 0.3 | 0.33 | 5.2 | 0.05 | 30.0 | 137.0 | 0.99304 | 3.26 | 0.58 | 11.1 | 0 | 0.020369866862893105 |
8.0 | 0.62 | 0.35 | 2.8 | 0.086 | 28.0 | 52.0 | 0.997 | 3.31 | 0.62 | 10.8 | 1 | 0.003430728567764163 |
7.5 | 0.17 | 0.32 | 1.7 | 0.04 | 51.0 | 148.0 | 0.9916 | 3.21 | 0.44 | 11.5 | 0 | 0.8796462416648865 |
5.6 | 0.175 | 0.29 | 0.8 | 0.043 | 20.0 | 67.0 | 0.99112 | 3.28 | 0.48 | 9.9 | 0 | 0.003872503759339452 |
4.4 | 0.46 | 0.1 | 2.8 | 0.024 | 31.0 | 111.0 | 0.98816 | 3.48 | 0.34 | 13.1 | 0 | 0.13440218567848206 |
7.5 | 0.3 | 0.32 | 1.4 | 0.032 | 31.0 | 161.0 | 0.99154 | 2.95 | 0.42 | 10.5 | 0 | 0.006134617142379284 |
6.3 | 0.26 | 0.42 | 7.1 | 0.045 | 62.0 | 209.0 | 0.99544 | 3.2 | 0.53 | 9.5 | 0 | 1.783178886398673E-4 |
6.0 | 0.31 | 0.24 | 3.3 | 0.041 | 25.0 | 143.0 | 0.9914 | 3.31 | 0.44 | 11.3 | 0 | 0.041554518043994904 |
11.6 | 0.47 | 0.44 | 1.6 | 0.147 | 36.0 | 51.0 | 0.99836 | 3.38 | 0.86 | 9.9 | 1 | 0.02042556181550026 |
6.7 | 0.35 | 0.48 | 8.8 | 0.056 | 35.0 | 167.0 | 0.99628 | 3.04 | 0.47 | 9.4 | 0 | 5.436002393253148E-4 |
9.0 | 0.62 | 0.04 | 1.9 | 0.146 | 27.0 | 90.0 | 0.9984 | 3.16 | 0.7 | 9.4 | 1 | 2.608247450552881E-4 |
6.5 | 0.23 | 0.25 | 17.3 | 0.046 | 15.0 | 110.0 | 0.99828 | 3.15 | 0.42 | 9.2 | 0 | 2.588152710814029E-4 |
9.8 | 0.36 | 0.46 | 10.5 | 0.038 | 4.0 | 83.0 | 0.9956 | 2.89 | 0.3 | 10.1 | 0 | 0.0014501246623694897 |
7.9 | 0.35 | 0.46 | 3.6 | 0.078 | 15.0 | 37.0 | 0.9973 | 3.35 | 0.86 | 12.8 | 1 | 0.9709089994430542 |
7.1 | 0.24 | 0.41 | 17.8 | 0.046 | 39.0 | 145.0 | 0.9998 | 3.32 | 0.39 | 8.7 | 0 | 0.00956463348120451 |
5.9 | 0.3 | 0.29 | 1.1 | 0.036 | 23.0 | 56.0 | 0.9904 | 3.19 | 0.38 | 11.3 | 0 | 0.010640747845172882 |
6.7 | 0.7 | 0.08 | 3.75 | 0.067 | 8.0 | 16.0 | 0.99334 | 3.43 | 0.52 | 12.6 | 1 | 0.01553721446543932 |
6.4 | 0.57 | 0.12 | 2.3 | 0.12 | 25.0 | 36.0 | 0.99519 | 3.47 | 0.71 | 11.3 | 1 | 0.864683985710144 |
6.0 | 0.26 | 0.29 | 3.1 | 0.041 | 37.0 | 144.0 | 0.98944 | 3.22 | 0.39 | 12.8 | 0 | 0.9682543277740479 |
6.9 | 0.17 | 0.22 | 4.6 | 0.064 | 55.0 | 152.0 | 0.9952 | 3.29 | 0.37 | 9.3 | 0 | 0.0021151809487491846 |
5.6 | 0.35 | 0.37 | 1.0 | 0.038 | 6.0 | 72.0 | 0.9902 | 3.37 | 0.34 | 11.4 | 0 | 0.014635407365858555 |
5.3 | 0.16 | 0.39 | 1.0 | 0.028 | 40.0 | 101.0 | 0.99156 | 3.57 | 0.59 | 10.6 | 0 | 0.011618298478424549 |
6.2 | 0.31 | 0.23 | 3.3 | 0.052 | 34.0 | 113.0 | 0.99429 | 3.16 | 0.48 | 8.4 | 0 | 0.002168501727283001 |
7.0 | 0.28 | 0.39 | 8.7 | 0.051 | 32.0 | 141.0 | 0.9961 | 3.38 | 0.53 | 10.5 | 0 | 0.02191106043756008 |
7.4 | 0.64 | 0.17 | 5.4 | 0.168 | 52.0 | 98.0 | 0.99736 | 3.28 | 0.5 | 9.5 | 1 | 6.664164247922599E-4 |
8.0 | 0.58 | 0.16 | 2.0 | 0.12 | 3.0 | 7.0 | 0.99454 | 3.22 | 0.58 | 11.2 | 1 | 0.02530890516936779 |
6.1 | 0.38 | 0.15 | 1.8 | 0.072 | 6.0 | 19.0 | 0.9955 | 3.42 | 0.57 | 9.4 | 1 | 0.0019624310079962015 |
6.5 | 0.39 | 0.81 | 1.2 | 0.217 | 14.0 | 74.0 | 0.9936 | 3.08 | 0.53 | 9.5 | 0 | 5.412403261289E-4 |
8.2 | 0.52 | 0.34 | 1.2 | 0.042 | 18.0 | 167.0 | 0.99366 | 3.24 | 0.39 | 10.6 | 0 | 0.0017203710740432143 |
6.2 | 0.23 | 0.36 | 17.2 | 0.039 | 37.0 | 130.0 | 0.99946 | 3.23 | 0.43 | 8.8 | 0 | 7.341491291299462E-4 |
8.4 | 0.22 | 0.28 | 18.8 | 0.028 | 55.0 | 130.0 | 0.998 | 2.96 | 0.35 | 11.6 | 0 | 0.005639814306050539 |
7.0 | 0.48 | 0.12 | 4.5 | 0.05 | 23.0 | 86.0 | 0.99398 | 2.86 | 0.35 | 9.0 | 0 | 7.160695968195796E-4 |
7.7 | 0.34 | 0.28 | 11.0 | 0.04 | 31.0 | 117.0 | 0.99815 | 3.27 | 0.29 | 9.2 | 0 | 0.0035020422656089067 |
9.9 | 0.54 | 0.45 | 2.3 | 0.071 | 16.0 | 40.0 | 0.9991 | 3.39 | 0.62 | 9.4 | 1 | 0.003385338233783841 |
6.3 | 0.27 | 0.37 | 7.9 | 0.047 | 58.0 | 215.0 | 0.99542 | 3.19 | 0.48 | 9.5 | 0 | 2.2263465507421643E-4 |
9.6 | 0.29 | 0.46 | 1.45 | 0.039 | 77.5 | 223.0 | 0.9944 | 2.92 | 0.46 | 9.5 | 0 | 0.0017145829042419791 |
9.4 | 0.16 | 0.23 | 1.6 | 0.042 | 14.0 | 67.0 | 0.9942 | 3.07 | 0.32 | 9.5 | 0 | 0.005379735957831144 |
6.8 | 0.38 | 0.29 | 9.9 | 0.037 | 40.0 | 146.0 | 0.99326 | 3.11 | 0.37 | 11.5 | 0 | 0.025041529908776283 |
6.0 | 0.28 | 0.29 | 19.3 | 0.051 | 36.0 | 174.0 | 0.99911 | 3.14 | 0.5 | 9.0 | 0 | 6.508899969048798E-4 |
6.3 | 0.24 | 0.29 | 1.6 | 0.052 | 48.0 | 185.0 | 0.9934 | 3.21 | 0.5 | 9.4 | 0 | 0.005372313316911459 |
5.6 | 0.205 | 0.16 | 12.55 | 0.051 | 31.0 | 115.0 | 0.99564 | 3.4 | 0.38 | 10.8 | 0 | 0.008476578630506992 |
8.1 | 0.36 | 0.59 | 13.6 | 0.051 | 60.0 | 134.0 | 0.99886 | 2.96 | 0.39 | 8.7 | 0 | 0.0026909206062555313 |
6.6 | 0.22 | 0.37 | 15.4 | 0.035 | 62.0 | 153.0 | 0.99845 | 3.02 | 0.4 | 9.3 | 0 | 0.0029015750624239445 |
7.7 | 0.29 | 0.48 | 2.3 | 0.049 | 36.0 | 178.0 | 0.9931 | 3.17 | 0.64 | 10.6 | 0 | 0.015605042688548565 |
6.5 | 0.21 | 0.37 | 2.5 | 0.048 | 70.0 | 138.0 | 0.9917 | 3.33 | 0.75 | 11.4 | 0 | 0.9725679755210876 |
6.8 | 0.59 | 0.06 | 6.0 | 0.06 | 11.0 | 18.0 | 0.9962 | 3.41 | 0.59 | 10.8 | 1 | 0.9296406507492065 |
6.6 | 0.16 | 0.4 | 1.5 | 0.044 | 48.0 | 143.0 | 0.9912 | 3.54 | 0.52 | 12.4 | 0 | 0.9460657835006714 |
7.6 | 0.39 | 0.32 | 3.6 | 0.035 | 22.0 | 93.0 | 0.99144 | 3.08 | 0.6 | 12.5 | 0 | 0.9876891374588013 |
6.5 | 0.24 | 0.38 | 1.0 | 0.027 | 31.0 | 90.0 | 0.98926 | 3.24 | 0.36 | 12.3 | 0 | 0.023763230070471764 |
9.8 | 0.44 | 0.47 | 2.5 | 0.063 | 9.0 | 28.0 | 0.9981 | 3.24 | 0.65 | 10.8 | 1 | 0.013574243523180485 |
7.1 | 0.31 | 0.5 | 14.5 | 0.059 | 6.0 | 148.0 | 0.9983 | 2.94 | 0.44 | 9.1 | 0 | 0.01131429336965084 |
6.8 | 0.32 | 0.28 | 4.8 | 0.034 | 25.0 | 100.0 | 0.99026 | 3.08 | 0.47 | 12.4 | 0 | 0.9881150722503662 |
6.2 | 0.56 | 0.09 | 1.7 | 0.053 | 24.0 | 32.0 | 0.99402 | 3.54 | 0.6 | 11.3 | 1 | 0.010193511843681335 |
7.7 | 0.46 | 0.18 | 3.3 | 0.054 | 18.0 | 143.0 | 0.99392 | 3.12 | 0.51 | 10.8 | 0 | 0.001233598799444735 |
8.2 | 0.29 | 0.33 | 9.1 | 0.036 | 28.0 | 118.0 | 0.9953 | 2.96 | 0.4 | 10.9 | 0 | 0.8690248131752014 |
10.6 | 1.02 | 0.43 | 2.9 | 0.076 | 26.0 | 88.0 | 0.9984 | 3.08 | 0.57 | 10.1 | 1 | 9.273840696550906E-4 |
6.2 | 0.36 | 0.26 | 13.2 | 0.051 | 54.0 | 201.0 | 0.9976 | 3.25 | 0.46 | 9.0 | 0 | 0.0022995457984507084 |
7.6 | 0.48 | 0.28 | 10.4 | 0.049 | 57.0 | 205.0 | 0.99748 | 3.24 | 0.45 | 9.3 | 0 | 0.0018195603042840958 |
5.8 | 0.28 | 0.34 | 4.0 | 0.031 | 40.0 | 99.0 | 0.9896 | 3.39 | 0.39 | 12.8 | 0 | 0.9969178438186646 |
7.5 | 0.4 | 1.0 | 19.5 | 0.041 | 33.0 | 148.0 | 0.9977 | 3.24 | 0.38 | 12.0 | 0 | 0.022350231185555458 |
8.7 | 0.23 | 0.32 | 13.4 | 0.044 | 35.0 | 169.0 | 0.99975 | 3.12 | 0.47 | 8.8 | 0 | 0.9608659744262695 |
7.0 | 0.27 | 0.48 | 6.1 | 0.042 | 60.0 | 184.0 | 0.99566 | 3.2 | 0.5 | 9.4 | 0 | 5.375688779167831E-4 |
6.6 | 0.39 | 0.39 | 11.9 | 0.057 | 51.0 | 221.0 | 0.99851 | 3.26 | 0.51 | 8.9 | 0 | 0.0038905905093997717 |
7.8 | 0.56 | 0.19 | 1.8 | 0.104 | 12.0 | 47.0 | 0.9964 | 3.19 | 0.93 | 9.5 | 1 | 0.004085764288902283 |
6.3 | 0.18 | 0.24 | 3.4 | 0.053 | 20.0 | 119.0 | 0.99373 | 3.11 | 0.52 | 9.2 | 0 | 0.0029702712781727314 |
9.0 | 0.38 | 0.53 | 2.1 | 0.102 | 19.0 | 76.0 | 0.99001 | 2.93 | 0.57 | 12.9 | 0 | 0.0186296459287405 |
6.6 | 0.35 | 0.29 | 14.4 | 0.044 | 54.0 | 177.0 | 0.9991 | 3.17 | 0.58 | 8.9 | 0 | 0.023067444562911987 |
6.4 | 0.38 | 0.14 | 2.2 | 0.038 | 15.0 | 25.0 | 0.99514 | 3.44 | 0.65 | 11.1 | 1 | 0.017073726281523705 |
6.2 | 0.21 | 0.24 | 1.2 | 0.051 | 31.0 | 95.0 | 0.99036 | 3.24 | 0.57 | 11.3 | 0 | 0.004966433160007 |
6.8 | 0.26 | 0.34 | 15.1 | 0.06 | 42.0 | 162.0 | 0.99705 | 3.24 | 0.52 | 10.5 | 0 | 0.008718721568584442 |
8.8 | 0.6 | 0.29 | 2.2 | 0.098 | 5.0 | 15.0 | 0.9988 | 3.36 | 0.49 | 9.1 | 1 | 0.002779798349365592 |
6.7 | 0.32 | 0.44 | 2.4 | 0.061 | 24.0 | 34.0 | 0.99484 | 3.29 | 0.8 | 11.6 | 1 | 0.9650216698646545 |
6.2 | 0.32 | 0.12 | 4.8 | 0.054 | 6.0 | 97.0 | 0.99424 | 3.16 | 0.5 | 9.3 | 0 | 2.3972481722012162E-4 |
7.15 | 0.17 | 0.24 | 9.6 | 0.119 | 56.0 | 178.0 | 0.99578 | 3.15 | 0.44 | 10.2 | 0 | 0.0022230050526559353 |
6.3 | 0.27 | 0.37 | 7.9 | 0.047 | 58.0 | 215.0 | 0.99542 | 3.19 | 0.48 | 9.5 | 0 | 2.2263465507421643E-4 |
6.6 | 0.27 | 0.31 | 5.3 | 0.137 | 35.0 | 163.0 | 0.9951 | 3.2 | 0.38 | 9.3 | 0 | 0.0015957632567733526 |
7.4 | 0.3 | 0.49 | 8.2 | 0.055 | 49.0 | 188.0 | 0.9974 | 3.52 | 0.58 | 9.7 | 0 | 0.004973367787897587 |
7.5 | 0.29 | 0.67 | 8.1 | 0.037 | 53.0 | 166.0 | 0.9966 | 2.9 | 0.41 | 8.9 | 0 | 3.658780187834054E-4 |
7.3 | 0.55 | 0.03 | 1.6 | 0.072 | 17.0 | 42.0 | 0.9956 | 3.37 | 0.48 | 9.0 | 1 | 0.0030912242364138365 |
8.2 | 0.18 | 0.38 | 1.1 | 0.04 | 41.0 | 92.0 | 0.99062 | 2.88 | 0.6 | 12.0 | 0 | 0.029999202117323875 |
6.9 | 0.4 | 0.24 | 2.5 | 0.083 | 30.0 | 45.0 | 0.9959 | 3.26 | 0.58 | 10.0 | 1 | 0.002721367171034217 |
7.0 | 0.26 | 0.26 | 10.8 | 0.039 | 37.0 | 184.0 | 0.99787 | 3.47 | 0.58 | 10.3 | 0 | 0.8637649416923523 |
7.8 | 0.4 | 0.26 | 9.5 | 0.059 | 32.0 | 178.0 | 0.9955 | 3.04 | 0.43 | 10.9 | 0 | 0.0022082061041146517 |
6.4 | 0.15 | 0.44 | 1.2 | 0.043 | 67.0 | 150.0 | 0.9907 | 3.14 | 0.73 | 11.2 | 0 | 0.9742897152900696 |
7.4 | 0.24 | 0.42 | 14.0 | 0.066 | 48.0 | 198.0 | 0.9979 | 2.89 | 0.42 | 8.9 | 0 | 0.016539830714464188 |
9.2 | 0.23 | 0.35 | 10.7 | 0.037 | 34.0 | 145.0 | 0.9981 | 3.09 | 0.32 | 9.7 | 0 | 0.005976762156933546 |
8.0 | 0.57 | 0.39 | 3.9 | 0.034 | 22.0 | 122.0 | 0.9917 | 3.29 | 0.67 | 12.8 | 0 | 0.9658230543136597 |
7.1 | 0.18 | 0.26 | 1.3 | 0.041 | 20.0 | 71.0 | 0.9926 | 3.04 | 0.74 | 9.9 | 0 | 0.05246594175696373 |
8.1 | 0.38 | 0.48 | 1.8 | 0.157 | 5.0 | 17.0 | 0.9976 | 3.3 | 1.05 | 9.4 | 1 | 0.0017709688981994987 |
7.1 | 0.21 | 0.27 | 8.6 | 0.056 | 26.0 | 111.0 | 0.9956 | 2.95 | 0.52 | 9.5 | 0 | 0.006467135157436132 |
7.1 | 0.18 | 0.39 | 14.5 | 0.051 | 48.0 | 156.0 | 0.99947 | 3.35 | 0.78 | 9.1 | 0 | 0.018350129947066307 |
5.7 | 0.36 | 0.21 | 6.7 | 0.038 | 51.0 | 166.0 | 0.9941 | 3.29 | 0.63 | 10.0 | 0 | 0.0054717520251870155 |
7.0 | 0.65 | 0.02 | 2.1 | 0.066 | 8.0 | 25.0 | 0.9972 | 3.47 | 0.67 | 9.5 | 1 | 0.0034150697756558657 |
6.9 | 0.4 | 0.14 | 2.4 | 0.085 | 21.0 | 40.0 | 0.9968 | 3.43 | 0.63 | 9.7 | 1 | 0.008706411346793175 |
7.5 | 0.32 | 0.24 | 4.6 | 0.053 | 8.0 | 134.0 | 0.9958 | 3.14 | 0.5 | 9.1 | 0 | 0.001309470389969647 |
7.0 | 0.3 | 0.38 | 14.9 | 0.032 | 60.0 | 181.0 | 0.9983 | 3.18 | 0.61 | 9.3 | 0 | 0.8119427561759949 |
6.7 | 0.13 | 0.57 | 6.6 | 0.056 | 60.0 | 150.0 | 0.99548 | 2.96 | 0.43 | 9.4 | 0 | 0.00878129992634058 |
8.0 | 0.28 | 0.42 | 7.1 | 0.045 | 41.0 | 169.0 | 0.9959 | 3.17 | 0.43 | 10.6 | 0 | 0.01727435179054737 |
7.3 | 0.19 | 0.27 | 13.9 | 0.057 | 45.0 | 155.0 | 0.99807 | 2.94 | 0.41 | 8.8 | 0 | 0.9880402684211731 |
5.7 | 0.28 | 0.28 | 2.2 | 0.019 | 15.0 | 65.0 | 0.9902 | 3.06 | 0.52 | 11.2 | 0 | 0.02454240247607231 |
7.5 | 0.57 | 0.02 | 2.6 | 0.077 | 11.0 | 35.0 | 0.99557 | 3.36 | 0.62 | 10.8 | 1 | 0.01031325850635767 |
6.6 | 0.55 | 0.01 | 2.7 | 0.034 | 56.0 | 122.0 | 0.9906 | 3.15 | 0.3 | 11.9 | 0 | 0.04059310257434845 |
7.6 | 0.39 | 0.31 | 2.3 | 0.082 | 23.0 | 71.0 | 0.9982 | 3.52 | 0.65 | 9.7 | 1 | 5.710021359845996E-4 |
6.6 | 0.28 | 0.42 | 8.2 | 0.044 | 60.0 | 196.0 | 0.99562 | 3.14 | 0.48 | 9.4 | 0 | 3.180474741384387E-4 |
7.9 | 0.44 | 0.37 | 5.85 | 0.033 | 27.0 | 93.0 | 0.992 | 3.16 | 0.54 | 12.6 | 0 | 0.9942924976348877 |
8.1 | 0.27 | 0.33 | 1.3 | 0.045 | 26.0 | 100.0 | 0.99066 | 2.98 | 0.44 | 12.4 | 0 | 0.01242974866181612 |
6.2 | 0.37 | 0.24 | 6.1 | 0.032 | 19.0 | 86.0 | 0.98934 | 3.04 | 0.26 | 13.4 | 0 | 0.9906991124153137 |
5.9 | 0.17 | 0.29 | 3.1 | 0.03 | 32.0 | 123.0 | 0.98913 | 3.41 | 0.33 | 13.7 | 0 | 0.9808032512664795 |
7.7 | 0.965 | 0.1 | 2.1 | 0.112 | 11.0 | 22.0 | 0.9963 | 3.26 | 0.5 | 9.5 | 1 | 0.0036368677392601967 |
7.0 | 0.5 | 0.14 | 1.8 | 0.078 | 10.0 | 23.0 | 0.99636 | 3.53 | 0.61 | 10.4 | 1 | 0.017075952142477036 |
6.6 | 0.28 | 0.42 | 8.2 | 0.044 | 60.0 | 196.0 | 0.99562 | 3.14 | 0.48 | 9.4 | 0 | 3.180474741384387E-4 |
7.3 | 0.28 | 0.35 | 1.6 | 0.054 | 31.0 | 148.0 | 0.99178 | 3.18 | 0.47 | 10.7 | 0 | 0.008353336714208126 |
6.8 | 0.27 | 0.3 | 13.0 | 0.047 | 69.0 | 160.0 | 0.99705 | 3.16 | 0.5 | 9.6 | 0 | 5.14763465616852E-4 |
6.4 | 0.24 | 0.32 | 14.9 | 0.047 | 54.0 | 162.0 | 0.9968 | 3.28 | 0.5 | 10.2 | 0 | 0.008532842621207237 |
6.8 | 0.32 | 0.37 | 3.4 | 0.023 | 19.0 | 87.0 | 0.9902 | 3.14 | 0.53 | 12.7 | 0 | 0.017300216481089592 |
7.5 | 0.17 | 0.71 | 11.8 | 0.038 | 52.0 | 148.0 | 0.99801 | 3.03 | 0.46 | 8.9 | 0 | 0.009775901213288307 |
7.6 | 0.48 | 0.37 | 1.2 | 0.034 | 5.0 | 57.0 | 0.99256 | 3.05 | 0.54 | 10.4 | 0 | 0.001510436530224979 |
6.0 | 0.43 | 0.34 | 7.6 | 0.045 | 25.0 | 118.0 | 0.99222 | 3.03 | 0.37 | 11.0 | 0 | 0.024521194398403168 |
8.4 | 0.35 | 0.56 | 13.8 | 0.048 | 55.0 | 190.0 | 0.9993 | 3.07 | 0.58 | 9.4 | 0 | 0.0025425157509744167 |
6.1 | 0.32 | 0.25 | 1.7 | 0.034 | 37.0 | 136.0 | 0.992 | 3.47 | 0.5 | 10.8 | 0 | 0.8919641375541687 |
6.7 | 0.24 | 0.33 | 12.3 | 0.046 | 31.0 | 145.0 | 0.9983 | 3.36 | 0.4 | 9.5 | 0 | 0.0023177654948085546 |
6.7 | 0.23 | 0.33 | 1.8 | 0.036 | 23.0 | 96.0 | 0.9925 | 3.32 | 0.4 | 10.8 | 0 | 0.06474670767784119 |
6.3 | 0.27 | 0.25 | 5.8 | 0.038 | 52.0 | 155.0 | 0.995 | 3.28 | 0.38 | 9.4 | 0 | 0.002198766218498349 |
6.9 | 0.21 | 0.81 | 1.1 | 0.137 | 52.0 | 123.0 | 0.9932 | 3.03 | 0.39 | 9.2 | 0 | 0.014252481050789356 |
8.0 | 0.25 | 0.13 | 17.2 | 0.036 | 49.0 | 219.0 | 0.9996 | 2.96 | 0.46 | 9.7 | 0 | 0.003739548148587346 |
5.7 | 0.28 | 0.35 | 1.2 | 0.052 | 39.0 | 141.0 | 0.99108 | 3.44 | 0.69 | 11.3 | 0 | 0.04083186388015747 |
8.3 | 0.66 | 0.15 | 1.9 | 0.079 | 17.0 | 42.0 | 0.9972 | 3.31 | 0.54 | 9.6 | 1 | 3.009929205290973E-4 |
6.6 | 0.17 | 0.3 | 1.1 | 0.031 | 13.0 | 73.0 | 0.99095 | 3.17 | 0.58 | 11.0 | 0 | 0.07146400958299637 |
7.9 | 0.54 | 0.34 | 2.5 | 0.076 | 8.0 | 17.0 | 0.99235 | 3.2 | 0.72 | 13.1 | 1 | 0.9480963945388794 |
8.3 | 0.18 | 0.3 | 1.1 | 0.033 | 20.0 | 57.0 | 0.99109 | 3.02 | 0.51 | 11.0 | 0 | 0.010759877972304821 |
10.9 | 0.53 | 0.49 | 4.6 | 0.118 | 10.0 | 17.0 | 1.0002 | 3.07 | 0.56 | 11.7 | 1 | 0.023601729422807693 |
7.2 | 0.36 | 0.46 | 2.1 | 0.074 | 24.0 | 44.0 | 0.99534 | 3.4 | 0.85 | 11.0 | 1 | 0.9615651965141296 |
10.6 | 0.31 | 0.49 | 2.2 | 0.063 | 18.0 | 40.0 | 0.9976 | 3.14 | 0.51 | 9.8 | 1 | 0.010627307929098606 |
7.3 | 0.22 | 0.4 | 14.75 | 0.042 | 44.5 | 129.5 | 0.9998 | 3.36 | 0.41 | 9.1 | 0 | 0.9496947526931763 |
8.2 | 0.31 | 0.43 | 7.0 | 0.047 | 18.0 | 87.0 | 0.99628 | 3.23 | 0.64 | 10.6 | 0 | 0.009227443486452103 |
6.5 | 0.29 | 0.25 | 10.6 | 0.039 | 32.0 | 120.0 | 0.9962 | 3.31 | 0.34 | 10.1 | 0 | 0.00871208030730486 |
9.1 | 0.22 | 0.24 | 2.1 | 0.078 | 1.0 | 28.0 | 0.999 | 3.41 | 0.87 | 10.3 | 1 | 0.008228311315178871 |
7.2 | 0.23 | 0.19 | 13.7 | 0.052 | 47.0 | 197.0 | 0.99865 | 3.12 | 0.53 | 9.0 | 0 | 0.012363866902887821 |
7.1 | 0.46 | 0.14 | 2.8 | 0.076 | 15.0 | 37.0 | 0.99624 | 3.36 | 0.49 | 10.7 | 1 | 0.0011812784941866994 |
6.9 | 0.52 | 0.25 | 2.6 | 0.081 | 10.0 | 37.0 | 0.99685 | 3.46 | 0.5 | 11.0 | 1 | 0.001487838220782578 |
7.1 | 0.32 | 0.24 | 13.1 | 0.05 | 52.0 | 204.0 | 0.998 | 3.1 | 0.49 | 8.8 | 0 | 0.010533100925385952 |
6.8 | 0.21 | 0.42 | 1.2 | 0.045 | 24.0 | 126.0 | 0.99234 | 3.09 | 0.87 | 10.9 | 0 | 0.026021797209978104 |
8.0 | 0.2 | 0.4 | 5.2 | 0.055 | 41.0 | 167.0 | 0.9953 | 3.18 | 0.4 | 10.6 | 0 | 0.8233959674835205 |
7.4 | 0.18 | 0.3 | 8.8 | 0.064 | 26.0 | 103.0 | 0.9961 | 2.94 | 0.56 | 9.3 | 0 | 0.0026291643735021353 |
6.8 | 0.22 | 0.31 | 6.9 | 0.037 | 33.0 | 121.0 | 0.99176 | 3.02 | 0.39 | 11.9 | 0 | 0.9452503323554993 |
5.0 | 0.2 | 0.4 | 1.9 | 0.015 | 20.0 | 98.0 | 0.9897 | 3.37 | 0.55 | 12.05 | 0 | 0.06474573910236359 |
6.3 | 0.24 | 0.22 | 11.9 | 0.05 | 65.0 | 179.0 | 0.99659 | 3.06 | 0.58 | 9.3 | 0 | 2.728207327891141E-4 |
7.4 | 0.62 | 0.05 | 1.9 | 0.068 | 24.0 | 42.0 | 0.9961 | 3.42 | 0.57 | 11.5 | 1 | 0.0033662687055766582 |
9.6 | 0.68 | 0.24 | 2.2 | 0.087 | 5.0 | 28.0 | 0.9988 | 3.14 | 0.6 | 10.2 | 1 | 0.0024880545679479837 |
6.5 | 0.29 | 0.52 | 7.9 | 0.049 | 35.0 | 192.0 | 0.99551 | 3.16 | 0.51 | 9.5 | 0 | 0.0013684827135875821 |
7.0 | 0.46 | 0.2 | 16.7 | 0.046 | 50.0 | 184.0 | 0.99898 | 3.08 | 0.56 | 9.4 | 0 | 0.0021231318823993206 |
8.4 | 0.65 | 0.6 | 2.1 | 0.112 | 12.0 | 90.0 | 0.9973 | 3.2 | 0.52 | 9.2 | 1 | 4.612051707226783E-4 |
7.8 | 0.39 | 0.26 | 9.9 | 0.059 | 33.0 | 181.0 | 0.9955 | 3.04 | 0.42 | 10.9 | 0 | 0.001875649788416922 |
6.6 | 0.725 | 0.09 | 5.5 | 0.117 | 9.0 | 17.0 | 0.99655 | 3.35 | 0.49 | 10.8 | 1 | 0.019253870472311974 |
5.8 | 0.36 | 0.5 | 1.0 | 0.127 | 63.0 | 178.0 | 0.99212 | 3.1 | 0.45 | 9.7 | 0 | 0.0012200977653265 |
9.3 | 0.36 | 0.39 | 1.5 | 0.08 | 41.0 | 55.0 | 0.99652 | 3.47 | 0.73 | 10.9 | 1 | 0.011752982623875141 |
7.5 | 0.18 | 0.31 | 11.7 | 0.051 | 24.0 | 94.0 | 0.997 | 3.19 | 0.44 | 9.5 | 0 | 0.9037631154060364 |
8.0 | 0.4 | 0.33 | 7.7 | 0.034 | 27.0 | 98.0 | 0.9935 | 3.18 | 0.41 | 12.2 | 0 | 0.9303209185600281 |
6.0 | 0.26 | 0.33 | 4.35 | 0.04 | 15.0 | 80.0 | 0.98934 | 3.29 | 0.5 | 12.7 | 0 | 0.04740304499864578 |
8.8 | 0.55 | 0.04 | 2.2 | 0.119 | 14.0 | 56.0 | 0.9962 | 3.21 | 0.6 | 10.9 | 1 | 6.927406648173928E-4 |
6.4 | 0.29 | 0.3 | 6.5 | 0.209 | 62.0 | 156.0 | 0.99478 | 3.1 | 0.4 | 9.4 | 0 | 5.81351516302675E-4 |
7.3 | 0.13 | 0.31 | 2.3 | 0.054 | 22.0 | 104.0 | 0.9924 | 3.24 | 0.92 | 11.5 | 0 | 0.9371384382247925 |
6.4 | 0.23 | 0.32 | 1.9 | 0.038 | 40.0 | 118.0 | 0.99074 | 3.32 | 0.53 | 11.8 | 0 | 0.9427400231361389 |
7.4 | 0.21 | 0.27 | 1.2 | 0.041 | 27.0 | 99.0 | 0.9927 | 3.19 | 0.33 | 9.8 | 0 | 0.007548855617642403 |
6.8 | 0.26 | 0.42 | 1.7 | 0.049 | 41.0 | 122.0 | 0.993 | 3.47 | 0.48 | 10.5 | 0 | 0.9502912759780884 |
7.0 | 0.29 | 0.49 | 3.8 | 0.047 | 37.0 | 136.0 | 0.9938 | 2.95 | 0.4 | 9.4 | 0 | 3.685999836307019E-4 |
9.4 | 0.28 | 0.3 | 1.6 | 0.045 | 36.0 | 139.0 | 0.99534 | 3.11 | 0.49 | 9.3 | 0 | 4.0723950951360166E-4 |
8.3 | 0.33 | 0.42 | 1.15 | 0.033 | 18.0 | 96.0 | 0.9911 | 3.2 | 0.32 | 12.4 | 0 | 0.04667888209223747 |
5.3 | 0.275 | 0.24 | 7.4 | 0.038 | 28.0 | 114.0 | 0.99313 | 3.38 | 0.51 | 11.0 | 0 | 0.014003646560013294 |
7.2 | 0.22 | 0.35 | 5.5 | 0.054 | 37.0 | 183.0 | 0.99474 | 3.08 | 0.5 | 10.3 | 0 | 0.004665950778871775 |
6.6 | 0.32 | 0.26 | 4.6 | 0.031 | 26.0 | 120.0 | 0.99198 | 3.4 | 0.73 | 12.5 | 0 | 0.9739524722099304 |
6.3 | 0.3 | 0.48 | 1.8 | 0.069 | 18.0 | 61.0 | 0.9959 | 3.44 | 0.78 | 10.3 | 1 | 0.004731116816401482 |
7.8 | 0.44 | 0.28 | 2.7 | 0.1 | 18.0 | 95.0 | 0.9966 | 3.22 | 0.67 | 9.4 | 1 | 4.5351588050834835E-4 |
7.1 | 0.43 | 0.42 | 5.5 | 0.071 | 28.0 | 128.0 | 0.9973 | 3.42 | 0.71 | 10.5 | 1 | 0.007229063659906387 |
5.9 | 0.24 | 0.26 | 12.3 | 0.053 | 34.0 | 134.0 | 0.9972 | 3.34 | 0.45 | 9.5 | 0 | 0.001104721799492836 |
6.6 | 0.44 | 0.15 | 2.1 | 0.076 | 22.0 | 53.0 | 0.9957 | 3.32 | 0.62 | 9.3 | 1 | 3.9928924525156617E-4 |
6.7 | 0.19 | 0.39 | 1.0 | 0.032 | 14.0 | 71.0 | 0.98912 | 3.31 | 0.38 | 13.0 | 0 | 0.9729631543159485 |
6.0 | 0.38 | 0.26 | 3.5 | 0.035 | 38.0 | 111.0 | 0.98872 | 3.18 | 0.47 | 13.6 | 0 | 0.9621039032936096 |
6.8 | 0.5 | 0.11 | 1.5 | 0.075 | 16.0 | 49.0 | 0.99545 | 3.36 | 0.79 | 9.5 | 1 | 0.0022253775969147682 |
7.1 | 0.32 | 0.32 | 11.0 | 0.038 | 16.0 | 66.0 | 0.9937 | 3.24 | 0.4 | 11.5 | 0 | 0.006232038605958223 |
7.8 | 0.4 | 0.49 | 7.8 | 0.06 | 34.0 | 162.0 | 0.9966 | 3.26 | 0.58 | 11.3 | 0 | 0.020947657525539398 |
7.8 | 0.19 | 0.26 | 8.9 | 0.039 | 42.0 | 182.0 | 0.996 | 3.18 | 0.46 | 9.9 | 0 | 0.005275850649923086 |
7.2 | 0.23 | 0.39 | 14.2 | 0.058 | 49.0 | 192.0 | 0.9979 | 2.98 | 0.48 | 9.0 | 0 | 0.8996444940567017 |
7.3 | 0.91 | 0.1 | 1.8 | 0.074 | 20.0 | 56.0 | 0.99672 | 3.35 | 0.56 | 9.2 | 1 | 6.401854916475713E-4 |
7.9 | 0.25 | 0.34 | 11.4 | 0.04 | 53.0 | 202.0 | 0.99708 | 3.11 | 0.57 | 9.6 | 0 | 0.02411791682243347 |
6.7 | 0.24 | 0.46 | 2.2 | 0.033 | 19.0 | 111.0 | 0.99045 | 3.1 | 0.62 | 11.9 | 0 | 0.014920823276042938 |
7.0 | 0.36 | 0.21 | 2.4 | 0.086 | 24.0 | 69.0 | 0.99556 | 3.4 | 0.53 | 10.1 | 1 | 0.0015035341493785381 |
6.7 | 0.5 | 0.36 | 11.5 | 0.096 | 18.0 | 92.0 | 0.99642 | 3.11 | 0.49 | 9.6 | 0 | 4.531489685177803E-4 |
6.8 | 0.25 | 0.3 | 11.8 | 0.043 | 53.0 | 133.0 | 0.99524 | 3.03 | 0.58 | 10.4 | 0 | 0.02692379616200924 |
7.0 | 0.14 | 0.28 | 1.3 | 0.026 | 10.0 | 56.0 | 0.99352 | 3.46 | 0.45 | 9.9 | 0 | 0.021845560520887375 |
7.4 | 0.2 | 0.35 | 2.1 | 0.038 | 30.0 | 116.0 | 0.9949 | 3.49 | 0.77 | 10.3 | 0 | 0.9065589308738708 |
6.6 | 0.7 | 0.08 | 2.6 | 0.106 | 14.0 | 27.0 | 0.99665 | 3.44 | 0.58 | 10.2 | 1 | 0.0031558824703097343 |
6.7 | 0.18 | 0.31 | 10.6 | 0.035 | 42.0 | 143.0 | 0.99572 | 3.08 | 0.49 | 9.8 | 0 | 0.9060518741607666 |
7.5 | 0.38 | 0.33 | 5.0 | 0.045 | 30.0 | 131.0 | 0.9942 | 3.32 | 0.44 | 10.9 | 0 | 0.007515462581068277 |
6.8 | 0.24 | 0.38 | 8.3 | 0.045 | 50.0 | 185.0 | 0.99578 | 3.15 | 0.5 | 9.5 | 0 | 0.0024725408293306828 |
9.2 | 0.25 | 0.34 | 1.2 | 0.026 | 31.0 | 93.0 | 0.9916 | 2.93 | 0.37 | 11.3 | 0 | 0.8599525094032288 |
10.1 | 0.31 | 0.35 | 1.6 | 0.075 | 9.0 | 28.0 | 0.99672 | 3.24 | 0.83 | 11.2 | 1 | 0.9810463786125183 |
6.15 | 0.21 | 0.37 | 3.2 | 0.021 | 20.0 | 80.0 | 0.99076 | 3.39 | 0.47 | 12.0 | 0 | 0.017040716484189034 |
5.0 | 1.04 | 0.24 | 1.6 | 0.05 | 32.0 | 96.0 | 0.9934 | 3.74 | 0.62 | 11.5 | 1 | 0.006129168439656496 |
7.1 | 0.38 | 0.4 | 2.2 | 0.042 | 54.0 | 201.0 | 0.99177 | 3.03 | 0.5 | 11.4 | 0 | 0.00786233227699995 |
6.5 | 0.21 | 0.51 | 17.6 | 0.045 | 34.0 | 125.0 | 0.99966 | 3.2 | 0.47 | 8.8 | 0 | 0.0018898192793130875 |
6.8 | 0.14 | 0.18 | 1.4 | 0.047 | 30.0 | 90.0 | 0.99164 | 3.27 | 0.54 | 11.2 | 0 | 0.007924862205982208 |
7.8 | 0.24 | 0.38 | 2.1 | 0.058 | 14.0 | 167.0 | 0.994 | 3.21 | 0.55 | 9.9 | 0 | 0.0071731554344296455 |
5.5 | 0.17 | 0.23 | 2.9 | 0.039 | 10.0 | 108.0 | 0.99243 | 3.28 | 0.5 | 10.0 | 0 | 0.002263179514557123 |
6.7 | 0.56 | 0.09 | 2.9 | 0.079 | 7.0 | 22.0 | 0.99669 | 3.46 | 0.61 | 10.2 | 1 | 0.00750516215339303 |
7.2 | 0.62 | 0.01 | 2.3 | 0.065 | 8.0 | 46.0 | 0.99332 | 3.32 | 0.51 | 11.8 | 1 | 0.0021854161750525236 |
6.7 | 0.22 | 0.33 | 1.2 | 0.036 | 36.0 | 86.0 | 0.99058 | 3.1 | 0.76 | 11.4 | 0 | 0.07781589031219482 |
9.1 | 0.28 | 0.49 | 2.0 | 0.059 | 10.0 | 112.0 | 0.9958 | 3.15 | 0.46 | 10.1 | 0 | 5.71487529668957E-4 |
6.4 | 0.31 | 0.26 | 13.2 | 0.046 | 57.0 | 205.0 | 0.9975 | 3.17 | 0.41 | 9.6 | 0 | 8.626714698038995E-4 |
5.0 | 0.4 | 0.5 | 4.3 | 0.046 | 29.0 | 80.0 | 0.9902 | 3.49 | 0.66 | 13.6 | 1 | 0.03542355075478554 |
8.0 | 0.17 | 0.29 | 2.4 | 0.029 | 52.0 | 119.0 | 0.98944 | 3.03 | 0.33 | 12.9 | 0 | 0.04263770952820778 |
6.4 | 0.27 | 0.45 | 8.3 | 0.05 | 52.0 | 196.0 | 0.9955 | 3.18 | 0.48 | 9.5 | 0 | 4.413380811456591E-4 |
8.4 | 0.2 | 0.38 | 11.8 | 0.055 | 51.0 | 170.0 | 1.0004 | 3.34 | 0.82 | 8.9 | 0 | 0.0603061318397522 |
7.6 | 0.43 | 0.29 | 2.1 | 0.075 | 19.0 | 66.0 | 0.99718 | 3.4 | 0.64 | 9.5 | 1 | 0.001305708079598844 |
6.4 | 0.31 | 0.28 | 1.5 | 0.037 | 12.0 | 119.0 | 0.9919 | 3.32 | 0.51 | 10.4 | 0 | 0.9369392395019531 |
7.4 | 0.16 | 0.3 | 13.7 | 0.056 | 33.0 | 168.0 | 0.99825 | 2.9 | 0.44 | 8.7 | 0 | 0.9916422367095947 |
7.3 | 0.21 | 0.29 | 1.6 | 0.034 | 29.0 | 118.0 | 0.9917 | 3.3 | 0.5 | 11.0 | 0 | 0.9674010276794434 |
7.1 | 0.75 | 0.01 | 2.2 | 0.059 | 11.0 | 18.0 | 0.99242 | 3.39 | 0.4 | 12.8 | 1 | 0.008807744830846786 |
5.8 | 0.26 | 0.3 | 2.6 | 0.034 | 75.0 | 129.0 | 0.9902 | 3.2 | 0.38 | 11.5 | 0 | 0.03381295129656792 |
5.7 | 0.26 | 0.24 | 17.8 | 0.059 | 23.0 | 124.0 | 0.99773 | 3.3 | 0.5 | 10.1 | 0 | 0.0011875994969159365 |
6.5 | 0.26 | 0.34 | 16.3 | 0.051 | 56.0 | 197.0 | 1.0004 | 3.49 | 0.42 | 9.8 | 0 | 0.006658060941845179 |
5.6 | 0.25 | 0.19 | 2.4 | 0.049 | 42.0 | 166.0 | 0.992 | 3.25 | 0.43 | 10.4 | 0 | 9.421831928193569E-4 |
8.2 | 0.23 | 0.42 | 1.9 | 0.069 | 9.0 | 17.0 | 0.99376 | 3.21 | 0.54 | 12.3 | 1 | 0.018810546025633812 |
5.7 | 0.22 | 0.2 | 16.0 | 0.044 | 41.0 | 113.0 | 0.99862 | 3.22 | 0.46 | 8.9 | 0 | 1.6850148676894605E-4 |
7.6 | 0.36 | 0.49 | 11.3 | 0.046 | 87.0 | 221.0 | 0.9984 | 3.01 | 0.43 | 9.2 | 0 | 6.659964565187693E-4 |
6.2 | 0.46 | 0.17 | 1.6 | 0.073 | 7.0 | 11.0 | 0.99425 | 3.61 | 0.54 | 11.4 | 1 | 0.010520714335143566 |
6.3 | 0.34 | 0.36 | 4.9 | 0.035 | 31.0 | 185.0 | 0.9946 | 3.15 | 0.49 | 9.7 | 0 | 0.0036995364353060722 |
6.9 | 0.21 | 0.24 | 1.8 | 0.021 | 17.0 | 80.0 | 0.98992 | 3.15 | 0.46 | 12.3 | 0 | 0.9125360250473022 |
6.8 | 0.32 | 0.32 | 8.7 | 0.029 | 31.0 | 105.0 | 0.99146 | 3.0 | 0.34 | 12.3 | 0 | 0.9290609359741211 |
6.7 | 0.31 | 0.3 | 2.4 | 0.038 | 30.0 | 83.0 | 0.98867 | 3.09 | 0.36 | 12.8 | 0 | 0.9683154821395874 |
8.2 | 0.37 | 0.64 | 13.9 | 0.043 | 22.0 | 171.0 | 0.99873 | 2.99 | 0.8 | 9.3 | 0 | 0.0013233715435490012 |
6.6 | 0.31 | 0.07 | 1.5 | 0.033 | 55.0 | 144.0 | 0.99208 | 3.16 | 0.42 | 10.0 | 0 | 0.00380527856759727 |
5.2 | 0.645 | 0.0 | 2.15 | 0.08 | 15.0 | 28.0 | 0.99444 | 3.78 | 0.61 | 12.5 | 1 | 0.01047809049487114 |
6.3 | 0.32 | 0.26 | 12.0 | 0.049 | 63.0 | 170.0 | 0.9961 | 3.14 | 0.55 | 9.9 | 0 | 0.001139268046244979 |
6.2 | 0.22 | 0.3 | 12.4 | 0.054 | 108.0 | 152.0 | 0.99728 | 3.1 | 0.47 | 9.5 | 0 | 6.253985338844359E-4 |
7.4 | 0.18 | 0.29 | 1.4 | 0.042 | 34.0 | 101.0 | 0.99384 | 3.54 | 0.6 | 10.5 | 0 | 0.935178816318512 |
5.7 | 0.1 | 0.27 | 1.3 | 0.047 | 21.0 | 100.0 | 0.9928 | 3.27 | 0.46 | 9.5 | 0 | 0.0036183916963636875 |
10.5 | 0.42 | 0.66 | 2.95 | 0.116 | 12.0 | 29.0 | 0.997 | 3.24 | 0.75 | 11.7 | 1 | 0.9490739107131958 |
8.3 | 0.49 | 0.43 | 2.5 | 0.036 | 32.0 | 116.0 | 0.9944 | 3.23 | 0.47 | 10.7 | 0 | 0.00765836238861084 |
6.5 | 0.615 | 0.0 | 1.9 | 0.065 | 9.0 | 18.0 | 0.9972 | 3.46 | 0.65 | 9.2 | 1 | 0.0021201386116445065 |
8.9 | 0.27 | 0.28 | 0.8 | 0.024 | 29.0 | 128.0 | 0.98984 | 3.01 | 0.35 | 12.4 | 0 | 0.008853645995259285 |
6.2 | 0.66 | 0.48 | 1.2 | 0.029 | 29.0 | 75.0 | 0.9892 | 3.33 | 0.39 | 12.8 | 0 | 0.9495501518249512 |
6.1 | 0.68 | 0.52 | 1.4 | 0.037 | 32.0 | 123.0 | 0.99022 | 3.24 | 0.45 | 12.0 | 0 | 0.012203033082187176 |
6.6 | 0.21 | 0.3 | 9.9 | 0.041 | 64.0 | 174.0 | 0.995 | 3.07 | 0.5 | 10.1 | 0 | 0.008280971087515354 |
6.2 | 0.3 | 0.32 | 1.3 | 0.054 | 27.0 | 183.0 | 0.99266 | 3.3 | 0.43 | 10.1 | 0 | 0.0011360796634107828 |
5.7 | 0.21 | 0.25 | 1.1 | 0.035 | 26.0 | 81.0 | 0.9902 | 3.31 | 0.52 | 11.4 | 0 | 0.018155600875616074 |
5.9 | 0.22 | 0.38 | 1.3 | 0.046 | 24.0 | 90.0 | 0.99232 | 3.2 | 0.47 | 10.0 | 0 | 0.002276132581755519 |
7.8 | 0.57 | 0.31 | 1.8 | 0.069 | 26.0 | 120.0 | 0.99625 | 3.29 | 0.53 | 9.3 | 1 | 4.975745105184615E-4 |
7.5 | 0.33 | 0.32 | 11.1 | 0.036 | 25.0 | 119.0 | 0.9962 | 3.15 | 0.34 | 10.5 | 0 | 0.009844166226685047 |
6.6 | 0.24 | 0.22 | 12.3 | 0.051 | 35.0 | 146.0 | 0.99676 | 3.1 | 0.67 | 9.4 | 0 | 3.517625737003982E-4 |
7.3 | 0.24 | 0.34 | 7.5 | 0.048 | 29.0 | 152.0 | 0.9962 | 3.1 | 0.54 | 9.0 | 0 | 0.012147101573646069 |
6.6 | 0.23 | 0.2 | 11.4 | 0.044 | 45.0 | 131.0 | 0.99604 | 2.96 | 0.51 | 9.7 | 0 | 6.16051082033664E-4 |
6.1 | 0.19 | 0.37 | 2.6 | 0.041 | 24.0 | 99.0 | 0.99153 | 3.18 | 0.5 | 10.9 | 0 | 0.024976378306746483 |
6.0 | 0.24 | 0.33 | 2.5 | 0.026 | 31.0 | 85.0 | 0.99014 | 3.13 | 0.5 | 11.3 | 0 | 0.9214284420013428 |
7.4 | 0.26 | 0.43 | 6.0 | 0.022 | 22.0 | 125.0 | 0.9928 | 3.13 | 0.55 | 11.5 | 0 | 0.023354467004537582 |
6.0 | 0.24 | 0.32 | 6.3 | 0.03 | 34.0 | 129.0 | 0.9946 | 3.52 | 0.41 | 10.4 | 0 | 0.046717725694179535 |
7.9 | 0.64 | 0.46 | 10.6 | 0.244 | 33.0 | 227.0 | 0.9983 | 2.87 | 0.74 | 9.1 | 0 | 7.78110115788877E-4 |
8.7 | 0.23 | 0.32 | 13.4 | 0.044 | 35.0 | 169.0 | 0.99975 | 3.12 | 0.47 | 8.8 | 0 | 0.9608659744262695 |
7.2 | 0.4 | 0.49 | 1.1 | 0.048 | 11.0 | 138.0 | 0.9929 | 3.01 | 0.42 | 9.3 | 0 | 5.015135975554585E-4 |
6.9 | 0.32 | 0.16 | 1.4 | 0.051 | 15.0 | 96.0 | 0.994 | 3.22 | 0.38 | 9.5 | 0 | 7.431516423821449E-4 |
6.4 | 0.24 | 0.28 | 11.5 | 0.05 | 34.0 | 163.0 | 0.9969 | 3.31 | 0.45 | 9.5 | 0 | 0.0027132935356348753 |
6.4 | 0.23 | 0.35 | 10.3 | 0.042 | 54.0 | 140.0 | 0.9967 | 3.23 | 0.47 | 9.2 | 0 | 0.0010201091645285487 |
7.4 | 0.26 | 0.43 | 6.0 | 0.022 | 22.0 | 125.0 | 0.9928 | 3.13 | 0.55 | 11.5 | 0 | 0.023354467004537582 |
6.2 | 0.37 | 0.3 | 6.6 | 0.346 | 79.0 | 200.0 | 0.9954 | 3.29 | 0.58 | 9.6 | 0 | 4.5188062358647585E-4 |
8.5 | 0.18 | 0.3 | 1.1 | 0.028 | 34.0 | 95.0 | 0.99272 | 2.83 | 0.36 | 10.0 | 0 | 0.02517513744533062 |
6.8 | 0.27 | 0.29 | 4.6 | 0.046 | 6.0 | 88.0 | 0.99458 | 3.34 | 0.48 | 10.6 | 0 | 0.00712970457971096 |
7.8 | 0.39 | 0.42 | 2.0 | 0.086 | 9.0 | 21.0 | 0.99526 | 3.39 | 0.66 | 11.6 | 1 | 0.03149202838540077 |
6.4 | 0.33 | 0.44 | 8.9 | 0.055 | 52.0 | 164.0 | 0.99488 | 3.1 | 0.48 | 9.6 | 0 | 0.0012533200206235051 |
7.1 | 0.23 | 0.39 | 13.7 | 0.058 | 26.0 | 172.0 | 0.99755 | 2.9 | 0.46 | 9.0 | 0 | 0.07194004207849503 |
9.1 | 0.52 | 0.33 | 1.3 | 0.07 | 9.0 | 30.0 | 0.9978 | 3.24 | 0.6 | 9.3 | 1 | 0.004854309372603893 |
6.6 | 0.34 | 0.28 | 1.3 | 0.035 | 32.0 | 90.0 | 0.9916 | 3.1 | 0.42 | 10.7 | 0 | 0.01742987148463726 |
6.9 | 0.55 | 0.15 | 2.2 | 0.076 | 19.0 | 40.0 | 0.9961 | 3.41 | 0.59 | 10.1 | 1 | 0.0012258371571078897 |
6.3 | 0.22 | 0.22 | 5.6 | 0.039 | 31.0 | 128.0 | 0.99296 | 3.12 | 0.46 | 10.4 | 0 | 0.0041766115464270115 |
5.8 | 0.335 | 0.14 | 5.8 | 0.046 | 49.0 | 197.0 | 0.9937 | 3.3 | 0.71 | 10.3 | 0 | 0.0015507627977058291 |
8.8 | 0.47 | 0.49 | 2.9 | 0.085 | 17.0 | 110.0 | 0.9982 | 3.29 | 0.6 | 9.8 | 1 | 3.8064728141762316E-4 |
6.9 | 0.19 | 0.6 | 4.0 | 0.037 | 6.0 | 122.0 | 0.99255 | 2.92 | 0.59 | 10.4 | 0 | 0.015589033253490925 |
7.5 | 0.34 | 0.28 | 4.0 | 0.028 | 46.0 | 100.0 | 0.98958 | 3.2 | 0.5 | 13.2 | 0 | 0.9940205216407776 |
7.0 | 0.78 | 0.08 | 2.0 | 0.093 | 10.0 | 19.0 | 0.9956 | 3.4 | 0.47 | 10.0 | 1 | 0.006329833529889584 |
7.2 | 0.21 | 1.0 | 1.1 | 0.154 | 46.0 | 114.0 | 0.9931 | 2.95 | 0.43 | 9.2 | 0 | 0.012776268646121025 |
6.8 | 0.23 | 0.3 | 6.95 | 0.044 | 42.0 | 179.0 | 0.9946 | 3.25 | 0.56 | 10.6 | 0 | 0.01236514188349247 |
7.7 | 0.39 | 0.28 | 4.9 | 0.035 | 36.0 | 109.0 | 0.9918 | 3.19 | 0.58 | 12.2 | 0 | 0.9897956252098083 |
5.8 | 0.28 | 0.18 | 1.2 | 0.058 | 7.0 | 108.0 | 0.99288 | 3.23 | 0.58 | 9.55 | 0 | 0.0011342603247612715 |
6.6 | 0.26 | 0.46 | 6.9 | 0.047 | 59.0 | 183.0 | 0.99594 | 3.2 | 0.45 | 9.3 | 0 | 2.7144537307322025E-4 |
6.6 | 0.24 | 0.38 | 8.0 | 0.042 | 56.0 | 187.0 | 0.99577 | 3.21 | 0.46 | 9.2 | 0 | 7.834394928067923E-4 |
6.0 | 0.58 | 0.2 | 2.4 | 0.075 | 15.0 | 50.0 | 0.99467 | 3.58 | 0.67 | 12.5 | 1 | 0.014307222329080105 |
6.1 | 0.21 | 0.19 | 1.4 | 0.046 | 51.0 | 131.0 | 0.99184 | 3.22 | 0.39 | 10.5 | 0 | 0.00313918711617589 |
7.3 | 0.38 | 0.23 | 6.5 | 0.05 | 18.0 | 102.0 | 0.99304 | 3.1 | 0.55 | 11.2 | 0 | 0.007020110264420509 |
7.4 | 0.16 | 0.27 | 15.5 | 0.05 | 25.0 | 135.0 | 0.9984 | 2.9 | 0.43 | 8.7 | 0 | 0.9851395487785339 |
7.1 | 0.21 | 0.37 | 2.4 | 0.026 | 23.0 | 100.0 | 0.9903 | 3.15 | 0.38 | 11.4 | 0 | 0.9626607894897461 |
8.8 | 0.34 | 0.33 | 9.7 | 0.036 | 46.0 | 172.0 | 0.9966 | 3.08 | 0.4 | 10.2 | 0 | 0.002544792601838708 |
6.8 | 0.23 | 0.32 | 1.6 | 0.026 | 43.0 | 147.0 | 0.9904 | 3.29 | 0.54 | 12.5 | 0 | 0.07340606302022934 |
6.0 | 0.34 | 0.29 | 6.1 | 0.046 | 29.0 | 134.0 | 0.99462 | 3.48 | 0.57 | 10.7 | 0 | 0.024154670536518097 |
6.3 | 0.39 | 0.16 | 1.4 | 0.08 | 11.0 | 23.0 | 0.9955 | 3.34 | 0.56 | 9.3 | 1 | 0.0013545292895287275 |
6.5 | 0.24 | 0.32 | 7.6 | 0.038 | 48.0 | 203.0 | 0.9958 | 3.45 | 0.54 | 9.7 | 0 | 0.9296579957008362 |
6.5 | 0.27 | 0.19 | 6.6 | 0.045 | 98.0 | 175.0 | 0.99364 | 3.16 | 0.34 | 10.1 | 0 | 4.97032015118748E-4 |
5.7 | 0.43 | 0.3 | 5.7 | 0.039 | 24.0 | 98.0 | 0.992 | 3.54 | 0.61 | 12.3 | 0 | 0.9812718033790588 |
8.6 | 0.485 | 0.29 | 4.1 | 0.026 | 19.0 | 101.0 | 0.9918 | 3.01 | 0.38 | 12.4 | 0 | 0.02972564287483692 |
6.2 | 0.45 | 0.2 | 1.6 | 0.069 | 3.0 | 15.0 | 0.9958 | 3.41 | 0.56 | 9.2 | 1 | 0.0013649655738845468 |
5.9 | 0.27 | 0.27 | 9.0 | 0.051 | 43.0 | 136.0 | 0.9941 | 3.25 | 0.53 | 10.7 | 0 | 0.008558063767850399 |
7.4 | 0.28 | 0.3 | 5.3 | 0.054 | 44.0 | 161.0 | 0.9941 | 3.12 | 0.48 | 10.3 | 0 | 0.004000405315309763 |
8.2 | 0.37 | 0.36 | 1.0 | 0.034 | 17.0 | 93.0 | 0.9906 | 3.04 | 0.32 | 11.7 | 0 | 0.8422521948814392 |
7.9 | 0.51 | 0.34 | 2.6 | 0.049 | 13.0 | 135.0 | 0.99335 | 3.09 | 0.51 | 10.0 | 0 | 0.0016310199862346053 |
6.2 | 0.34 | 0.3 | 11.1 | 0.047 | 28.0 | 237.0 | 0.9981 | 3.18 | 0.49 | 8.7 | 0 | 0.004044633824378252 |
6.4 | 0.27 | 0.49 | 7.3 | 0.046 | 53.0 | 206.0 | 0.9956 | 3.24 | 0.43 | 9.2 | 0 | 1.6115655307658017E-4 |
7.1 | 0.26 | 0.32 | 14.45 | 0.074 | 29.0 | 107.0 | 0.998 | 2.96 | 0.42 | 9.2 | 0 | 0.004679521080106497 |
7.9 | 0.3 | 0.68 | 8.3 | 0.05 | 37.5 | 278.0 | 0.99316 | 3.01 | 0.51 | 12.3 | 1 | 0.9336342811584473 |
7.2 | 0.685 | 0.21 | 9.5 | 0.07 | 33.0 | 172.0 | 0.9971 | 3.0 | 0.55 | 9.1 | 0 | 3.9451694465242326E-4 |
5.3 | 0.24 | 0.33 | 1.3 | 0.033 | 25.0 | 97.0 | 0.9906 | 3.59 | 0.38 | 11.0 | 0 | 0.9588112235069275 |
6.1 | 0.45 | 0.27 | 0.8 | 0.039 | 13.0 | 82.0 | 0.9927 | 3.23 | 0.32 | 9.5 | 0 | 0.0015129377134144306 |
5.7 | 0.26 | 0.25 | 10.4 | 0.02 | 7.0 | 57.0 | 0.994 | 3.39 | 0.37 | 10.6 | 0 | 0.015607577748596668 |
7.6 | 0.48 | 0.31 | 9.4 | 0.046 | 6.0 | 194.0 | 0.99714 | 3.07 | 0.61 | 9.4 | 0 | 0.0024555192794650793 |
6.1 | 0.38 | 0.14 | 3.9 | 0.06 | 27.0 | 113.0 | 0.99344 | 3.07 | 0.34 | 9.2 | 0 | 0.0020443713292479515 |
6.6 | 0.21 | 0.39 | 2.3 | 0.041 | 31.0 | 102.0 | 0.99221 | 3.22 | 0.58 | 10.9 | 0 | 0.9475135207176208 |
7.5 | 0.29 | 0.26 | 14.95 | 0.067 | 47.0 | 178.0 | 0.99838 | 3.04 | 0.49 | 9.2 | 0 | 0.004083666950464249 |
5.7 | 0.22 | 0.2 | 16.0 | 0.044 | 41.0 | 113.0 | 0.99862 | 3.22 | 0.46 | 8.9 | 0 | 1.6850148676894605E-4 |
7.3 | 0.22 | 0.37 | 14.3 | 0.063 | 48.0 | 191.0 | 0.9978 | 2.89 | 0.38 | 9.0 | 0 | 0.03318009153008461 |
5.6 | 0.19 | 0.47 | 4.5 | 0.03 | 19.0 | 112.0 | 0.9922 | 3.56 | 0.45 | 11.2 | 0 | 0.07054152339696884 |
6.4 | 0.15 | 0.36 | 1.8 | 0.034 | 43.0 | 150.0 | 0.9922 | 3.42 | 0.69 | 11.0 | 0 | 0.9741061925888062 |
6.3 | 0.31 | 0.3 | 10.0 | 0.046 | 49.0 | 212.0 | 0.9962 | 3.74 | 0.55 | 11.9 | 0 | 0.017285112291574478 |
7.6 | 0.16 | 0.44 | 1.4 | 0.043 | 25.0 | 109.0 | 0.9932 | 3.11 | 0.75 | 10.3 | 0 | 0.006467986386269331 |
8.3 | 0.21 | 0.4 | 1.6 | 0.032 | 35.0 | 110.0 | 0.9907 | 3.02 | 0.6 | 12.9 | 0 | 0.9194912910461426 |
6.6 | 0.46 | 0.49 | 7.4 | 0.052 | 19.0 | 184.0 | 0.9956 | 3.11 | 0.38 | 9.0 | 0 | 8.87764326762408E-4 |
7.2 | 0.34 | 0.32 | 2.5 | 0.09 | 43.0 | 113.0 | 0.9966 | 3.32 | 0.79 | 11.1 | 1 | 0.019545776769518852 |
5.6 | 0.35 | 0.14 | 5.0 | 0.046 | 48.0 | 198.0 | 0.9937 | 3.3 | 0.71 | 10.3 | 0 | 0.0010334511753171682 |
5.7 | 0.245 | 0.33 | 1.1 | 0.049 | 28.0 | 150.0 | 0.9927 | 3.13 | 0.42 | 9.3 | 0 | 0.0013438507448881865 |
7.6 | 0.645 | 0.03 | 1.9 | 0.086 | 14.0 | 57.0 | 0.9969 | 3.37 | 0.46 | 10.3 | 1 | 0.00160258321557194 |
7.6 | 0.32 | 0.58 | 16.75 | 0.05 | 43.0 | 163.0 | 0.9999 | 3.15 | 0.54 | 9.2 | 0 | 0.0027730639558285475 |
7.8 | 0.91 | 0.07 | 1.9 | 0.058 | 22.0 | 47.0 | 0.99525 | 3.51 | 0.43 | 10.7 | 1 | 0.005234219133853912 |
7.8 | 0.27 | 0.35 | 1.2 | 0.05 | 36.0 | 140.0 | 0.99138 | 3.09 | 0.45 | 11.2 | 0 | 0.0025023703929036856 |
7.6 | 0.34 | 0.39 | 7.6 | 0.04 | 45.0 | 215.0 | 0.9965 | 3.11 | 0.53 | 9.2 | 0 | 4.82153904158622E-4 |
11.3 | 0.34 | 0.45 | 2.0 | 0.082 | 6.0 | 15.0 | 0.9988 | 2.94 | 0.66 | 9.2 | 1 | 0.0013876904267817736 |
9.4 | 0.615 | 0.28 | 3.2 | 0.087 | 18.0 | 72.0 | 1.0001 | 3.31 | 0.53 | 9.7 | 1 | 0.001132675795815885 |
6.4 | 0.4 | 0.19 | 3.2 | 0.033 | 28.0 | 124.0 | 0.9904 | 3.22 | 0.54 | 12.7 | 0 | 0.9656537175178528 |
6.6 | 0.25 | 0.36 | 8.1 | 0.045 | 54.0 | 180.0 | 0.9958 | 3.08 | 0.42 | 9.2 | 0 | 7.27405771613121E-4 |
6.7 | 0.24 | 0.31 | 2.3 | 0.044 | 37.0 | 113.0 | 0.99013 | 3.29 | 0.46 | 12.9 | 0 | 0.09935010969638824 |
6.4 | 0.18 | 0.32 | 9.6 | 0.052 | 24.0 | 90.0 | 0.9963 | 3.35 | 0.49 | 9.4 | 0 | 0.008384063839912415 |
7.3 | 0.59 | 0.26 | 2.0 | 0.08 | 17.0 | 104.0 | 0.99584 | 3.28 | 0.52 | 9.9 | 1 | 2.5077926693484187E-4 |
6.6 | 0.16 | 0.25 | 9.8 | 0.049 | 59.5 | 137.0 | 0.995 | 3.16 | 0.38 | 10.0 | 0 | 0.0059852697886526585 |
7.5 | 0.58 | 0.14 | 2.2 | 0.077 | 27.0 | 60.0 | 0.9963 | 3.28 | 0.59 | 9.8 | 1 | 4.2573275277391076E-4 |
6.7 | 0.105 | 0.32 | 12.4 | 0.051 | 34.0 | 106.0 | 0.998 | 3.54 | 0.45 | 9.2 | 0 | 0.015666229650378227 |
6.5 | 0.23 | 0.2 | 7.5 | 0.05 | 44.0 | 179.0 | 0.99504 | 3.18 | 0.48 | 9.53333333333333 | 0 | 3.4483542549423873E-4 |
5.7 | 0.16 | 0.26 | 6.3 | 0.043 | 28.0 | 113.0 | 0.9936 | 3.06 | 0.58 | 9.9 | 0 | 0.004363322630524635 |
6.2 | 0.23 | 0.36 | 17.2 | 0.039 | 37.0 | 130.0 | 0.99946 | 3.23 | 0.43 | 8.8 | 0 | 7.341491291299462E-4 |
7.7 | 0.28 | 0.33 | 6.7 | 0.037 | 32.0 | 155.0 | 0.9951 | 3.39 | 0.62 | 10.7 | 0 | 0.9799205660820007 |
6.2 | 0.29 | 0.29 | 5.6 | 0.046 | 35.0 | 178.0 | 0.99313 | 3.25 | 0.51 | 10.5333333333333 | 0 | 0.0017520221881568432 |
7.8 | 0.54 | 0.26 | 2.0 | 0.088 | 23.0 | 48.0 | 0.9981 | 3.41 | 0.74 | 9.2 | 1 | 0.0011539518600329757 |
6.8 | 0.26 | 0.32 | 7.0 | 0.041 | 38.0 | 118.0 | 0.9939 | 3.25 | 0.52 | 10.8 | 0 | 0.011643529869616032 |
5.0 | 0.29 | 0.54 | 5.7 | 0.035 | 54.0 | 155.0 | 0.98976 | 3.27 | 0.34 | 12.9 | 0 | 0.9767929315567017 |
11.2 | 0.4 | 0.5 | 2.0 | 0.099 | 19.0 | 50.0 | 0.99783 | 3.1 | 0.58 | 10.4 | 1 | 0.001838423777371645 |
7.4 | 0.3 | 0.22 | 5.25 | 0.053 | 33.0 | 180.0 | 0.9926 | 3.13 | 0.45 | 11.6 | 0 | 0.009197613224387169 |
6.7 | 0.11 | 0.34 | 8.8 | 0.043 | 41.0 | 113.0 | 0.9962 | 3.42 | 0.4 | 9.3 | 0 | 0.9120542407035828 |
6.3 | 0.17 | 0.42 | 2.8 | 0.028 | 45.0 | 107.0 | 0.9908 | 3.27 | 0.43 | 11.8 | 0 | 0.016938216984272003 |
7.2 | 0.34 | 0.21 | 2.5 | 0.075 | 41.0 | 68.0 | 0.99586 | 3.37 | 0.54 | 10.1 | 1 | 8.081487030722201E-4 |
7.6 | 0.37 | 0.51 | 11.7 | 0.094 | 58.0 | 181.0 | 0.99776 | 2.91 | 0.51 | 9.0 | 0 | 9.672121959738433E-4 |
7.2 | 0.725 | 0.05 | 4.65 | 0.086 | 4.0 | 11.0 | 0.9962 | 3.41 | 0.39 | 10.9 | 1 | 0.002754471730440855 |
6.4 | 0.14 | 0.31 | 1.2 | 0.034 | 53.0 | 138.0 | 0.99084 | 3.38 | 0.35 | 11.5 | 0 | 0.9695730209350586 |
11.4 | 0.26 | 0.44 | 3.6 | 0.071 | 6.0 | 19.0 | 0.9986 | 3.12 | 0.82 | 9.3 | 1 | 0.01638842187821865 |
6.2 | 0.44 | 0.18 | 7.7 | 0.096 | 28.0 | 210.0 | 0.99771 | 3.56 | 0.72 | 9.2 | 0 | 9.682264062575996E-4 |
6.8 | 0.28 | 0.36 | 1.6 | 0.04 | 25.0 | 87.0 | 0.9924 | 3.23 | 0.66 | 10.3 | 0 | 0.04461444169282913 |
6.9 | 0.3 | 0.25 | 3.3 | 0.041 | 26.0 | 124.0 | 0.99428 | 3.18 | 0.5 | 9.3 | 0 | 8.807581034488976E-4 |
7.6 | 0.345 | 0.26 | 1.9 | 0.043 | 15.0 | 134.0 | 0.9936 | 3.08 | 0.38 | 9.5 | 0 | 9.613612783141434E-4 |
12.4 | 0.42 | 0.49 | 4.6 | 0.073 | 19.0 | 43.0 | 0.9978 | 3.02 | 0.61 | 9.5 | 1 | 0.009629831649363041 |
11.1 | 0.31 | 0.53 | 2.2 | 0.06 | 3.0 | 10.0 | 0.99572 | 3.02 | 0.83 | 10.9 | 1 | 0.9384447336196899 |
5.9 | 0.26 | 0.29 | 5.4 | 0.046 | 34.0 | 116.0 | 0.99224 | 3.24 | 0.41 | 11.4 | 0 | 0.0050916592590510845 |
7.5 | 0.24 | 0.62 | 10.6 | 0.045 | 51.0 | 153.0 | 0.99779 | 3.16 | 0.44 | 8.8 | 0 | 0.0036551114171743393 |
8.0 | 0.32 | 0.36 | 4.6 | 0.042 | 56.0 | 178.0 | 0.9928 | 3.29 | 0.47 | 12.0 | 0 | 0.06805989146232605 |
7.1 | 0.2 | 0.37 | 1.5 | 0.049 | 28.0 | 129.0 | 0.99226 | 3.15 | 0.52 | 10.8 | 0 | 0.013825556263327599 |
9.4 | 0.42 | 0.32 | 6.5 | 0.027 | 20.0 | 167.0 | 0.99479 | 3.08 | 0.43 | 10.6 | 0 | 0.006036173552274704 |
5.3 | 0.36 | 0.27 | 6.3 | 0.028 | 40.0 | 132.0 | 0.99186 | 3.37 | 0.4 | 11.6 | 0 | 0.05298036336898804 |
8.8 | 0.46 | 0.45 | 2.6 | 0.065 | 7.0 | 18.0 | 0.9947 | 3.32 | 0.79 | 14.0 | 1 | 0.08603698760271072 |
8.1 | 0.29 | 0.49 | 7.1 | 0.042 | 22.0 | 124.0 | 0.9944 | 3.14 | 0.41 | 10.8 | 0 | 0.015035143122076988 |
7.1 | 0.46 | 0.14 | 2.8 | 0.076 | 15.0 | 37.0 | 0.99624 | 3.36 | 0.49 | 10.7 | 1 | 0.0011812784941866994 |
8.0 | 0.61 | 0.38 | 12.1 | 0.301 | 24.0 | 220.0 | 0.9993 | 2.94 | 0.48 | 9.2 | 0 | 0.001332051120698452 |
6.2 | 0.15 | 0.49 | 0.9 | 0.033 | 17.0 | 51.0 | 0.9932 | 3.3 | 0.7 | 9.4 | 0 | 0.005528271198272705 |
6.2 | 0.27 | 0.32 | 8.8 | 0.047 | 65.0 | 224.0 | 0.9961 | 3.17 | 0.47 | 8.9 | 0 | 2.521506685297936E-4 |
6.9 | 0.31 | 0.33 | 12.7 | 0.038 | 33.0 | 116.0 | 0.9954 | 3.04 | 0.65 | 10.4 | 0 | 0.02999713085591793 |
6.8 | 0.28 | 0.4 | 22.0 | 0.048 | 48.0 | 167.0 | 1.001 | 2.93 | 0.5 | 8.7 | 0 | 0.002993520349264145 |
7.2 | 0.24 | 0.29 | 2.2 | 0.037 | 37.0 | 102.0 | 0.992 | 3.27 | 0.64 | 11.0 | 0 | 0.9888529777526855 |
6.1 | 0.19 | 0.37 | 2.6 | 0.041 | 24.0 | 99.0 | 0.99153 | 3.18 | 0.5 | 10.9 | 0 | 0.024976378306746483 |
5.9 | 0.415 | 0.02 | 0.8 | 0.038 | 22.0 | 63.0 | 0.9932 | 3.36 | 0.36 | 9.3 | 0 | 0.0096383485943079 |
6.3 | 0.26 | 0.25 | 5.2 | 0.046 | 11.0 | 133.0 | 0.99202 | 2.97 | 0.68 | 11.0 | 0 | 0.011365913785994053 |
7.1 | 0.25 | 0.39 | 2.1 | 0.036 | 30.0 | 124.0 | 0.9908 | 3.28 | 0.43 | 12.2 | 0 | 0.9863220453262329 |
8.3 | 0.16 | 0.48 | 1.7 | 0.057 | 31.0 | 98.0 | 0.9943 | 3.15 | 0.41 | 10.3 | 0 | 0.03663511201739311 |
7.6 | 0.41 | 0.14 | 3.0 | 0.087 | 21.0 | 43.0 | 0.9964 | 3.32 | 0.57 | 10.5 | 1 | 0.001902263960801065 |
7.1 | 0.2 | 0.37 | 1.5 | 0.049 | 28.0 | 129.0 | 0.99226 | 3.15 | 0.52 | 10.8 | 0 | 0.013825556263327599 |
7.5 | 0.24 | 0.31 | 13.1 | 0.05 | 26.0 | 180.0 | 0.99884 | 3.05 | 0.53 | 9.1 | 0 | 0.014874984510242939 |
8.4 | 0.56 | 0.04 | 2.0 | 0.082 | 10.0 | 22.0 | 0.9976 | 3.22 | 0.44 | 9.6 | 1 | 0.0016470466507598758 |
6.3 | 0.12 | 0.36 | 2.1 | 0.044 | 47.0 | 146.0 | 0.9914 | 3.27 | 0.74 | 11.4 | 0 | 0.9590189456939697 |
6.1 | 0.24 | 0.26 | 1.7 | 0.033 | 61.0 | 134.0 | 0.9903 | 3.19 | 0.81 | 11.9 | 0 | 0.9659882187843323 |
7.6 | 0.29 | 0.29 | 4.4 | 0.051 | 26.0 | 146.0 | 0.9939 | 3.16 | 0.39 | 10.2 | 0 | 0.00238398858346045 |
6.0 | 0.13 | 0.28 | 5.7 | 0.038 | 56.0 | 189.5 | 0.9948 | 3.59 | 0.43 | 10.6 | 0 | 0.8624364733695984 |
7.4 | 0.29 | 0.48 | 12.8 | 0.037 | 61.5 | 182.0 | 0.99808 | 3.02 | 0.34 | 8.8 | 0 | 6.514625274576247E-4 |
7.5 | 0.71 | 0.0 | 1.6 | 0.092 | 22.0 | 31.0 | 0.99635 | 3.38 | 0.58 | 10.0 | 1 | 0.007304852362722158 |
8.2 | 0.23 | 0.49 | 0.9 | 0.057 | 15.0 | 73.0 | 0.9928 | 3.07 | 0.38 | 10.4 | 0 | 0.007465945091098547 |
6.8 | 0.21 | 0.4 | 6.3 | 0.032 | 40.0 | 121.0 | 0.99214 | 3.18 | 0.53 | 12.0 | 0 | 0.9170844554901123 |
6.4 | 0.28 | 0.29 | 1.6 | 0.052 | 34.0 | 127.0 | 0.9929 | 3.48 | 0.56 | 10.5 | 0 | 0.9730873107910156 |
6.9 | 0.21 | 0.28 | 2.4 | 0.056 | 49.0 | 159.0 | 0.9944 | 3.02 | 0.47 | 8.8 | 0 | 0.9117656946182251 |
7.6 | 0.79 | 0.21 | 2.3 | 0.087 | 21.0 | 68.0 | 0.9955 | 3.12 | 0.44 | 9.2 | 1 | 2.3710432287771255E-4 |
7.5 | 0.42 | 0.14 | 10.7 | 0.046 | 18.0 | 95.0 | 0.9959 | 3.22 | 0.33 | 10.7 | 0 | 0.0016100506763905287 |
10.2 | 0.29 | 0.65 | 2.4 | 0.075 | 6.0 | 17.0 | 0.99565 | 3.22 | 0.63 | 11.8 | 1 | 0.11069446057081223 |
5.1 | 0.165 | 0.22 | 5.7 | 0.047 | 42.0 | 146.0 | 0.9934 | 3.18 | 0.55 | 9.9 | 0 | 5.899682873860002E-4 |
6.4 | 0.17 | 0.34 | 13.4 | 0.044 | 45.0 | 139.0 | 0.99752 | 3.06 | 0.43 | 9.1 | 0 | 0.002509643556550145 |
8.5 | 0.34 | 0.4 | 4.7 | 0.055 | 3.0 | 9.0 | 0.99738 | 3.38 | 0.66 | 11.6 | 1 | 0.9095790386199951 |
7.6 | 0.51 | 0.24 | 2.4 | 0.091 | 8.0 | 38.0 | 0.998 | 3.47 | 0.66 | 9.6 | 1 | 0.0038855932652950287 |
7.5 | 0.19 | 0.4 | 7.1 | 0.056 | 50.0 | 110.0 | 0.9954 | 3.06 | 0.52 | 9.9 | 0 | 0.01821119338274002 |
7.3 | 0.2 | 0.29 | 19.9 | 0.039 | 69.0 | 237.0 | 1.00037 | 3.1 | 0.48 | 9.2 | 0 | 0.006637043785303831 |
6.6 | 0.36 | 0.52 | 10.1 | 0.05 | 29.0 | 140.0 | 0.99628 | 3.07 | 0.4 | 9.4 | 0 | 4.938433412462473E-4 |
8.2 | 0.37 | 0.27 | 1.7 | 0.028 | 10.0 | 59.0 | 0.9923 | 2.97 | 0.48 | 10.4 | 0 | 0.0026636652182787657 |
7.0 | 0.16 | 0.25 | 14.3 | 0.044 | 27.0 | 149.0 | 0.998 | 2.91 | 0.46 | 9.2 | 0 | 0.014094755984842777 |
7.2 | 0.2 | 0.61 | 16.2 | 0.043 | 14.0 | 103.0 | 0.9987 | 3.06 | 0.36 | 9.2 | 0 | 0.001567455125041306 |
8.6 | 0.16 | 0.49 | 7.3 | 0.043 | 9.0 | 63.0 | 0.9953 | 3.13 | 0.59 | 10.5 | 0 | 0.020841235294938087 |
12.7 | 0.6 | 0.49 | 2.8 | 0.075 | 5.0 | 19.0 | 0.9994 | 3.14 | 0.57 | 11.4 | 1 | 0.002538532018661499 |
6.5 | 0.46 | 0.24 | 11.5 | 0.051 | 56.0 | 171.0 | 0.99588 | 3.08 | 0.56 | 9.8 | 0 | 0.001550292712636292 |
6.5 | 0.28 | 0.34 | 4.6 | 0.054 | 22.0 | 130.0 | 0.99193 | 3.2 | 0.46 | 12.0 | 0 | 0.9355559349060059 |
7.0 | 0.43 | 0.02 | 1.9 | 0.08 | 15.0 | 28.0 | 0.99492 | 3.35 | 0.81 | 10.6 | 1 | 0.017208535224199295 |
7.2 | 0.61 | 0.08 | 4.0 | 0.082 | 26.0 | 108.0 | 0.99641 | 3.25 | 0.51 | 9.4 | 1 | 1.4345889212563634E-4 |
6.8 | 0.3 | 0.22 | 6.2 | 0.06 | 41.0 | 190.0 | 0.99858 | 3.18 | 0.51 | 9.2 | 0 | 7.812726544216275E-4 |
7.4 | 0.19 | 0.42 | 6.4 | 0.067 | 39.0 | 212.0 | 0.9958 | 3.3 | 0.33 | 9.6 | 0 | 0.0072531369514763355 |
6.4 | 0.22 | 0.32 | 7.9 | 0.029 | 34.0 | 124.0 | 0.9948 | 3.4 | 0.39 | 10.2 | 0 | 0.0452432781457901 |
5.8 | 0.28 | 0.27 | 2.6 | 0.054 | 30.0 | 156.0 | 0.9914 | 3.53 | 0.42 | 12.4 | 0 | 0.09076899290084839 |
6.1 | 0.38 | 0.42 | 5.0 | 0.016 | 31.0 | 113.0 | 0.99007 | 3.15 | 0.31 | 12.4 | 0 | 0.9475165009498596 |
6.9 | 0.56 | 0.26 | 10.9 | 0.06 | 55.0 | 193.0 | 0.9969 | 3.21 | 0.44 | 9.4 | 0 | 0.001698148320429027 |
5.9 | 0.35 | 0.47 | 2.2 | 0.11 | 14.0 | 138.0 | 0.9932 | 3.09 | 0.5 | 9.1 | 0 | 0.0014739162288606167 |
7.3 | 0.31 | 0.69 | 10.2 | 0.041 | 58.0 | 160.0 | 0.9977 | 3.06 | 0.45 | 8.6 | 0 | 0.001022046897560358 |
6.6 | 0.22 | 0.37 | 1.2 | 0.059 | 45.0 | 199.0 | 0.993 | 3.37 | 0.55 | 10.3 | 0 | 0.8715085387229919 |
12.0 | 0.37 | 0.76 | 4.2 | 0.066 | 7.0 | 38.0 | 1.0004 | 3.22 | 0.6 | 13.0 | 1 | 0.8481268286705017 |
6.8 | 0.18 | 0.24 | 9.8 | 0.058 | 64.0 | 188.0 | 0.9952 | 3.13 | 0.51 | 10.6 | 0 | 0.022946352139115334 |
8.6 | 0.31 | 0.3 | 0.9 | 0.045 | 16.0 | 109.0 | 0.99249 | 2.95 | 0.39 | 10.1 | 0 | 9.836236713454127E-4 |
4.8 | 0.13 | 0.32 | 1.2 | 0.042 | 40.0 | 98.0 | 0.9898 | 3.42 | 0.64 | 11.8 | 0 | 0.9373663067817688 |
7.1 | 0.28 | 0.26 | 1.9 | 0.049 | 12.0 | 86.0 | 0.9934 | 3.15 | 0.38 | 9.4 | 0 | 9.311651811003685E-4 |
7.8 | 0.43 | 0.49 | 13.0 | 0.033 | 37.0 | 158.0 | 0.9955 | 3.14 | 0.35 | 11.3 | 0 | 0.021478353068232536 |
7.8 | 0.64 | 0.0 | 1.9 | 0.072 | 27.0 | 55.0 | 0.9962 | 3.31 | 0.63 | 11.0 | 1 | 0.001040216418914497 |
7.1 | 0.26 | 0.32 | 5.9 | 0.037 | 39.0 | 97.0 | 0.9934 | 3.31 | 0.4 | 11.6 | 0 | 0.06312219053506851 |
6.7 | 0.23 | 0.31 | 2.1 | 0.046 | 30.0 | 96.0 | 0.9926 | 3.33 | 0.64 | 10.7 | 0 | 0.9740280508995056 |
8.2 | 0.24 | 0.34 | 5.1 | 0.062 | 8.0 | 22.0 | 0.9974 | 3.22 | 0.94 | 10.9 | 1 | 0.046776458621025085 |
12.2 | 0.34 | 0.5 | 2.4 | 0.066 | 10.0 | 21.0 | 1.0 | 3.12 | 1.18 | 9.2 | 1 | 0.007674454711377621 |
6.9 | 0.18 | 0.38 | 6.5 | 0.039 | 20.0 | 110.0 | 0.9943 | 3.1 | 0.42 | 10.5 | 0 | 0.05622175708413124 |
5.2 | 0.155 | 0.33 | 1.6 | 0.028 | 13.0 | 59.0 | 0.98975 | 3.3 | 0.84 | 11.9 | 0 | 0.9721608757972717 |
7.4 | 0.33 | 0.44 | 7.6 | 0.05 | 40.0 | 227.0 | 0.99679 | 3.12 | 0.52 | 9.0 | 0 | 0.0015441806754097342 |
10.3 | 0.27 | 0.24 | 2.1 | 0.072 | 15.0 | 33.0 | 0.9956 | 3.22 | 0.66 | 12.8 | 1 | 0.02152394689619541 |
5.6 | 0.13 | 0.27 | 4.8 | 0.028 | 22.0 | 104.0 | 0.9948 | 3.34 | 0.45 | 9.2 | 0 | 0.005749796982854605 |
7.3 | 0.305 | 0.39 | 1.2 | 0.059 | 7.0 | 11.0 | 0.99331 | 3.29 | 0.52 | 11.5 | 1 | 0.045606207102537155 |
6.0 | 0.2 | 0.26 | 6.8 | 0.049 | 22.0 | 93.0 | 0.9928 | 3.15 | 0.42 | 11.0 | 0 | 0.0047393436543643475 |
5.3 | 0.585 | 0.07 | 7.1 | 0.044 | 34.0 | 145.0 | 0.9945 | 3.34 | 0.57 | 9.7 | 0 | 0.001302200835198164 |
6.0 | 0.36 | 0.39 | 3.2 | 0.027 | 20.0 | 125.0 | 0.991 | 3.38 | 0.39 | 11.3 | 0 | 0.9488707780838013 |
6.9 | 0.36 | 0.34 | 4.2 | 0.018 | 57.0 | 119.0 | 0.9898 | 3.28 | 0.36 | 12.7 | 0 | 0.9730829000473022 |
8.5 | 0.16 | 0.35 | 1.6 | 0.039 | 24.0 | 147.0 | 0.9935 | 2.96 | 0.36 | 10.0 | 0 | 0.006338921841233969 |
7.4 | 0.66 | 0.0 | 1.8 | 0.075 | 13.0 | 40.0 | 0.9978 | 3.51 | 0.56 | 9.4 | 1 | 6.981771439313889E-4 |
6.4 | 0.595 | 0.14 | 5.2 | 0.058 | 15.0 | 97.0 | 0.9951 | 3.38 | 0.36 | 9.0 | 0 | 0.0063329413533210754 |
8.9 | 0.12 | 0.45 | 1.8 | 0.075 | 10.0 | 21.0 | 0.99552 | 3.41 | 0.76 | 11.9 | 1 | 0.9847062230110168 |
6.9 | 0.25 | 0.35 | 9.2 | 0.034 | 42.0 | 150.0 | 0.9947 | 3.21 | 0.36 | 11.5 | 0 | 0.04965755715966225 |
6.9 | 0.19 | 0.31 | 19.25 | 0.043 | 38.0 | 167.0 | 0.99954 | 2.93 | 0.52 | 9.1 | 0 | 0.8546333909034729 |
6.7 | 0.3 | 0.74 | 5.0 | 0.038 | 35.0 | 157.0 | 0.9945 | 3.21 | 0.46 | 9.9 | 0 | 0.0026682710740715265 |
7.4 | 0.2 | 0.29 | 1.7 | 0.047 | 16.0 | 100.0 | 0.99243 | 3.28 | 0.45 | 10.6 | 0 | 0.044938284903764725 |
7.6 | 0.31 | 0.29 | 10.5 | 0.04 | 21.0 | 145.0 | 0.9966 | 3.04 | 0.35 | 9.4 | 0 | 4.198297392576933E-4 |
6.8 | 0.68 | 0.21 | 2.1 | 0.07 | 9.0 | 23.0 | 0.99546 | 3.38 | 0.6 | 10.3 | 1 | 0.0018920016009360552 |
6.4 | 0.21 | 0.28 | 5.9 | 0.047 | 29.0 | 101.0 | 0.99278 | 3.15 | 0.4 | 11.0 | 0 | 0.012112402357161045 |
9.7 | 0.53 | 0.6 | 2.0 | 0.039 | 5.0 | 19.0 | 0.99585 | 3.3 | 0.86 | 12.4 | 1 | 0.014325013384222984 |
5.8 | 0.32 | 0.2 | 2.6 | 0.027 | 17.0 | 123.0 | 0.98936 | 3.36 | 0.78 | 13.9 | 0 | 0.97754967212677 |
7.4 | 0.63 | 0.07 | 2.4 | 0.09 | 11.0 | 37.0 | 0.9979 | 3.43 | 0.76 | 9.7 | 1 | 0.013796201907098293 |
7.5 | 0.24 | 0.31 | 13.1 | 0.05 | 26.0 | 180.0 | 0.99884 | 3.05 | 0.53 | 9.1 | 0 | 0.014874984510242939 |
6.8 | 0.26 | 0.22 | 4.8 | 0.041 | 110.0 | 198.0 | 0.99437 | 3.29 | 0.67 | 10.6 | 0 | 0.0033459917176514864 |
7.3 | 0.815 | 0.09 | 11.4 | 0.044 | 45.0 | 204.0 | 0.99713 | 3.15 | 0.46 | 9.0 | 0 | 3.379957051947713E-4 |
6.9 | 0.21 | 0.33 | 1.4 | 0.056 | 35.0 | 136.0 | 0.9938 | 3.63 | 0.78 | 10.3 | 0 | 0.07026474922895432 |
7.2 | 0.695 | 0.13 | 2.0 | 0.076 | 12.0 | 20.0 | 0.99546 | 3.29 | 0.54 | 10.1 | 1 | 0.002621313789859414 |
6.9 | 0.4 | 0.17 | 12.9 | 0.033 | 59.0 | 186.0 | 0.99754 | 3.08 | 0.49 | 9.4 | 0 | 7.646307349205017E-4 |
7.2 | 0.25 | 0.28 | 14.4 | 0.055 | 55.0 | 205.0 | 0.9986 | 3.12 | 0.38 | 9.0 | 0 | 0.9334162473678589 |
6.4 | 0.57 | 0.02 | 1.8 | 0.067 | 4.0 | 11.0 | 0.997 | 3.46 | 0.68 | 9.5 | 1 | 0.005216920282691717 |
10.4 | 0.64 | 0.24 | 2.8 | 0.105 | 29.0 | 53.0 | 0.9998 | 3.24 | 0.67 | 9.9 | 1 | 0.01499571930617094 |
4.9 | 0.47 | 0.17 | 1.9 | 0.035 | 60.0 | 148.0 | 0.98964 | 3.27 | 0.35 | 11.5 | 0 | 0.07255466282367706 |
13.4 | 0.27 | 0.62 | 2.6 | 0.082 | 6.0 | 21.0 | 1.0002 | 3.16 | 0.67 | 9.7 | 1 | 0.0176024679094553 |
7.1 | 0.2 | 0.3 | 0.9 | 0.019 | 4.0 | 28.0 | 0.98931 | 3.2 | 0.36 | 12.0 | 0 | 0.005845004227012396 |
6.3 | 0.21 | 0.4 | 1.7 | 0.031 | 48.0 | 134.0 | 0.9917 | 3.42 | 0.49 | 11.5 | 0 | 0.04867717996239662 |
6.6 | 0.32 | 0.47 | 15.6 | 0.063 | 27.0 | 173.0 | 0.99872 | 3.18 | 0.56 | 9.0 | 0 | 0.001502561615779996 |
6.2 | 0.32 | 0.5 | 6.5 | 0.048 | 61.0 | 186.0 | 0.9948 | 3.19 | 0.45 | 9.6 | 0 | 7.303899037651718E-4 |
6.9 | 0.3 | 0.49 | 7.6 | 0.057 | 25.0 | 156.0 | 0.9962 | 3.43 | 0.63 | 11.0 | 0 | 0.9289548397064209 |
6.7 | 0.2 | 0.42 | 14.0 | 0.038 | 83.0 | 160.0 | 0.9987 | 3.16 | 0.5 | 9.4 | 0 | 0.009214479476213455 |
6.9 | 0.3 | 0.21 | 15.7 | 0.056 | 49.0 | 159.0 | 0.99827 | 3.11 | 0.48 | 9.0 | 0 | 0.0015334871131926775 |
7.9 | 1.04 | 0.05 | 2.2 | 0.084 | 13.0 | 29.0 | 0.9959 | 3.22 | 0.55 | 9.9 | 1 | 8.703300845809281E-4 |
6.5 | 0.27 | 0.19 | 6.6 | 0.045 | 98.0 | 175.0 | 0.99364 | 3.16 | 0.34 | 10.1 | 0 | 4.97032015118748E-4 |
6.5 | 0.22 | 0.31 | 3.9 | 0.046 | 17.0 | 106.0 | 0.99098 | 3.15 | 0.31 | 11.5 | 0 | 0.09971918910741806 |
6.3 | 0.3 | 0.34 | 1.6 | 0.049 | 14.0 | 132.0 | 0.994 | 3.3 | 0.49 | 9.5 | 0 | 0.0018713403260335326 |
6.4 | 0.22 | 0.3 | 11.2 | 0.046 | 53.0 | 149.0 | 0.99479 | 3.21 | 0.34 | 10.8 | 0 | 0.007557184435427189 |
7.6 | 0.23 | 0.34 | 1.6 | 0.043 | 24.0 | 129.0 | 0.99305 | 3.12 | 0.7 | 10.4 | 0 | 0.003143798094242811 |
6.8 | 0.3 | 0.33 | 12.8 | 0.041 | 60.0 | 168.0 | 0.99659 | 3.1 | 0.56 | 9.8 | 0 | 0.00391704635694623 |
7.4 | 0.28 | 0.36 | 1.1 | 0.028 | 42.0 | 105.0 | 0.9893 | 2.99 | 0.39 | 12.4 | 0 | 0.8886852860450745 |
6.2 | 0.235 | 0.34 | 1.9 | 0.036 | 4.0 | 117.0 | 0.99032 | 3.4 | 0.44 | 12.2 | 0 | 0.006451164372265339 |
6.2 | 0.28 | 0.45 | 7.5 | 0.045 | 46.0 | 203.0 | 0.99573 | 3.26 | 0.46 | 9.2 | 0 | 3.5932735772803426E-4 |
6.3 | 0.27 | 0.51 | 7.6 | 0.049 | 35.0 | 200.0 | 0.99548 | 3.16 | 0.54 | 9.4 | 0 | 0.001243714476004243 |
9.6 | 0.41 | 0.37 | 2.3 | 0.091 | 10.0 | 23.0 | 0.99786 | 3.24 | 0.56 | 10.5 | 1 | 0.01991872675716877 |
6.5 | 0.32 | 0.23 | 8.5 | 0.051 | 20.0 | 138.0 | 0.9943 | 3.03 | 0.42 | 10.7 | 0 | 0.0034508677199482918 |
7.1 | 0.17 | 0.38 | 7.4 | 0.052 | 49.0 | 182.0 | 0.9958 | 3.35 | 0.52 | 9.6 | 0 | 0.002275340259075165 |
8.1 | 0.19 | 0.4 | 0.9 | 0.037 | 73.0 | 180.0 | 0.9926 | 3.06 | 0.34 | 10.0 | 0 | 0.011974669992923737 |
7.3 | 0.25 | 0.26 | 7.2 | 0.048 | 52.0 | 207.0 | 0.99587 | 3.12 | 0.37 | 9.2 | 0 | 0.0012751166941598058 |
9.9 | 0.5 | 0.24 | 2.3 | 0.103 | 6.0 | 14.0 | 0.9978 | 3.34 | 0.52 | 10.0 | 1 | 0.00426108855754137 |
9.0 | 0.45 | 0.49 | 2.6 | 0.084 | 21.0 | 75.0 | 0.9987 | 3.35 | 0.57 | 9.7 | 1 | 5.545644671656191E-4 |
6.6 | 0.27 | 0.25 | 1.2 | 0.033 | 36.0 | 111.0 | 0.98918 | 3.16 | 0.37 | 12.4 | 0 | 0.011795049533247948 |
6.8 | 0.19 | 0.58 | 14.2 | 0.038 | 51.0 | 164.0 | 0.9975 | 3.12 | 0.48 | 9.6 | 0 | 0.015204963274300098 |
7.3 | 0.14 | 0.49 | 1.1 | 0.038 | 28.0 | 99.0 | 0.9928 | 3.2 | 0.72 | 10.6 | 0 | 0.05173246189951897 |
7.3 | 0.24 | 0.34 | 15.4 | 0.05 | 38.0 | 174.0 | 0.9983 | 3.03 | 0.42 | 9.0 | 0 | 0.016723770648241043 |
7.8 | 0.445 | 0.56 | 1.0 | 0.04 | 8.0 | 84.0 | 0.9938 | 3.25 | 0.43 | 10.8 | 0 | 0.0016302677104249597 |
5.6 | 0.42 | 0.34 | 2.4 | 0.022 | 34.0 | 97.0 | 0.98915 | 3.22 | 0.38 | 12.8 | 0 | 0.9619507789611816 |
8.7 | 0.42 | 0.45 | 2.4 | 0.072 | 32.0 | 59.0 | 0.99617 | 3.33 | 0.77 | 12.0 | 1 | 0.04215645045042038 |
6.1 | 0.27 | 0.33 | 2.2 | 0.021 | 26.0 | 117.0 | 0.9886 | 3.12 | 0.3 | 12.5 | 0 | 0.015626272186636925 |
6.7 | 0.16 | 0.28 | 2.5 | 0.046 | 40.0 | 153.0 | 0.9921 | 3.38 | 0.51 | 11.4 | 0 | 0.9141088724136353 |
6.0 | 0.31 | 0.27 | 2.3 | 0.042 | 19.0 | 120.0 | 0.98952 | 3.32 | 0.41 | 12.7 | 0 | 0.9181186556816101 |
6.4 | 0.32 | 0.5 | 10.7 | 0.047 | 57.0 | 206.0 | 0.9968 | 3.08 | 0.6 | 9.4 | 0 | 9.42974875215441E-4 |
8.0 | 0.18 | 0.37 | 0.9 | 0.049 | 36.0 | 109.0 | 0.99007 | 2.89 | 0.44 | 12.7 | 1 | 0.004893437493592501 |
7.5 | 0.28 | 0.33 | 7.7 | 0.048 | 42.0 | 180.0 | 0.9974 | 3.37 | 0.59 | 10.1 | 0 | 0.01641991175711155 |
6.5 | 0.21 | 0.4 | 7.3 | 0.041 | 49.0 | 115.0 | 0.99268 | 3.21 | 0.43 | 11.0 | 0 | 0.024358488619327545 |
6.7 | 0.28 | 0.42 | 3.5 | 0.035 | 43.0 | 105.0 | 0.99021 | 3.18 | 0.38 | 12.2 | 0 | 0.0350559838116169 |
8.2 | 0.56 | 0.23 | 3.4 | 0.078 | 14.0 | 104.0 | 0.9976 | 3.28 | 0.62 | 9.4 | 1 | 2.0031406893394887E-4 |
7.0 | 0.16 | 0.3 | 2.6 | 0.043 | 34.0 | 90.0 | 0.99047 | 2.88 | 0.47 | 11.2 | 0 | 0.019490327686071396 |
6.4 | 0.23 | 0.26 | 8.1 | 0.054 | 47.0 | 181.0 | 0.9954 | 3.12 | 0.49 | 9.4 | 0 | 6.293793558143079E-4 |
6.3 | 0.28 | 0.34 | 8.1 | 0.038 | 44.0 | 129.0 | 0.99248 | 3.26 | 0.29 | 12.1 | 0 | 0.10637065023183823 |
5.9 | 0.18 | 0.28 | 1.0 | 0.037 | 24.0 | 88.0 | 0.99094 | 3.29 | 0.55 | 10.65 | 0 | 0.8750344514846802 |
6.8 | 0.21 | 0.27 | 18.15 | 0.042 | 41.0 | 146.0 | 1.0001 | 3.3 | 0.36 | 8.7 | 0 | 0.0066173202358186245 |
6.7 | 0.24 | 0.41 | 2.9 | 0.039 | 48.0 | 122.0 | 0.99052 | 3.25 | 0.43 | 12.0 | 0 | 0.04828253760933876 |
6.3 | 0.13 | 0.42 | 1.1 | 0.043 | 63.0 | 146.0 | 0.99066 | 3.13 | 0.72 | 11.2 | 0 | 0.9880614280700684 |
6.8 | 0.18 | 0.35 | 5.4 | 0.054 | 53.0 | 143.0 | 0.99287 | 3.1 | 0.54 | 11.0 | 0 | 0.9508795738220215 |
7.3 | 0.21 | 0.3 | 10.9 | 0.037 | 18.0 | 112.0 | 0.997 | 3.4 | 0.5 | 9.6 | 0 | 0.040153760462999344 |
6.5 | 0.24 | 0.39 | 17.3 | 0.052 | 22.0 | 126.0 | 0.99888 | 3.11 | 0.47 | 9.2 | 0 | 5.760281346738338E-4 |
6.8 | 0.23 | 0.32 | 8.6 | 0.046 | 47.0 | 159.0 | 0.99452 | 3.08 | 0.52 | 10.5 | 0 | 0.044247664511203766 |
6.8 | 0.34 | 0.69 | 1.3 | 0.058 | 12.0 | 171.0 | 0.9931 | 3.06 | 0.47 | 9.7 | 0 | 7.352356915362179E-4 |
6.5 | 0.27 | 0.4 | 10.0 | 0.039 | 74.0 | 227.0 | 0.99582 | 3.18 | 0.5 | 9.4 | 0 | 6.031348602846265E-4 |
8.0 | 0.24 | 0.26 | 1.7 | 0.033 | 36.0 | 136.0 | 0.99316 | 3.44 | 0.51 | 10.4 | 0 | 0.9060077667236328 |
6.8 | 0.15 | 0.32 | 8.8 | 0.058 | 24.0 | 110.0 | 0.9972 | 3.4 | 0.4 | 8.8 | 0 | 0.10876435041427612 |
6.0 | 0.11 | 0.47 | 10.6 | 0.052 | 69.0 | 148.0 | 0.9958 | 2.91 | 0.34 | 9.3 | 0 | 0.002757488517090678 |
7.2 | 0.17 | 0.41 | 1.6 | 0.052 | 24.0 | 126.0 | 0.99228 | 3.19 | 0.49 | 10.8 | 0 | 0.02206580340862274 |
6.9 | 0.2 | 0.3 | 4.7 | 0.041 | 40.0 | 148.0 | 0.9932 | 3.16 | 0.35 | 10.2 | 0 | 0.0045937043614685535 |
5.9 | 0.17 | 0.28 | 0.7 | 0.027 | 5.0 | 28.0 | 0.98985 | 3.13 | 0.32 | 10.6 | 0 | 0.010227839462459087 |
8.3 | 0.18 | 0.3 | 1.1 | 0.033 | 20.0 | 57.0 | 0.99109 | 3.02 | 0.51 | 11.0 | 0 | 0.010759877972304821 |
7.2 | 0.57 | 0.06 | 1.6 | 0.076 | 9.0 | 27.0 | 0.9972 | 3.36 | 0.7 | 9.6 | 1 | 0.016697796061635017 |
6.6 | 0.25 | 0.51 | 8.0 | 0.047 | 61.0 | 189.0 | 0.99604 | 3.22 | 0.49 | 9.2 | 0 | 7.595156785100698E-4 |
7.3 | 0.4 | 0.24 | 6.7 | 0.058 | 41.0 | 166.0 | 0.995 | 3.2 | 0.41 | 9.9 | 0 | 0.001988715725019574 |
6.8 | 0.28 | 0.29 | 11.9 | 0.052 | 51.0 | 149.0 | 0.99544 | 3.02 | 0.58 | 10.4 | 0 | 0.015471823513507843 |
6.8 | 0.33 | 0.28 | 1.2 | 0.032 | 38.0 | 131.0 | 0.9889 | 3.19 | 0.41 | 13.0 | 0 | 0.0882938802242279 |
9.4 | 0.27 | 0.53 | 2.4 | 0.074 | 6.0 | 18.0 | 0.9962 | 3.2 | 1.13 | 12.0 | 1 | 0.9839180707931519 |
7.7 | 0.28 | 0.24 | 2.4 | 0.044 | 29.0 | 157.0 | 0.99312 | 3.27 | 0.56 | 10.6 | 0 | 0.011209137737751007 |
6.6 | 0.33 | 0.24 | 16.05 | 0.045 | 31.0 | 147.0 | 0.99822 | 3.08 | 0.52 | 9.2 | 0 | 9.001975413411856E-4 |
7.1 | 0.59 | 0.01 | 2.5 | 0.077 | 20.0 | 85.0 | 0.99746 | 3.55 | 0.59 | 9.8 | 1 | 3.5423587542027235E-4 |
6.6 | 0.28 | 0.3 | 7.8 | 0.049 | 57.0 | 202.0 | 0.9958 | 3.24 | 0.39 | 9.5 | 0 | 3.9555973489768803E-4 |
10.8 | 0.45 | 0.33 | 2.5 | 0.099 | 20.0 | 38.0 | 0.99818 | 3.24 | 0.71 | 10.8 | 1 | 0.023227931931614876 |
7.8 | 0.32 | 0.33 | 10.4 | 0.031 | 47.0 | 194.0 | 0.99692 | 3.07 | 0.58 | 9.6 | 0 | 0.012843062169849873 |
6.7 | 0.36 | 0.28 | 8.3 | 0.034 | 29.0 | 81.0 | 0.99151 | 2.96 | 0.39 | 12.5 | 0 | 0.031208785250782967 |
6.7 | 0.26 | 0.26 | 4.0 | 0.079 | 35.5 | 216.0 | 0.9956 | 3.31 | 0.68 | 9.5 | 0 | 0.001825183629989624 |
6.9 | 0.28 | 0.33 | 1.2 | 0.039 | 16.0 | 98.0 | 0.9904 | 3.07 | 0.39 | 11.7 | 0 | 0.013760337606072426 |
6.1 | 0.35 | 0.07 | 1.4 | 0.069 | 22.0 | 108.0 | 0.9934 | 3.23 | 0.52 | 9.2 | 0 | 4.6903439215384424E-4 |
5.9 | 0.24 | 0.12 | 1.4 | 0.035 | 60.0 | 247.0 | 0.99358 | 3.34 | 0.44 | 9.6 | 0 | 0.006284307222813368 |
7.5 | 0.28 | 0.34 | 4.2 | 0.028 | 36.0 | 116.0 | 0.991 | 2.99 | 0.41 | 12.3 | 0 | 0.9395624399185181 |
6.1 | 0.37 | 0.36 | 4.7 | 0.035 | 36.0 | 116.0 | 0.991 | 3.31 | 0.62 | 12.6 | 0 | 0.12990842759609222 |
7.1 | 0.875 | 0.05 | 5.7 | 0.082 | 3.0 | 14.0 | 0.99808 | 3.4 | 0.52 | 10.2 | 1 | 0.005389035679399967 |
7.8 | 0.53 | 0.33 | 2.4 | 0.08 | 24.0 | 144.0 | 0.99655 | 3.3 | 0.6 | 9.5 | 1 | 6.365575245581567E-4 |
7.6 | 0.32 | 0.25 | 9.5 | 0.03 | 15.0 | 136.0 | 0.99367 | 3.1 | 0.44 | 12.1 | 0 | 0.035778727382421494 |
6.4 | 0.24 | 0.49 | 5.8 | 0.053 | 25.0 | 120.0 | 0.9942 | 3.01 | 0.98 | 10.5 | 0 | 0.006541768554598093 |
8.1 | 0.3 | 0.31 | 1.1 | 0.041 | 49.0 | 123.0 | 0.9914 | 2.99 | 0.45 | 11.1 | 0 | 0.004542839247733355 |
6.7 | 0.36 | 0.26 | 7.9 | 0.034 | 39.0 | 123.0 | 0.99119 | 2.99 | 0.3 | 12.2 | 0 | 0.9395993947982788 |
6.6 | 0.36 | 0.52 | 11.3 | 0.046 | 8.0 | 110.0 | 0.9966 | 3.07 | 0.46 | 9.4 | 0 | 3.651140723377466E-4 |
6.3 | 0.27 | 0.46 | 11.75 | 0.037 | 61.0 | 212.0 | 0.9971 | 3.25 | 0.53 | 9.5 | 0 | 0.0023023600224405527 |
8.8 | 0.48 | 0.41 | 3.3 | 0.092 | 26.0 | 52.0 | 0.9982 | 3.31 | 0.53 | 10.5 | 1 | 0.0035963882692158222 |
9.2 | 0.22 | 0.4 | 2.4 | 0.054 | 18.0 | 151.0 | 0.9952 | 3.04 | 0.46 | 9.3 | 0 | 0.0031250014435499907 |
6.7 | 0.45 | 0.3 | 5.3 | 0.036 | 27.0 | 165.0 | 0.99122 | 3.12 | 0.46 | 12.2 | 0 | 0.05843113362789154 |
8.2 | 0.28 | 0.4 | 2.4 | 0.052 | 4.0 | 10.0 | 0.99356 | 3.33 | 0.7 | 12.8 | 1 | 0.9914606809616089 |
5.9 | 0.29 | 0.28 | 3.2 | 0.035 | 16.0 | 117.0 | 0.98959 | 3.26 | 0.42 | 12.6 | 0 | 0.04909363389015198 |
7.2 | 0.17 | 0.34 | 6.4 | 0.042 | 16.0 | 111.0 | 0.99278 | 2.99 | 0.4 | 10.8 | 0 | 0.012028559111058712 |
7.1 | 0.36 | 0.56 | 1.3 | 0.046 | 25.0 | 102.0 | 0.9923 | 3.24 | 0.33 | 10.5 | 0 | 0.003672334598377347 |
9.2 | 0.19 | 0.42 | 2.0 | 0.047 | 16.0 | 104.0 | 0.99517 | 3.09 | 0.66 | 10.0 | 0 | 0.010107862763106823 |
7.8 | 0.2 | 0.24 | 1.6 | 0.026 | 26.0 | 189.0 | 0.991 | 3.08 | 0.74 | 12.1 | 0 | 0.9162977933883667 |
8.2 | 0.42 | 0.49 | 2.6 | 0.084 | 32.0 | 55.0 | 0.9988 | 3.34 | 0.75 | 8.7 | 1 | 5.734075675718486E-4 |
9.9 | 0.54 | 0.45 | 2.3 | 0.071 | 16.0 | 40.0 | 0.9991 | 3.39 | 0.62 | 9.4 | 1 | 0.003385338233783841 |
7.6 | 0.665 | 0.1 | 1.5 | 0.066 | 27.0 | 55.0 | 0.99655 | 3.39 | 0.51 | 9.3 | 1 | 0.001138307387009263 |
6.8 | 0.11 | 0.42 | 1.1 | 0.042 | 51.0 | 132.0 | 0.99059 | 3.18 | 0.74 | 11.3 | 0 | 0.9774789214134216 |
8.3 | 0.6 | 0.13 | 2.6 | 0.085 | 6.0 | 24.0 | 0.9984 | 3.31 | 0.59 | 9.2 | 1 | 5.149683565832675E-4 |
6.4 | 0.16 | 0.28 | 2.2 | 0.042 | 33.0 | 93.0 | 0.9914 | 3.31 | 0.43 | 11.1 | 0 | 0.007065366953611374 |
8.5 | 0.46 | 0.31 | 2.25 | 0.078 | 32.0 | 58.0 | 0.998 | 3.33 | 0.54 | 9.8 | 1 | 7.502899970859289E-4 |
6.2 | 0.16 | 0.32 | 1.1 | 0.036 | 74.0 | 184.0 | 0.99096 | 3.22 | 0.41 | 11.0 | 0 | 0.026236362755298615 |
8.2 | 0.27 | 0.43 | 1.6 | 0.035 | 31.0 | 128.0 | 0.9916 | 3.1 | 0.5 | 12.3 | 0 | 0.025585660710930824 |
6.9 | 0.32 | 0.17 | 7.6 | 0.042 | 69.0 | 219.0 | 0.9959 | 3.13 | 0.4 | 8.9 | 0 | 1.8863857258111238E-4 |
10.4 | 0.44 | 0.42 | 1.5 | 0.145 | 34.0 | 48.0 | 0.99832 | 3.38 | 0.86 | 9.9 | 1 | 0.015392756089568138 |
6.5 | 0.19 | 0.3 | 0.8 | 0.043 | 33.0 | 144.0 | 0.9936 | 3.42 | 0.39 | 9.1 | 0 | 0.026762990280985832 |
6.3 | 0.26 | 0.42 | 7.1 | 0.045 | 62.0 | 209.0 | 0.99544 | 3.2 | 0.53 | 9.5 | 0 | 1.783178886398673E-4 |
6.9 | 0.63 | 0.01 | 2.4 | 0.076 | 14.0 | 39.0 | 0.99522 | 3.34 | 0.53 | 10.8 | 1 | 7.952429004944861E-4 |
6.1 | 0.25 | 0.18 | 10.5 | 0.049 | 41.0 | 124.0 | 0.9963 | 3.14 | 0.35 | 10.5 | 0 | 6.691603921353817E-4 |
6.3 | 0.34 | 0.19 | 5.8 | 0.041 | 22.0 | 145.0 | 0.9943 | 3.15 | 0.63 | 9.9 | 0 | 0.0013379711890593171 |
6.3 | 0.22 | 0.43 | 4.55 | 0.038 | 31.0 | 130.0 | 0.9918 | 3.35 | 0.33 | 11.5 | 0 | 0.9571211338043213 |
7.4 | 0.24 | 0.36 | 2.0 | 0.031 | 27.0 | 139.0 | 0.99055 | 3.28 | 0.48 | 12.5 | 0 | 0.9220747351646423 |
6.0 | 0.33 | 0.2 | 1.8 | 0.031 | 49.0 | 159.0 | 0.9919 | 3.41 | 0.53 | 11.0 | 0 | 0.03653550520539284 |
6.7 | 0.18 | 0.37 | 1.3 | 0.027 | 42.0 | 125.0 | 0.98939 | 3.24 | 0.37 | 12.8 | 0 | 0.9595497250556946 |
9.2 | 0.34 | 0.54 | 17.3 | 0.06 | 46.0 | 235.0 | 1.00182 | 3.08 | 0.61 | 8.8 | 0 | 0.001597694936208427 |
6.2 | 0.43 | 0.49 | 6.4 | 0.045 | 12.0 | 115.0 | 0.9963 | 3.27 | 0.57 | 9.0 | 0 | 0.002896913094446063 |
7.0 | 0.975 | 0.04 | 2.0 | 0.087 | 12.0 | 67.0 | 0.99565 | 3.35 | 0.6 | 9.4 | 1 | 6.062201573513448E-4 |
6.6 | 0.22 | 0.23 | 17.3 | 0.047 | 37.0 | 118.0 | 0.99906 | 3.08 | 0.46 | 8.8 | 0 | 3.668940917123109E-4 |
6.8 | 0.21 | 0.62 | 6.4 | 0.041 | 7.0 | 113.0 | 0.99358 | 2.96 | 0.59 | 10.2 | 0 | 0.013671333901584148 |
6.9 | 0.49 | 0.1 | 2.3 | 0.074 | 12.0 | 30.0 | 0.9959 | 3.42 | 0.58 | 10.2 | 1 | 0.009492073208093643 |
6.4 | 0.31 | 0.53 | 8.8 | 0.057 | 36.0 | 221.0 | 0.99642 | 3.17 | 0.44 | 9.1 | 0 | 5.159618449397385E-4 |
6.6 | 0.29 | 0.44 | 9.0 | 0.053 | 62.0 | 178.0 | 0.99685 | 3.02 | 0.45 | 8.9 | 0 | 9.471956873312593E-4 |
7.5 | 0.42 | 0.45 | 9.1 | 0.029 | 20.0 | 125.0 | 0.996 | 3.12 | 0.36 | 10.1 | 0 | 0.0014206573832780123 |
7.0 | 0.34 | 0.39 | 6.9 | 0.066 | 43.0 | 162.0 | 0.99561 | 3.11 | 0.53 | 9.5 | 0 | 5.500393453985453E-4 |
6.9 | 0.25 | 0.34 | 1.3 | 0.035 | 27.0 | 82.0 | 0.99045 | 3.18 | 0.44 | 12.2 | 0 | 0.01744682528078556 |
9.3 | 0.37 | 0.44 | 1.6 | 0.038 | 21.0 | 42.0 | 0.99526 | 3.24 | 0.81 | 10.8 | 1 | 0.9377866983413696 |
7.9 | 0.29 | 0.39 | 6.7 | 0.036 | 6.0 | 117.0 | 0.9938 | 3.12 | 0.42 | 10.7 | 0 | 0.011774446815252304 |
6.6 | 0.56 | 0.15 | 10.0 | 0.037 | 38.0 | 157.0 | 0.99642 | 3.28 | 0.52 | 9.4 | 0 | 9.750043391250074E-4 |
6.6 | 0.58 | 0.02 | 2.4 | 0.069 | 19.0 | 40.0 | 0.99387 | 3.38 | 0.66 | 12.6 | 1 | 0.08363926410675049 |
6.9 | 0.38 | 0.29 | 13.65 | 0.048 | 52.0 | 189.0 | 0.99784 | 3.0 | 0.6 | 9.5 | 0 | 0.03371163085103035 |
7.1 | 0.13 | 0.38 | 1.8 | 0.046 | 14.0 | 114.0 | 0.9925 | 3.32 | 0.9 | 11.7 | 0 | 0.10363535583019257 |
5.8 | 0.315 | 0.27 | 1.55 | 0.026 | 15.0 | 70.0 | 0.98994 | 3.37 | 0.4 | 11.9 | 0 | 0.8974432945251465 |
6.8 | 0.44 | 0.37 | 5.1 | 0.047 | 46.0 | 201.0 | 0.9938 | 3.08 | 0.65 | 10.5 | 0 | 0.0031450986862182617 |
6.1 | 1.1 | 0.16 | 4.4 | 0.033 | 8.0 | 109.0 | 0.99058 | 3.35 | 0.47 | 12.4 | 0 | 0.01412995159626007 |
6.7 | 0.29 | 0.45 | 14.3 | 0.054 | 30.0 | 181.0 | 0.99869 | 3.14 | 0.57 | 9.1 | 0 | 0.02066357620060444 |
7.0 | 0.54 | 0.0 | 2.1 | 0.079 | 39.0 | 55.0 | 0.9956 | 3.39 | 0.84 | 11.4 | 1 | 0.005451355595141649 |
7.3 | 0.26 | 0.36 | 5.2 | 0.04 | 31.0 | 141.0 | 0.9931 | 3.16 | 0.59 | 11.0 | 0 | 0.01210264302790165 |
6.2 | 0.43 | 0.22 | 1.8 | 0.078 | 21.0 | 56.0 | 0.99633 | 3.52 | 0.6 | 9.5 | 1 | 3.9473347715102136E-4 |
7.3 | 0.19 | 0.24 | 6.3 | 0.054 | 34.0 | 231.0 | 0.9964 | 3.36 | 0.54 | 10.0 | 0 | 0.0028029014356434345 |
6.7 | 0.46 | 0.24 | 1.7 | 0.077 | 18.0 | 34.0 | 0.9948 | 3.39 | 0.6 | 10.6 | 1 | 0.0010419178288429976 |
6.0 | 0.28 | 0.29 | 19.3 | 0.051 | 36.0 | 174.0 | 0.99911 | 3.14 | 0.5 | 9.0 | 0 | 6.508899969048798E-4 |
5.5 | 0.16 | 0.31 | 1.2 | 0.026 | 31.0 | 68.0 | 0.9898 | 3.33 | 0.44 | 11.65 | 0 | 0.04558026045560837 |
6.0 | 0.34 | 0.32 | 3.8 | 0.044 | 13.0 | 116.0 | 0.99108 | 3.39 | 0.44 | 11.8 | 0 | 0.912011981010437 |
6.2 | 0.36 | 0.24 | 2.2 | 0.095 | 19.0 | 42.0 | 0.9946 | 3.57 | 0.57 | 11.7 | 1 | 0.003564683021977544 |
6.8 | 0.48 | 0.08 | 1.8 | 0.074 | 40.0 | 64.0 | 0.99529 | 3.12 | 0.49 | 9.6 | 1 | 3.652050800155848E-4 |
6.8 | 0.17 | 0.34 | 2.0 | 0.04 | 38.0 | 111.0 | 0.99 | 3.24 | 0.45 | 12.9 | 0 | 0.032764971256256104 |
6.5 | 0.22 | 0.19 | 4.5 | 0.096 | 16.0 | 115.0 | 0.9937 | 3.02 | 0.44 | 9.6 | 0 | 9.100752067752182E-4 |
8.1 | 0.2 | 0.3 | 1.3 | 0.036 | 7.0 | 49.0 | 0.99242 | 2.99 | 0.73 | 10.3 | 0 | 0.02282591164112091 |
6.5 | 0.22 | 0.19 | 1.1 | 0.064 | 36.0 | 191.0 | 0.99297 | 3.05 | 0.5 | 9.5 | 0 | 0.0013939893105998635 |
6.9 | 0.22 | 0.32 | 5.8 | 0.041 | 20.0 | 119.0 | 0.99296 | 3.17 | 0.55 | 11.2 | 0 | 0.02912171743810177 |
6.5 | 0.23 | 0.45 | 2.1 | 0.027 | 43.0 | 104.0 | 0.99054 | 3.02 | 0.52 | 11.3 | 0 | 0.048298608511686325 |
7.6 | 0.19 | 0.41 | 1.1 | 0.04 | 38.0 | 143.0 | 0.9907 | 2.92 | 0.42 | 11.4 | 0 | 0.004219695460051298 |
6.0 | 0.24 | 0.28 | 3.95 | 0.038 | 61.0 | 134.0 | 0.99146 | 3.3 | 0.54 | 11.3 | 0 | 0.9664438366889954 |
8.6 | 0.685 | 0.1 | 1.6 | 0.092 | 3.0 | 12.0 | 0.99745 | 3.31 | 0.65 | 9.55 | 1 | 0.0010611562756821513 |
7.1 | 0.755 | 0.15 | 1.8 | 0.107 | 20.0 | 84.0 | 0.99593 | 3.19 | 0.5 | 9.5 | 1 | 2.6103772688657045E-4 |
6.7 | 0.22 | 0.39 | 10.2 | 0.038 | 60.0 | 149.0 | 0.99725 | 3.17 | 0.54 | 10.0 | 0 | 0.9110959768295288 |
9.2 | 0.43 | 0.49 | 2.4 | 0.086 | 23.0 | 116.0 | 0.9976 | 3.23 | 0.64 | 9.5 | 1 | 0.002309913747012615 |
6.1 | 0.27 | 0.25 | 1.8 | 0.041 | 9.0 | 109.0 | 0.9929 | 3.08 | 0.54 | 9.0 | 0 | 0.0012119112070649862 |
6.4 | 0.31 | 0.28 | 2.5 | 0.039 | 34.0 | 137.0 | 0.98946 | 3.22 | 0.38 | 12.7 | 0 | 0.07940990477800369 |
6.0 | 0.33 | 0.38 | 9.7 | 0.04 | 29.0 | 124.0 | 0.9954 | 3.47 | 0.48 | 11.0 | 0 | 0.06274176388978958 |
7.4 | 0.26 | 0.31 | 7.6 | 0.047 | 52.0 | 177.0 | 0.9962 | 3.13 | 0.45 | 8.9 | 0 | 0.0017185311298817396 |
7.6 | 0.31 | 0.26 | 1.7 | 0.073 | 40.0 | 157.0 | 0.9938 | 3.1 | 0.46 | 9.8 | 0 | 0.0016460918122902513 |
6.0 | 0.28 | 0.22 | 12.15 | 0.048 | 42.0 | 163.0 | 0.9957 | 3.2 | 0.46 | 10.1 | 0 | 2.911787305492908E-4 |
7.4 | 0.26 | 0.29 | 3.7 | 0.048 | 14.0 | 73.0 | 0.9915 | 3.06 | 0.45 | 11.4 | 0 | 0.032887041568756104 |
8.0 | 0.81 | 0.25 | 3.4 | 0.076 | 34.0 | 85.0 | 0.99668 | 3.19 | 0.42 | 9.2 | 1 | 4.031520802527666E-4 |
6.3 | 0.48 | 0.04 | 1.1 | 0.046 | 30.0 | 99.0 | 0.9928 | 3.24 | 0.36 | 9.6 | 0 | 5.755506572313607E-4 |
6.8 | 0.27 | 0.28 | 13.3 | 0.076 | 50.0 | 163.0 | 0.9979 | 3.03 | 0.38 | 8.6 | 0 | 9.764382848516107E-4 |
6.9 | 0.2 | 0.5 | 10.0 | 0.036 | 78.0 | 167.0 | 0.9964 | 3.15 | 0.55 | 10.2 | 0 | 0.014034735970199108 |
7.4 | 0.34 | 0.28 | 12.1 | 0.049 | 31.0 | 149.0 | 0.99677 | 3.22 | 0.49 | 10.3 | 0 | 0.006987015251070261 |
8.7 | 0.63 | 0.28 | 2.7 | 0.096 | 17.0 | 69.0 | 0.99734 | 3.26 | 0.63 | 10.2 | 1 | 0.0014869890874251723 |
6.2 | 0.28 | 0.28 | 4.3 | 0.026 | 22.0 | 105.0 | 0.989 | 2.98 | 0.64 | 13.1 | 0 | 0.9536960124969482 |
6.7 | 0.24 | 0.33 | 12.3 | 0.046 | 31.0 | 145.0 | 0.9983 | 3.36 | 0.4 | 9.5 | 0 | 0.0023177654948085546 |
6.7 | 0.19 | 0.23 | 6.2 | 0.047 | 36.0 | 117.0 | 0.9945 | 3.34 | 0.43 | 9.6 | 0 | 0.0035026343539357185 |
6.2 | 0.26 | 0.32 | 15.3 | 0.031 | 64.0 | 185.0 | 0.99835 | 3.31 | 0.61 | 9.4 | 0 | 0.01539541780948639 |
8.3 | 0.6 | 0.25 | 2.2 | 0.118 | 9.0 | 38.0 | 0.99616 | 3.15 | 0.53 | 9.8 | 1 | 0.0017200869042426348 |
6.4 | 0.42 | 0.19 | 9.3 | 0.043 | 28.0 | 145.0 | 0.99433 | 3.23 | 0.53 | 10.98 | 0 | 0.004190320614725351 |
8.4 | 0.665 | 0.61 | 2.0 | 0.112 | 13.0 | 95.0 | 0.997 | 3.16 | 0.54 | 9.1 | 1 | 3.235909971408546E-4 |
6.7 | 0.34 | 0.3 | 15.6 | 0.054 | 51.0 | 196.0 | 0.9982 | 3.19 | 0.49 | 9.3 | 0 | 0.001941390917636454 |
7.4 | 0.23 | 0.25 | 1.4 | 0.049 | 43.0 | 141.0 | 0.9934 | 3.42 | 0.54 | 10.2 | 0 | 0.9497902393341064 |
6.2 | 0.3 | 0.32 | 1.2 | 0.052 | 32.0 | 185.0 | 0.99266 | 3.28 | 0.44 | 10.1 | 0 | 0.00117747753392905 |
6.9 | 0.33 | 0.26 | 5.0 | 0.027 | 46.0 | 143.0 | 0.9924 | 3.25 | 0.43 | 11.2 | 0 | 0.9773195385932922 |
7.4 | 0.37 | 0.26 | 9.6 | 0.05 | 33.0 | 134.0 | 0.99608 | 3.13 | 0.46 | 10.4 | 0 | 8.885665447451174E-4 |
9.3 | 0.36 | 0.39 | 1.5 | 0.08 | 41.0 | 55.0 | 0.99652 | 3.47 | 0.73 | 10.9 | 1 | 0.011752982623875141 |
6.9 | 0.54 | 0.04 | 3.0 | 0.077 | 7.0 | 27.0 | 0.9987 | 3.69 | 0.91 | 9.4 | 1 | 4.1800233884714544E-4 |
6.2 | 0.21 | 0.27 | 1.7 | 0.038 | 41.0 | 150.0 | 0.9933 | 3.49 | 0.71 | 10.5 | 0 | 0.9650802612304688 |
5.8 | 0.6 | 0.0 | 1.3 | 0.044 | 72.0 | 197.0 | 0.99202 | 3.56 | 0.43 | 10.9 | 0 | 0.0032613552175462246 |
6.5 | 0.51 | 0.25 | 1.7 | 0.048 | 39.0 | 177.0 | 0.99212 | 3.28 | 0.57 | 10.6 | 0 | 0.002468859078362584 |
6.8 | 0.36 | 0.32 | 1.6 | 0.039 | 10.0 | 124.0 | 0.9948 | 3.3 | 0.67 | 9.6 | 0 | 0.0014277779264375567 |
13.2 | 0.46 | 0.52 | 2.2 | 0.071 | 12.0 | 35.0 | 1.0006 | 3.1 | 0.56 | 9.0 | 1 | 0.0030451035127043724 |
7.7 | 0.18 | 0.3 | 1.2 | 0.046 | 49.0 | 199.0 | 0.99413 | 3.03 | 0.38 | 9.3 | 0 | 0.022824544459581375 |
6.3 | 0.695 | 0.55 | 12.9 | 0.056 | 58.0 | 252.0 | 0.99806 | 3.29 | 0.49 | 8.7 | 0 | 6.824544398114085E-4 |
7.9 | 0.35 | 0.24 | 15.6 | 0.072 | 44.0 | 229.0 | 0.99785 | 3.03 | 0.59 | 10.5 | 0 | 0.001311535364948213 |
6.8 | 0.12 | 0.3 | 12.9 | 0.049 | 32.0 | 88.0 | 0.99654 | 3.2 | 0.35 | 9.9 | 0 | 0.03290209174156189 |
7.3 | 0.19 | 0.49 | 15.55 | 0.058 | 50.0 | 134.0 | 0.9998 | 3.42 | 0.36 | 9.1 | 0 | 0.9447118043899536 |
6.7 | 0.47 | 0.34 | 8.9 | 0.043 | 31.0 | 172.0 | 0.9964 | 3.22 | 0.6 | 9.2 | 0 | 0.0020339814946055412 |
8.3 | 0.3 | 0.49 | 3.8 | 0.09 | 11.0 | 24.0 | 0.99498 | 3.27 | 0.64 | 12.1 | 1 | 0.9744718074798584 |
7.1 | 0.16 | 0.25 | 1.3 | 0.034 | 28.0 | 123.0 | 0.9915 | 3.27 | 0.55 | 11.4 | 0 | 0.041309211403131485 |
7.0 | 0.42 | 0.19 | 2.3 | 0.071 | 18.0 | 36.0 | 0.99476 | 3.39 | 0.56 | 10.9 | 1 | 0.0027783899568021297 |
8.4 | 0.35 | 0.71 | 12.2 | 0.046 | 22.0 | 160.0 | 0.9982 | 2.98 | 0.65 | 9.4 | 0 | 4.85344062326476E-4 |
6.4 | 0.24 | 0.49 | 5.8 | 0.053 | 25.0 | 120.0 | 0.9942 | 3.01 | 0.98 | 10.5 | 0 | 0.006541768554598093 |
8.0 | 0.28 | 0.44 | 1.8 | 0.081 | 28.0 | 68.0 | 0.99501 | 3.36 | 0.66 | 11.2 | 1 | 0.002928321249783039 |
5.0 | 0.35 | 0.25 | 7.8 | 0.031 | 24.0 | 116.0 | 0.99241 | 3.39 | 0.4 | 11.3 | 0 | 0.01345854066312313 |
5.8 | 0.36 | 0.26 | 3.3 | 0.038 | 40.0 | 153.0 | 0.9911 | 3.34 | 0.55 | 11.3 | 0 | 0.04131815582513809 |
11.9 | 0.695 | 0.53 | 3.4 | 0.128 | 7.0 | 21.0 | 0.9992 | 3.17 | 0.84 | 12.2 | 1 | 0.9229516386985779 |
7.3 | 0.33 | 0.22 | 1.4 | 0.041 | 40.0 | 177.0 | 0.99287 | 3.14 | 0.48 | 9.9 | 0 | 5.245940992608666E-4 |
6.5 | 0.26 | 0.28 | 12.5 | 0.046 | 80.0 | 225.0 | 0.99685 | 3.18 | 0.41 | 10.0 | 0 | 6.041912129148841E-4 |
7.0 | 0.31 | 0.29 | 1.4 | 0.037 | 33.0 | 128.0 | 0.9896 | 3.12 | 0.36 | 12.2 | 0 | 0.8884886503219604 |
7.0 | 0.12 | 0.29 | 10.3 | 0.039 | 41.0 | 98.0 | 0.99564 | 3.19 | 0.38 | 9.8 | 0 | 0.9679784774780273 |
6.8 | 0.36 | 0.32 | 1.8 | 0.067 | 4.0 | 8.0 | 0.9928 | 3.36 | 0.55 | 12.8 | 1 | 0.9472044110298157 |
7.0 | 0.56 | 0.17 | 1.7 | 0.065 | 15.0 | 24.0 | 0.99514 | 3.44 | 0.68 | 10.55 | 1 | 0.9256985187530518 |
10.9 | 0.39 | 0.47 | 1.8 | 0.118 | 6.0 | 14.0 | 0.9982 | 3.3 | 0.75 | 9.8 | 1 | 0.00694586057215929 |
7.0 | 0.31 | 0.35 | 1.6 | 0.063 | 13.0 | 119.0 | 0.99184 | 3.22 | 0.5 | 10.7 | 0 | 0.006520157679915428 |
6.4 | 0.31 | 0.39 | 7.5 | 0.04 | 57.0 | 213.0 | 0.99475 | 3.32 | 0.43 | 10.0 | 0 | 0.004869484808295965 |
6.0 | 0.13 | 0.36 | 1.6 | 0.052 | 23.0 | 72.0 | 0.98974 | 3.1 | 0.5 | 11.5 | 0 | 0.943878173828125 |
7.3 | 0.205 | 0.31 | 1.7 | 0.06 | 34.0 | 110.0 | 0.9963 | 3.72 | 0.69 | 10.5 | 0 | 0.010451026260852814 |
10.6 | 0.34 | 0.49 | 3.2 | 0.078 | 20.0 | 78.0 | 0.9992 | 3.19 | 0.7 | 10.0 | 1 | 0.0026985453441739082 |
6.8 | 0.64 | 0.1 | 2.1 | 0.085 | 18.0 | 101.0 | 0.9956 | 3.34 | 0.52 | 10.2 | 1 | 4.1112827602773905E-4 |
8.6 | 0.37 | 0.7 | 12.15 | 0.039 | 21.0 | 158.0 | 0.9983 | 3.0 | 0.73 | 9.3 | 0 | 0.0010125628905370831 |
7.1 | 0.62 | 0.06 | 1.3 | 0.07 | 5.0 | 12.0 | 0.9942 | 3.17 | 0.48 | 9.8 | 1 | 0.0018506946507841349 |
6.9 | 0.29 | 0.3 | 8.2 | 0.026 | 35.0 | 112.0 | 0.99144 | 3.0 | 0.37 | 12.3 | 0 | 0.04193001613020897 |
7.4 | 0.22 | 0.28 | 9.0 | 0.046 | 22.0 | 121.0 | 0.99468 | 3.1 | 0.55 | 10.8 | 0 | 0.025308553129434586 |
6.9 | 0.37 | 0.23 | 9.5 | 0.057 | 54.0 | 166.0 | 0.99568 | 3.23 | 0.42 | 10.0 | 0 | 6.254533655010164E-4 |
6.0 | 0.26 | 0.18 | 7.0 | 0.055 | 50.0 | 194.0 | 0.99591 | 3.21 | 0.43 | 9.0 | 0 | 3.1700218096375465E-4 |
5.2 | 0.34 | 0.0 | 1.8 | 0.05 | 27.0 | 63.0 | 0.9916 | 3.68 | 0.79 | 14.0 | 1 | 0.039752718061208725 |
7.2 | 0.21 | 0.29 | 3.1 | 0.044 | 39.0 | 122.0 | 0.99143 | 3.0 | 0.6 | 11.3 | 0 | 0.07446765154600143 |
5.6 | 0.225 | 0.24 | 9.8 | 0.054 | 59.0 | 140.0 | 0.99545 | 3.17 | 0.39 | 10.2 | 0 | 0.0011124128941446543 |
7.4 | 0.49 | 0.27 | 2.1 | 0.071 | 14.0 | 25.0 | 0.99388 | 3.35 | 0.63 | 12.0 | 1 | 0.011491373181343079 |
7.6 | 0.17 | 0.46 | 0.9 | 0.036 | 63.0 | 147.0 | 0.99126 | 3.02 | 0.41 | 10.7 | 0 | 0.022345615550875664 |
6.7 | 0.66 | 0.0 | 13.0 | 0.033 | 32.0 | 75.0 | 0.99551 | 3.15 | 0.5 | 10.7 | 0 | 0.0010189638705924153 |
7.4 | 0.36 | 0.32 | 1.9 | 0.036 | 27.0 | 119.0 | 0.99196 | 3.15 | 0.49 | 11.2 | 0 | 0.04197188839316368 |
7.9 | 0.69 | 0.21 | 2.1 | 0.08 | 33.0 | 141.0 | 0.9962 | 3.25 | 0.51 | 9.9 | 1 | 1.4118352555669844E-4 |
7.4 | 0.18 | 0.27 | 1.3 | 0.048 | 26.0 | 105.0 | 0.994 | 3.52 | 0.66 | 10.6 | 0 | 0.04988418146967888 |
7.3 | 0.22 | 0.31 | 2.3 | 0.018 | 45.0 | 80.0 | 0.98936 | 3.06 | 0.34 | 12.9 | 0 | 0.9724825024604797 |
7.5 | 0.18 | 0.45 | 4.6 | 0.041 | 67.0 | 158.0 | 0.9927 | 3.01 | 0.38 | 10.6 | 0 | 0.033634208142757416 |
7.3 | 0.23 | 0.37 | 1.9 | 0.041 | 51.0 | 165.0 | 0.9908 | 3.26 | 0.4 | 12.2 | 0 | 0.9677072167396545 |
7.1 | 0.12 | 0.3 | 3.1 | 0.018 | 15.0 | 37.0 | 0.99004 | 3.02 | 0.52 | 11.9 | 0 | 0.9629055857658386 |
7.1 | 0.34 | 0.86 | 1.4 | 0.174 | 36.0 | 99.0 | 0.99288 | 2.92 | 0.5 | 9.3 | 0 | 0.0012647579424083233 |
5.8 | 0.24 | 0.28 | 1.4 | 0.038 | 40.0 | 76.0 | 0.98711 | 3.1 | 0.29 | 13.9 | 0 | 0.9646397829055786 |
7.3 | 0.34 | 0.33 | 2.5 | 0.064 | 21.0 | 37.0 | 0.9952 | 3.35 | 0.77 | 12.1 | 1 | 0.9876672029495239 |
9.8 | 0.36 | 0.45 | 1.6 | 0.042 | 11.0 | 124.0 | 0.9944 | 2.93 | 0.46 | 10.8 | 0 | 0.010374616831541061 |
6.4 | 0.17 | 0.32 | 2.4 | 0.048 | 41.0 | 200.0 | 0.9938 | 3.5 | 0.5 | 9.7 | 0 | 0.01836015284061432 |
11.9 | 0.39 | 0.69 | 2.8 | 0.095 | 17.0 | 35.0 | 0.9994 | 3.1 | 0.61 | 10.8 | 1 | 0.016166161745786667 |
7.0 | 0.13 | 0.37 | 12.85 | 0.042 | 36.0 | 105.0 | 0.99581 | 3.05 | 0.55 | 10.7 | 0 | 0.029500233009457588 |
5.8 | 0.35 | 0.29 | 3.2 | 0.034 | 41.0 | 151.0 | 0.9912 | 3.35 | 0.58 | 11.6333333333333 | 0 | 0.9659104943275452 |
6.5 | 0.2 | 0.31 | 2.1 | 0.033 | 32.0 | 95.0 | 0.989435 | 2.96 | 0.61 | 12.0 | 0 | 0.027638055384159088 |
5.5 | 0.32 | 0.45 | 4.9 | 0.028 | 25.0 | 191.0 | 0.9922 | 3.51 | 0.49 | 11.5 | 0 | 0.9377204179763794 |
7.5 | 0.77 | 0.2 | 8.1 | 0.098 | 30.0 | 92.0 | 0.99892 | 3.2 | 0.58 | 9.2 | 1 | 3.815419040620327E-4 |
6.9 | 0.17 | 0.25 | 1.6 | 0.047 | 34.0 | 132.0 | 0.9914 | 3.16 | 0.48 | 11.4 | 0 | 0.03217584639787674 |
6.6 | 0.25 | 0.35 | 2.9 | 0.034 | 38.0 | 121.0 | 0.99008 | 3.19 | 0.4 | 12.8 | 0 | 0.10999802500009537 |
6.3 | 0.24 | 0.37 | 1.8 | 0.031 | 6.0 | 61.0 | 0.9897 | 3.3 | 0.34 | 12.2 | 0 | 0.015069236978888512 |
8.3 | 0.6 | 0.25 | 2.2 | 0.118 | 9.0 | 38.0 | 0.99616 | 3.15 | 0.53 | 9.8 | 1 | 0.0017200869042426348 |
6.6 | 0.39 | 0.39 | 11.9 | 0.057 | 51.0 | 221.0 | 0.99851 | 3.26 | 0.51 | 8.9 | 0 | 0.0038905905093997717 |
7.4 | 0.16 | 0.27 | 15.5 | 0.05 | 25.0 | 135.0 | 0.9984 | 2.9 | 0.43 | 8.7 | 0 | 0.9851395487785339 |
8.9 | 0.4 | 0.32 | 5.6 | 0.087 | 10.0 | 47.0 | 0.9991 | 3.38 | 0.77 | 10.5 | 1 | 0.9230940341949463 |
6.2 | 0.12 | 0.26 | 5.7 | 0.044 | 56.0 | 158.0 | 0.9951 | 3.52 | 0.37 | 10.5 | 0 | 0.011266084387898445 |
6.7 | 0.41 | 0.27 | 2.6 | 0.033 | 25.0 | 85.0 | 0.99086 | 3.05 | 0.34 | 11.7 | 0 | 0.017297135666012764 |
6.5 | 0.41 | 0.22 | 4.8 | 0.052 | 49.0 | 142.0 | 0.9946 | 3.14 | 0.62 | 9.2 | 0 | 5.140446592122316E-4 |
7.1 | 0.6 | 0.0 | 1.8 | 0.074 | 16.0 | 34.0 | 0.9972 | 3.47 | 0.7 | 9.9 | 1 | 0.01600511372089386 |
7.3 | 0.3 | 0.25 | 2.5 | 0.045 | 32.0 | 122.0 | 0.99329 | 3.18 | 0.54 | 10.3 | 0 | 0.005259253084659576 |
6.8 | 0.32 | 0.3 | 3.3 | 0.029 | 15.0 | 80.0 | 0.99061 | 3.33 | 0.63 | 12.6 | 0 | 0.944897472858429 |
6.7 | 0.28 | 0.28 | 2.4 | 0.012 | 36.0 | 100.0 | 0.99064 | 3.26 | 0.39 | 11.7 | 0 | 0.9872050285339355 |
6.2 | 0.3 | 0.17 | 2.8 | 0.04 | 24.0 | 125.0 | 0.9939 | 3.01 | 0.46 | 9.0 | 0 | 0.0010926135582849383 |
6.7 | 0.18 | 0.28 | 10.2 | 0.039 | 29.0 | 115.0 | 0.99469 | 3.11 | 0.45 | 10.9 | 0 | 0.9911683797836304 |
6.8 | 0.15 | 0.41 | 12.9 | 0.044 | 79.5 | 182.0 | 0.99742 | 3.24 | 0.78 | 10.2 | 0 | 0.00811257865279913 |
8.7 | 0.69 | 0.31 | 3.0 | 0.086 | 23.0 | 81.0 | 1.0002 | 3.48 | 0.74 | 11.6 | 1 | 0.015061787329614162 |
6.2 | 0.26 | 0.2 | 8.0 | 0.047 | 35.0 | 111.0 | 0.99445 | 3.11 | 0.42 | 10.4 | 0 | 6.406896281987429E-4 |
5.9 | 0.25 | 0.19 | 12.4 | 0.047 | 50.0 | 162.0 | 0.9973 | 3.35 | 0.38 | 9.5 | 0 | 0.0016871698899194598 |
8.3 | 0.54 | 0.24 | 3.4 | 0.076 | 16.0 | 112.0 | 0.9976 | 3.27 | 0.61 | 9.4 | 1 | 2.986558247357607E-4 |
8.2 | 0.31 | 0.4 | 2.2 | 0.058 | 6.0 | 10.0 | 0.99536 | 3.31 | 0.68 | 11.2 | 1 | 0.9167342782020569 |
7.0 | 0.24 | 0.24 | 1.8 | 0.047 | 29.0 | 91.0 | 0.99251 | 3.3 | 0.43 | 9.9 | 0 | 0.01589989848434925 |
7.6 | 0.345 | 0.26 | 1.9 | 0.043 | 15.0 | 134.0 | 0.9936 | 3.08 | 0.38 | 9.5 | 0 | 9.613612783141434E-4 |
8.2 | 0.74 | 0.09 | 2.0 | 0.067 | 5.0 | 10.0 | 0.99418 | 3.28 | 0.57 | 11.8 | 1 | 0.006446065381169319 |
6.9 | 0.23 | 0.34 | 4.0 | 0.047 | 24.0 | 128.0 | 0.9944 | 3.2 | 0.52 | 9.7 | 0 | 0.002935371594503522 |
7.0 | 0.6 | 0.12 | 2.2 | 0.083 | 13.0 | 28.0 | 0.9966 | 3.52 | 0.62 | 10.2 | 1 | 0.8038749694824219 |
11.6 | 0.44 | 0.64 | 2.1 | 0.059 | 5.0 | 15.0 | 0.998 | 3.21 | 0.67 | 10.2 | 1 | 0.018257485702633858 |
7.1 | 0.17 | 0.31 | 1.6 | 0.037 | 15.0 | 103.0 | 0.991 | 3.14 | 0.5 | 12.0 | 0 | 0.02079176716506481 |
7.4 | 0.19 | 0.3 | 12.8 | 0.053 | 48.5 | 229.0 | 0.9986 | 3.14 | 0.49 | 9.1 | 0 | 0.9788848161697388 |
6.6 | 0.28 | 0.34 | 0.8 | 0.037 | 42.0 | 119.0 | 0.9888 | 3.03 | 0.37 | 12.5 | 0 | 0.013386632315814495 |
7.2 | 0.53 | 0.13 | 2.0 | 0.058 | 18.0 | 22.0 | 0.99573 | 3.21 | 0.68 | 9.9 | 1 | 0.03273240476846695 |
7.7 | 0.39 | 0.28 | 4.9 | 0.035 | 36.0 | 109.0 | 0.9918 | 3.19 | 0.58 | 12.2 | 0 | 0.9897956252098083 |
7.6 | 0.21 | 0.6 | 2.1 | 0.046 | 47.0 | 165.0 | 0.9936 | 3.05 | 0.54 | 10.1 | 0 | 0.9250134229660034 |
8.4 | 0.18 | 0.42 | 5.1 | 0.036 | 7.0 | 77.0 | 0.9939 | 3.16 | 0.52 | 11.7 | 0 | 0.00936033297330141 |
6.4 | 0.42 | 0.46 | 8.4 | 0.05 | 58.0 | 180.0 | 0.99495 | 3.18 | 0.46 | 9.7 | 0 | 9.7941467538476E-4 |
6.9 | 0.19 | 0.33 | 1.6 | 0.043 | 63.0 | 149.0 | 0.9925 | 3.44 | 0.52 | 10.8 | 0 | 0.025319170206785202 |
7.2 | 0.27 | 0.74 | 12.5 | 0.037 | 47.0 | 156.0 | 0.9981 | 3.04 | 0.44 | 8.7 | 0 | 0.0029928090516477823 |
7.7 | 0.39 | 0.12 | 1.7 | 0.097 | 19.0 | 27.0 | 0.99596 | 3.16 | 0.49 | 9.4 | 1 | 7.092177984304726E-4 |
7.0 | 0.29 | 0.26 | 1.6 | 0.044 | 12.0 | 87.0 | 0.9923 | 3.08 | 0.46 | 10.5 | 0 | 0.026244867593050003 |
8.3 | 0.23 | 0.43 | 3.2 | 0.035 | 14.0 | 101.0 | 0.9928 | 3.15 | 0.36 | 11.5 | 0 | 0.010557007044553757 |
7.4 | 0.34 | 0.3 | 14.9 | 0.037 | 70.0 | 169.0 | 0.99698 | 3.25 | 0.37 | 10.4 | 0 | 0.008540195412933826 |
8.2 | 0.73 | 0.21 | 1.7 | 0.074 | 5.0 | 13.0 | 0.9968 | 3.2 | 0.52 | 9.5 | 1 | 0.0012597866589203477 |
7.1 | 0.3 | 0.36 | 6.8 | 0.055 | 44.5 | 234.0 | 0.9972 | 3.49 | 0.64 | 10.2 | 0 | 0.0051595550030469894 |
7.1 | 0.59 | 0.0 | 2.2 | 0.078 | 26.0 | 44.0 | 0.99522 | 3.42 | 0.68 | 10.8 | 1 | 0.021802868694067 |
6.1 | 0.3 | 0.3 | 2.1 | 0.031 | 50.0 | 163.0 | 0.9895 | 3.39 | 0.43 | 12.7 | 0 | 0.9899473786354065 |
5.4 | 0.74 | 0.0 | 1.2 | 0.041 | 16.0 | 46.0 | 0.99258 | 4.01 | 0.59 | 12.5 | 1 | 0.02593737095594406 |
8.4 | 0.29 | 0.29 | 1.05 | 0.032 | 4.0 | 55.0 | 0.9908 | 2.91 | 0.32 | 11.4 | 0 | 0.009325387887656689 |
7.8 | 0.3 | 0.29 | 16.85 | 0.054 | 23.0 | 135.0 | 0.9998 | 3.16 | 0.38 | 9.0 | 0 | 0.004077394492924213 |
7.4 | 0.2 | 0.37 | 1.2 | 0.028 | 28.0 | 89.0 | 0.99132 | 3.14 | 0.61 | 11.8 | 0 | 0.052954766899347305 |
12.5 | 0.37 | 0.55 | 2.6 | 0.083 | 25.0 | 68.0 | 0.9995 | 3.15 | 0.82 | 10.4 | 1 | 0.009857555851340294 |
6.0 | 0.36 | 0.16 | 6.3 | 0.036 | 36.0 | 191.0 | 0.9942 | 3.17 | 0.62 | 9.8 | 0 | 0.00101988366805017 |
8.1 | 0.33 | 0.44 | 1.5 | 0.042 | 6.0 | 12.0 | 0.99542 | 3.35 | 0.61 | 10.7 | 1 | 0.030785350129008293 |
7.6 | 0.39 | 0.32 | 3.6 | 0.035 | 22.0 | 93.0 | 0.99144 | 3.08 | 0.6 | 12.5 | 0 | 0.9876891374588013 |
5.9 | 0.29 | 0.33 | 7.4 | 0.037 | 58.0 | 205.0 | 0.99495 | 3.26 | 0.41 | 9.6 | 0 | 0.0026708648074418306 |
6.4 | 0.38 | 0.24 | 7.2 | 0.047 | 41.0 | 151.0 | 0.99604 | 3.11 | 0.6 | 9.2 | 0 | 5.508633330464363E-4 |
6.0 | 0.16 | 0.36 | 1.6 | 0.042 | 13.0 | 61.0 | 0.99143 | 3.22 | 0.54 | 10.8 | 0 | 0.01489825826138258 |
6.9 | 0.22 | 0.49 | 7.0 | 0.063 | 50.0 | 168.0 | 0.9957 | 3.54 | 0.5 | 10.3 | 0 | 0.020039143040776253 |
7.1 | 0.36 | 0.3 | 1.6 | 0.08 | 35.0 | 70.0 | 0.99693 | 3.44 | 0.5 | 9.4 | 1 | 8.312936406582594E-4 |
7.3 | 0.13 | 0.27 | 4.6 | 0.08 | 34.0 | 172.0 | 0.9938 | 3.23 | 0.39 | 11.1 | 0 | 0.8825576305389404 |
6.6 | 0.32 | 0.33 | 2.5 | 0.052 | 40.0 | 219.5 | 0.99316 | 3.15 | 0.6 | 10.0 | 0 | 0.0014726795488968492 |
7.3 | 0.22 | 0.37 | 14.3 | 0.063 | 48.0 | 191.0 | 0.9978 | 2.89 | 0.38 | 9.0 | 0 | 0.03318009153008461 |
6.6 | 0.25 | 0.3 | 14.4 | 0.052 | 40.0 | 183.0 | 0.998 | 3.02 | 0.5 | 9.1 | 0 | 0.023252390325069427 |
8.4 | 0.35 | 0.56 | 13.8 | 0.048 | 55.0 | 190.0 | 0.9993 | 3.07 | 0.58 | 9.4 | 0 | 0.0025425157509744167 |
10.2 | 0.645 | 0.36 | 1.8 | 0.053 | 5.0 | 14.0 | 0.9982 | 3.17 | 0.42 | 10.0 | 1 | 0.0016229647444561124 |
6.5 | 0.22 | 0.34 | 12.0 | 0.053 | 55.0 | 177.0 | 0.9983 | 3.52 | 0.44 | 9.9 | 0 | 0.010934929363429546 |
6.5 | 0.25 | 0.27 | 17.4 | 0.064 | 29.0 | 140.0 | 0.99776 | 3.2 | 0.49 | 10.1 | 0 | 9.14691190700978E-4 |
7.6 | 0.18 | 0.28 | 7.1 | 0.041 | 29.0 | 110.0 | 0.99652 | 3.2 | 0.42 | 9.2 | 0 | 0.007518202066421509 |
5.9 | 0.26 | 0.25 | 12.5 | 0.034 | 38.0 | 152.0 | 0.9977 | 3.33 | 0.43 | 9.4 | 0 | 0.0026366899255663157 |
6.2 | 0.21 | 0.27 | 1.7 | 0.038 | 41.0 | 150.0 | 0.9933 | 3.49 | 0.71 | 10.5 | 0 | 0.9650802612304688 |
6.8 | 0.19 | 0.32 | 7.6 | 0.049 | 37.0 | 107.0 | 0.99332 | 3.12 | 0.44 | 10.7 | 0 | 0.8693210482597351 |
6.2 | 0.39 | 0.24 | 4.8 | 0.037 | 45.0 | 138.0 | 0.99174 | 3.23 | 0.43 | 11.2 | 0 | 0.9257086515426636 |
6.5 | 0.24 | 0.39 | 17.3 | 0.052 | 22.0 | 126.0 | 0.99888 | 3.11 | 0.47 | 9.2 | 0 | 5.760281346738338E-4 |
7.1 | 0.28 | 0.31 | 1.5 | 0.053 | 20.0 | 98.0 | 0.99069 | 3.15 | 0.5 | 11.4 | 0 | 0.01739666610956192 |
6.6 | 0.19 | 0.28 | 11.8 | 0.042 | 54.0 | 137.0 | 0.99492 | 3.18 | 0.37 | 10.8 | 0 | 0.0033521626610308886 |
7.0 | 0.28 | 0.33 | 14.6 | 0.043 | 47.0 | 168.0 | 0.9994 | 3.34 | 0.67 | 8.8 | 0 | 0.035414524376392365 |
7.3 | 0.27 | 0.37 | 9.7 | 0.042 | 36.0 | 130.0 | 0.9979 | 3.48 | 0.75 | 9.9 | 0 | 0.011170870624482632 |
6.0 | 0.2 | 0.38 | 1.3 | 0.034 | 37.0 | 104.0 | 0.98865 | 3.11 | 0.52 | 12.7 | 0 | 0.07085759937763214 |
6.2 | 0.34 | 0.25 | 12.1 | 0.059 | 33.0 | 171.0 | 0.99769 | 3.14 | 0.56 | 8.7 | 0 | 0.004047304391860962 |
7.4 | 0.29 | 0.28 | 10.2 | 0.032 | 43.0 | 138.0 | 0.9951 | 3.1 | 0.47 | 10.6 | 0 | 0.026839742437005043 |
6.1 | 0.46 | 0.32 | 6.2 | 0.053 | 10.0 | 94.0 | 0.99537 | 3.35 | 0.47 | 10.1 | 0 | 0.0016266652382910252 |
8.2 | 0.23 | 0.29 | 1.8 | 0.047 | 47.0 | 187.0 | 0.9933 | 3.13 | 0.5 | 10.2 | 0 | 0.017404843121767044 |
8.2 | 0.28 | 0.42 | 1.8 | 0.031 | 30.0 | 93.0 | 0.9917 | 3.09 | 0.39 | 11.4 | 0 | 0.035138558596372604 |
7.6 | 0.28 | 0.39 | 1.2 | 0.038 | 21.0 | 115.0 | 0.994 | 3.16 | 0.67 | 10.0 | 0 | 0.0014765552477911115 |
7.0 | 0.34 | 0.3 | 1.8 | 0.045 | 44.0 | 142.0 | 0.9914 | 2.99 | 0.45 | 10.8 | 0 | 0.0028043065685778856 |
7.6 | 0.5 | 0.29 | 2.3 | 0.086 | 5.0 | 14.0 | 0.99502 | 3.32 | 0.62 | 11.5 | 1 | 0.017077432945370674 |
5.9 | 0.29 | 0.16 | 7.9 | 0.044 | 48.0 | 197.0 | 0.99512 | 3.21 | 0.36 | 9.4 | 0 | 4.3206868576817214E-4 |
8.7 | 0.765 | 0.22 | 2.3 | 0.064 | 9.0 | 42.0 | 0.9963 | 3.1 | 0.55 | 9.4 | 1 | 2.875835925806314E-4 |
7.0 | 0.24 | 0.26 | 1.7 | 0.041 | 31.0 | 110.0 | 0.99142 | 3.2 | 0.53 | 11.0 | 0 | 0.02426009811460972 |
6.3 | 0.68 | 0.01 | 3.7 | 0.103 | 32.0 | 54.0 | 0.99586 | 3.51 | 0.66 | 11.3 | 1 | 0.006766395177692175 |
5.8 | 0.26 | 0.29 | 1.0 | 0.042 | 35.0 | 101.0 | 0.99044 | 3.36 | 0.48 | 11.4 | 0 | 0.9091703295707703 |
7.8 | 0.15 | 0.34 | 1.1 | 0.035 | 31.0 | 93.0 | 0.99096 | 3.07 | 0.72 | 11.3 | 0 | 0.89020836353302 |
7.3 | 0.18 | 0.31 | 17.3 | 0.055 | 32.0 | 197.0 | 1.0002 | 3.13 | 0.46 | 9.0 | 0 | 0.20837746560573578 |
7.6 | 0.35 | 0.46 | 14.7 | 0.047 | 33.0 | 151.0 | 0.99709 | 3.03 | 0.53 | 10.3 | 0 | 0.006563443690538406 |
15.5 | 0.645 | 0.49 | 4.2 | 0.095 | 10.0 | 23.0 | 1.00315 | 2.92 | 0.74 | 11.1 | 1 | 0.028600074350833893 |
6.2 | 0.17 | 0.3 | 1.1 | 0.037 | 14.0 | 79.0 | 0.993 | 3.5 | 0.54 | 10.3 | 0 | 0.052771855145692825 |
7.1 | 0.35 | 0.27 | 3.1 | 0.034 | 28.0 | 134.0 | 0.9897 | 3.26 | 0.38 | 13.1 | 0 | 0.9499262571334839 |
8.6 | 0.37 | 0.65 | 6.4 | 0.08 | 3.0 | 8.0 | 0.99817 | 3.27 | 0.58 | 11.0 | 1 | 0.011003587394952774 |
8.5 | 0.17 | 0.31 | 1.0 | 0.024 | 13.0 | 91.0 | 0.993 | 2.79 | 0.37 | 10.1 | 0 | 0.00858304277062416 |
5.6 | 0.41 | 0.22 | 7.1 | 0.05 | 44.0 | 154.0 | 0.9931 | 3.3 | 0.4 | 10.5 | 0 | 0.0031493024434894323 |
6.7 | 0.24 | 0.41 | 8.7 | 0.036 | 29.0 | 148.0 | 0.9952 | 3.22 | 0.62 | 9.9 | 0 | 0.011120235547423363 |
5.8 | 0.28 | 0.3 | 3.9 | 0.026 | 36.0 | 105.0 | 0.98963 | 3.26 | 0.58 | 12.75 | 0 | 0.05128045752644539 |
6.1 | 0.22 | 0.49 | 1.5 | 0.051 | 18.0 | 87.0 | 0.9928 | 3.3 | 0.46 | 9.6 | 0 | 0.0013162964023649693 |
5.3 | 0.76 | 0.03 | 2.7 | 0.043 | 27.0 | 93.0 | 0.9932 | 3.34 | 0.38 | 9.2 | 0 | 8.458032971248031E-4 |
6.1 | 0.64 | 0.02 | 2.4 | 0.069 | 26.0 | 46.0 | 0.99358 | 3.47 | 0.45 | 11.0 | 1 | 0.0011685565114021301 |
8.5 | 0.25 | 0.27 | 4.7 | 0.031 | 31.0 | 92.0 | 0.9922 | 3.01 | 0.33 | 12.0 | 0 | 0.016884110867977142 |
6.0 | 0.54 | 0.06 | 1.8 | 0.05 | 38.0 | 89.0 | 0.99236 | 3.3 | 0.5 | 10.55 | 1 | 0.0013655571965500712 |
7.9 | 0.21 | 0.4 | 1.2 | 0.039 | 38.0 | 107.0 | 0.992 | 3.21 | 0.54 | 10.8 | 0 | 0.015082137659192085 |
5.6 | 0.26 | 0.26 | 5.7 | 0.031 | 12.0 | 80.0 | 0.9923 | 3.25 | 0.38 | 10.8 | 0 | 0.04800743982195854 |
7.0 | 0.69 | 0.07 | 2.5 | 0.091 | 15.0 | 21.0 | 0.99572 | 3.38 | 0.6 | 11.3 | 1 | 0.007022011559456587 |
5.0 | 1.02 | 0.04 | 1.4 | 0.045 | 41.0 | 85.0 | 0.9938 | 3.75 | 0.48 | 10.5 | 1 | 0.010948577895760536 |
7.4 | 0.6 | 0.26 | 2.1 | 0.083 | 17.0 | 91.0 | 0.99616 | 3.29 | 0.56 | 9.8 | 1 | 4.6006462071090937E-4 |
8.0 | 0.38 | 0.44 | 1.9 | 0.098 | 6.0 | 15.0 | 0.9956 | 3.3 | 0.64 | 11.4 | 1 | 0.011728810146450996 |
6.6 | 0.25 | 0.31 | 12.4 | 0.059 | 52.0 | 181.0 | 0.9984 | 3.51 | 0.47 | 9.8 | 0 | 0.00705223623663187 |
8.0 | 0.3 | 0.36 | 11.0 | 0.034 | 8.0 | 70.0 | 0.99354 | 3.05 | 0.41 | 12.2 | 0 | 0.0710487887263298 |
6.1 | 0.21 | 0.3 | 6.3 | 0.039 | 47.0 | 136.0 | 0.99068 | 3.27 | 0.31 | 12.7 | 0 | 0.06314196437597275 |
6.5 | 0.18 | 0.31 | 1.7 | 0.044 | 30.0 | 127.0 | 0.9928 | 3.49 | 0.5 | 10.2 | 0 | 0.9202941060066223 |
6.2 | 0.33 | 0.19 | 5.6 | 0.042 | 22.0 | 143.0 | 0.99425 | 3.15 | 0.63 | 9.9 | 0 | 0.0013126832200214267 |
5.5 | 0.485 | 0.0 | 1.5 | 0.065 | 8.0 | 103.0 | 0.994 | 3.63 | 0.4 | 9.7 | 0 | 0.0017414502799510956 |
8.5 | 0.28 | 0.34 | 13.8 | 0.041 | 32.0 | 161.0 | 0.9981 | 3.13 | 0.4 | 9.9 | 0 | 0.0011877368669956923 |
6.0 | 0.17 | 0.29 | 5.0 | 0.028 | 25.0 | 108.0 | 0.99076 | 3.14 | 0.34 | 12.3 | 0 | 0.05751296877861023 |
5.6 | 0.695 | 0.06 | 6.8 | 0.042 | 9.0 | 84.0 | 0.99432 | 3.44 | 0.44 | 10.2 | 0 | 0.00686450581997633 |
7.6 | 0.3 | 0.4 | 2.2 | 0.054 | 29.0 | 175.0 | 0.99445 | 3.19 | 0.53 | 9.8 | 0 | 0.00250054057687521 |
7.3 | 0.51 | 0.18 | 2.1 | 0.07 | 12.0 | 28.0 | 0.99768 | 3.52 | 0.73 | 9.5 | 1 | 0.020017987117171288 |
6.7 | 0.41 | 0.27 | 2.6 | 0.033 | 25.0 | 85.0 | 0.99086 | 3.05 | 0.34 | 11.7 | 0 | 0.017297135666012764 |
6.7 | 0.28 | 0.34 | 8.9 | 0.048 | 32.0 | 111.0 | 0.99455 | 3.25 | 0.54 | 11.0 | 0 | 0.9808989763259888 |
Model serving#
To productionize the model for low latency predictions, use MLflow Model Serving (AWS|Azure|GCP) to deploy the model to an endpoint.
The following code illustrates how to issue requests using a REST API to get predictions from the deployed model.
You need a Databricks token to issue requests to your model endpoint. You can generate a token from the User Settings page (click Settings in the left sidebar). Copy the token into the next cell.
import os
os.environ["DATABRICKS_TOKEN"] = "<YOUR_TOKEN>"
Click Models in the left sidebar and navigate to the registered wine model. Click the serving tab, and then click Enable Serving.
Then, under Call The Model, click the Python button to display a Python code snippet to issue requests. Copy the code into this notebook. It should look similar to the code in the next cell.
You can use the token to make these requests from outside Databricks notebooks as well.
# Replace with code snippet from the model serving page
import os
import requests
import pandas as pd
def score_model(dataset: pd.DataFrame):
url = 'https://<DATABRICKS_URL>/model/wine_quality/Production/invocations'
headers = {'Authorization': f'Bearer {os.environ.get("DATABRICKS_TOKEN")}'}
data_json = dataset.to_dict(orient='split')
response = requests.request(method='POST', headers=headers, url=url, json=data_json)
if response.status_code != 200:
raise Exception(f'Request failed with status {response.status_code}, {response.text}')
return response.json()
The model predictions from the endpoint should agree with the results from locally evaluating the model.
# Model serving is designed for low-latency predictions on smaller batches of data
num_predictions = 5
served_predictions = score_model(X_test[:num_predictions])
model_evaluations = model.predict(X_test[:num_predictions])
# Compare the results from the deployed model and the trained model
pd.DataFrame({
"Model Prediction": model_evaluations,
"Served Model Prediction": served_predictions,
})